Question
Your task for this assignment is to select any one of your programming assignments and to rewrite it as generic software. This means that the
Your task for this assignment is to select any one of your programming assignments and to rewrite it as generic software. This means that the class for the data structure -- array, linked list, stack , queue, etc. -- should be rewritten as a generic class.
You can implement the software in your project to use the same data object as the original project, but if you write the data structure software itself as proper generic software, it should work with any class of object as the data object. The exception to this might be a search method, which might make reference to a specific property of the data object. If that's the case, you can omit the search method from this project.
I suggest you pick a simple assignment. Our purpose here is just to make sure that you understand and can write generic software.
PREVIOUS WORK DONE:
LIST CLASS:
import java.util.Iterator; //This is the class of the list itself public class List implements Iterable { // head holds the start of the list private ListNode head; // constructor public List() { // set read to null this.head = null; } // insert at the end of the list public void insertRear(T data) { ListNode aNode = new ListNode(data); if (this.head == null) { this.head = aNode; } else { ListNode rearNode = this.head; while (rearNode.getNext() != null) { rearNode = rearNode.getNext(); } rearNode.setNext(aNode); } } // returns an iterator to iterate the list @Override public Iterator iterator() { return new Iterator() { //hold the current list node being iterated. ListNode aNode = List.this.head; //returns true if current list node is the last element of the list, returns false otherwise. @Override public boolean hasNext() { return aNode != null; } @Override public T next() { if (this.hasNext()) { T data = aNode.getData(); aNode = aNode.getNext(); return data; } else { return null; } } }; } }
LIST NODE CLASS:
// class for a list node public class ListNode { // data holds the node's private T data; private ListNode next; // constructor public ListNode(T data) { // set data from parameter // set next to null this.data = data; this.next = null; } // getter for data public T getData() { // return data return data; } // setter for data public void setData(T data) { // set data from parameter this.data = data; } // getter for next public ListNode getNext() { // return next return next; } // setter for next public void setNext(ListNode next) { // set next from parameter this.next = next; } } OCCUPATION CLASS:
//This class contains the properties of an occupation, as well as manipulating said properties. public class Occupation { private String COS; private String title; private String employment; private String salary; public Occupation() { //default constructor, sets default values to the properties COS = ""; title = ""; employment = ""; salary = ""; } public Occupation(String COS, String title, String employment, String salary) { //contains parameters and set values to the properties based on parameters this.COS = COS; this.title = title; this.employment = employment; this.salary = salary; } /* The code below are my setters and getters for this project. They are for properties I declared. COS, title, employment, salary. I also made a toString() method to display the information. */ public String getCOS() { return COS; } public void setCOS(String cOS) { COS = cOS; } public String getTitle() { return this.title; } public void setTitle(String title) { this.title = title; } public String getEmployment() { return employment; } public void setEmployment(String employment) { this.employment = employment; } public String getSalary() { return salary; } public void setSalary(String salary) { this.salary = salary; } public String toString() { return "[" + COS + " : " + title + "] - " + employment + " - " + salary; } }
OCCUPATIONLIST CLASS:
