Question
Your task is to complete the following function/functions: 1. Given a position in the linked list, delete the node at that position.(Silver problem - Mandatory
Your task is to complete the following function/functions: 1. Given a position in the linked list, delete the node at that position.(Silver problem - Mandatory ) 2. Print the sum of all negative elements in the linked list.(Gold problem) If you want, you can refer to the the previous recitation manual (which was on Linked Lists) to implement node deletion.
#include
using namespace std;
//----------- Define Node --------------------------------------------------- struct Node{ int key; Node *next; };
//----------- Define Linked List --------------------------------------------------- class LinkedList { private: Node *head;
public: LinkedList(){ head = NULL; } void insert_begin(int newKey); void insert_end(int newKey); int negativeSum(); bool deleteAtIndex(int index); bool deleteAtHead(); void printList(); };
void LinkedList::insert_begin(int newKey){ //1. ALLOCATE NODE Node* newNode = new Node;
//2. PUT IN THE DATA newNode->key = newKey;
//3. Make next of newNode as head newNode->next = head;
//4. Change the head to newNode head = newNode; }
void LinkedList::insert_end(int newKey){ //1. ALLOCATE NODE Node* newNode = new Node;
//2. PUT IN THE DATA newNode->key = newKey;
//3. Make next of newNode as NULL newNode->next = NULL;
//4. Check if head is not Null if(head == NULL){ head = newNode; return; }
//4. Traverse the LinkedList till end Node* temp = head;
while(temp->next != NULL){ temp = temp->next; } temp->next = newNode; return; }
//TODO int LinkedList::negativeSum(){ if(head == NULL) return 0;
int total = 0; int count = 0;
// TODO Complete this function
cout<<"There is(are) "< } // Use deleteAtHead to delete the first item in the list bool LinkedList::deleteAtHead() { bool isDeleted = false; if(head == NULL){ cout<< "List is already empty"< Node *temp = head; head = temp->next; delete temp; isDeleted = true; return isDeleted; } //TODO bool LinkedList::deleteAtIndex(int n) { bool isDeleted = false; if(head == NULL){ cout<< "List is already empty"< Node *pres = head; Node *prev = NULL; //TODO Complete this function //isDeleted = true; return isDeleted; } void LinkedList::printList(){ Node* temp = head; while(temp->next != NULL){ cout<< temp->key <<" -> "; temp = temp->next; } cout< int main() { LinkedList li; cout<<"Adding nodes to List:"< cout<<"Running delete function."< /******************************* Silver Problem - Implement the deleteAtIndex function ********************************/ cout<<"Deleting node at index:"< if(!li.deleteAtHead()) { cout<<"Delete failed!"< cout<<"Get the sum of negatives"< /******************************* Gold Problem - Implement the negativeSum function ********************************/ int negsum; negsum = li.negativeSum(); cout<<"Sum of all the negative elements is "< }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
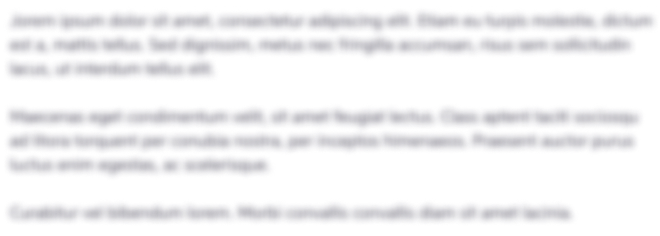
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started