Question
Your task is to write a program, called calc.c, which evaluates an arithmetic expression given on the command line. For example, here is a screenshot
Your task is to write a program, called calc.c, which evaluates an arithmetic expression given on the command line. For example, here is a screenshot of the program in action:
Note that I use square brackets for parentheses and "x" to denote multiplication. This is because the parenthesis and asterisk characters have special meanings when they appear on the command line. Use the word "token" to denote an operator, bracket, or integer in the expression. You should assume that the tokens on the command line are separated from each other by spaces. Then the argv array will contain one element for each token. You probably learned how to do expression evaluation in your data structures class. The idea is to use two stacks: a stack of strings (called the "operand stack") and a stack of integers (called the "value stack"). Use the following algorithm:
Note that this algorithm is overly simple. For example, it does not take into account operator precedence. For the purpose of this assignment, that is all we need. Your program needs to implement two operators: "+" for addition, and "x" for multiplication. These operations both take two operands. You should implement each stack as an array. The value stack is simply an array of ints. The operand stack should be an array of char * pointers that is, each element of the array denotes a string. You can assume that each stack will never grow larger than 50 elements. You should associate an integer with each stack that keeps track of the top of the stack. Your code must implement the following functions:
void pushValStack (int stack[], int *top, int value);
int popValStack (int stack[], int *top);
void pushOpStack (char *stack[], int *top, char *value);
char *popOpStack (char *stack[], int *top);
Use these functions when you need to push and pop one of the stacks. You are of course free to write additional functions as well. It is possible for your program to blow up for example, your input expression could have unbalanced parentheses. You are not required to check for bad input.
adminuser@adminuser-VirtualBox /Desktop s calc 200 100 x 5 ] 700 adminuser@admnuser-virtualBox ~/Desktop $ Galc [ 2 .#. )I x [ :L.:. l 90 adminuser@adminuser-VirtualBox -/Desktop $ For each token t: If t is an operator or a left bracket, push it on the operand stack. Else if t is a right bracket: Repeatedly pop the operand stack until you hit a left bracket. For each operator found: Pop the value stack twice to get the operands. Perform the operation on these operands Push the result of the operation on the value stack. Else t denotes a number. Convert t to an integer, and push it on the value stack. When you are out of tokens, repeatedly pop the operand stack until it is empty, performing each operation as before. At this point there should be one number on the value stack, which is the answer adminuser@adminuser-VirtualBox /Desktop s calc 200 100 x 5 ] 700 adminuser@admnuser-virtualBox ~/Desktop $ Galc [ 2 .#. )I x [ :L.:. l 90 adminuser@adminuser-VirtualBox -/Desktop $ For each token t: If t is an operator or a left bracket, push it on the operand stack. Else if t is a right bracket: Repeatedly pop the operand stack until you hit a left bracket. For each operator found: Pop the value stack twice to get the operands. Perform the operation on these operands Push the result of the operation on the value stack. Else t denotes a number. Convert t to an integer, and push it on the value stack. When you are out of tokens, repeatedly pop the operand stack until it is empty, performing each operation as before. At this point there should be one number on the value stack, which is theStep by Step Solution
There are 3 Steps involved in it
Step: 1
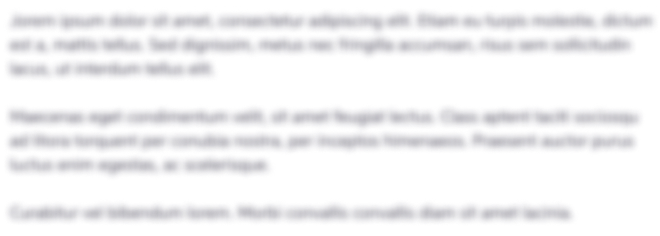
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started