import java.io.File; import java.util.Scanner; import java.util.Iterator; // Changed this class to list instead of array. Works the same way as array did but with list instead. public class OccupationList { private List occ; public OccupationList(String fileName) { occ = new List(); loadDataFile(fileName); } // Below is practically what allows me to load any text file and store it in the array. The text file comes from a user input from the executable class. public void loadDataFile(String fileName) { try { File currentDir = new File("."); File parentDir = currentDir.getParentFile(); File file = new File(parentDir,fileName); Scanner reader = new Scanner(file); while(reader.hasNextLine()) { String COS = reader.nextLine(); String title = reader.nextLine(); String employment = reader.nextLine(); String salary = reader.nextLine(); occ.insertRear(new Occupation(COS, title, employment, salary)); } reader.close(); } catch(Exception e) { System.out.println("File specified not found"); System.exit(0); } } public void displayOccupation() { // This method displays all occupations and corresponding information. Iterator anIterator = occ.iterator(); while (anIterator.hasNext()) { System.out.println(anIterator.next()); } } public void searchByCOS(String COS) { // This method searches and displays an occupation information based on the given COS. The COS will also be in the executable class boolean isFound = false; Occupation anOccupation = null; Iterator anIterator = occ.iterator(); while (anIterator.hasNext()) { anOccupation = anIterator.next(); if (COS.equals(anOccupation.getCOS())) { isFound = true; break; } } if (isFound) { System.out.println(anOccupation); } else { System.out.println("No COS information found."); } } public void searchByTitle(String title) { // This method is like searchbyCOS except you search for the title. boolean isFound = false; Occupation anOccupation = null; Iterator anIterator = occ.iterator(); while (anIterator.hasNext()) { anOccupation = anIterator.next(); if (title.equals(anOccupation.getTitle())) { isFound = true; break; } } if (isFound) { System.out.println(anOccupation); } else { System.out.println("No title information found."); } } }
OCCUPATIONMAIN CLASS: import java.util.Scanner; //This class is the executable class. Contains the main() method. public class OccupationMain { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter file name: "); //Asks the user for the name of the text file. In this case, we will be using "occupations.txt" String fileName = sc.nextLine(); OccupationList ol = new OccupationList(fileName); //Create instance of OccupationList object, using the file name as its parameter. while(true){ System.out.println("MENU"); //This is what displays after. It is a menu with user input. Infinite looping while true System.out.println("1 - Print all occupations"); System.out.println("2 - Print the total number of people employed across all occupations"); System.out.println("3 - Print the average of all the average salaries"); System.out.println("0 - Exit"); System.out.print("Enter choice: "); int choice = Integer.parseInt(sc.nextLine()); if(choice == 1) { //If user inputs "1", this calls the method displayOccupation() we made in OccupationList class. ol.displayOccupation(); System.out.println(); } else if(choice == 2) { //If user inputs "2", this calls the method searchByCOS() we made in OccupationList class. System.out.print("Enter COS to search: "); String COS = sc.nextLine(); ol.searchByCOS(COS); System.out.println(); } else if(choice == 3) { //If user inputs "3", this calls the method searchByTitle() we made in OccupationList class. System.out.print("Enter title to search: "); String title = sc.nextLine(); ol.searchByTitle(title); System.out.println(); } else if(choice == 0) { //If user inputs "0", this breaks the code. break; } } sc.close(); } }
THIS IS THE TEXT FILE IT RAN OFF WITH:
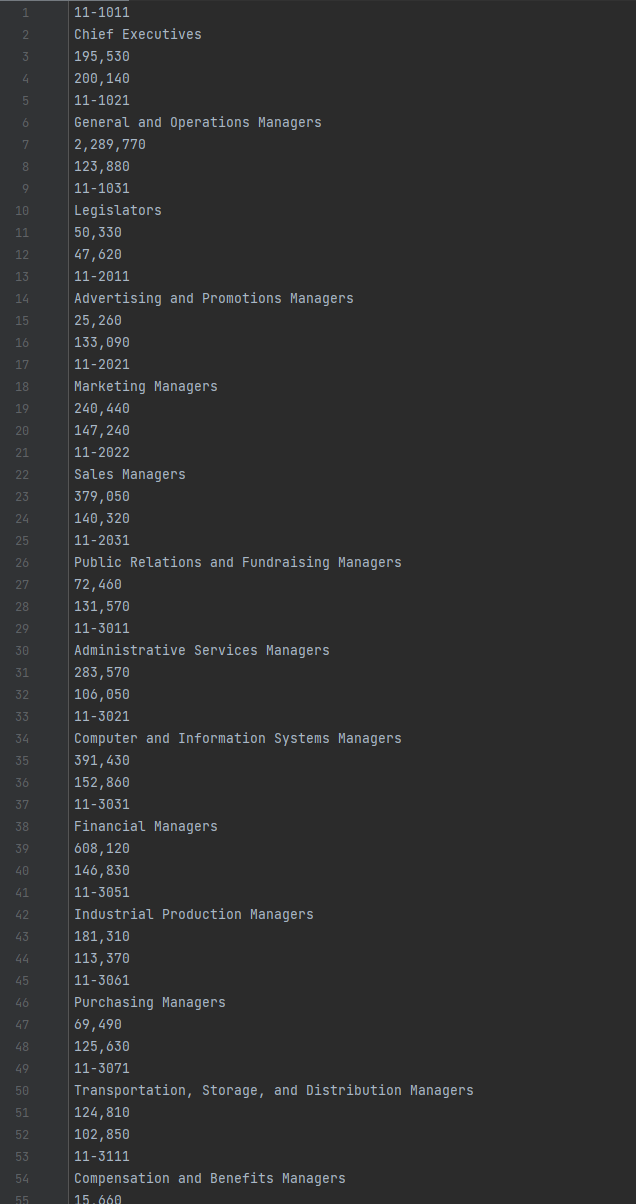
1 11-1011 2 Chief Executives 3 195,530 4 200,140 5 11-1021 6 7 General and Operations Managers 2,289,770 8 123,880 9 11-1031 10 Legislators 11 50,330 12 47,620 13 11-2011 14 15 Advertising and Promotions Managers 25,260 16 133,090 17 11-2021 18 Marketing Managers 19 240,440 20 147,240 21 11-2022 22 Sales Managers 23 379,050 24 140,320 25 11-2031 26 Public Relations and Fundraising Managers 27 72,460 28 131,570 29 11-3011 30 Administrative Services Managers 31 283,570 32 106,050 33 11-3021 34 Computer and Information Systems Managers 35 391,430 36 152,860 37 11-3031 38 Financial Managers 39 608,120 40 146,830 41 11-3051 42 43 Industrial Production Managers 181,310 44 113,370 45 11-3061 46 Purchasing Managers 47 69,490 48 125,630 49 11-3071 50 Transportation, Storage, and Distribution Managers 51 124,810 52 102,850 53 11-3111 54 Compensation and Benefits Managers 55 15.660
Step by Step Solution
There are 3 Steps involved in it
Step: 1
I Used Online Java Compiler OUTPUT CODE import javautil import javautilArrays Driver Class public class Driver Driver Main Method public static void mainString args SystemoutprintString Systemoutprint ...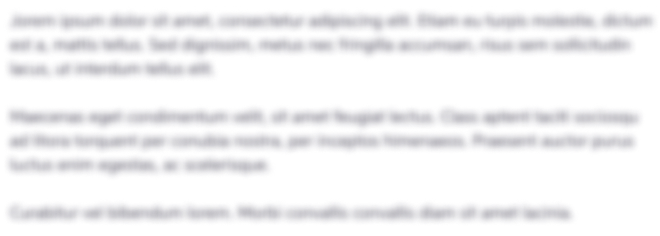
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started