Question
Your task is to write a program in JAVA that will output colored shape vertices in CSV format (comma-separated value[1]; something Microsoft Excel can open!).
Your task is to write a program in JAVA that will output colored shape vertices in CSV format (comma-separated value[1]; something Microsoft Excel can open!). To build up to this capability, you will be implementing a set of classes, including two subclasses. Here is the recommended sequence:
- To start, implement the Point2D class. It is quite similar to the class in a previous assignment, but now has you override the equals method to detect points that represent the same location, within a distance threshold.
- Then implement the Rectangle class, which is a subclass of Shape2D (provided for you). You will use simple arithmetic to implement such functionality as computing area, perimeter, and center point. The vertices (think corner points) for a rectangle are expected to start at the lower-left corner and proceed clockwise. Two rectangles are considered equal if they have lower-left and upper-right points that are equal.
- Now move on to the Triangle class, which is also a subclass of Shape2D. The math here can get a little more complex for area[2] and center point (centroid[3]). An axis-aligned bounding box (AABB[4]) is simply the rectangle that most closely bounds the vertices of the triangle. The vertices should be returned in the order they were provided in the constructor.
- Now move on to the PA4b class. The shapeVertices method receives an array of Shape2D objects and must produce a string which includes all the vertices in CSV format (on each line: "color",x,y). If the method encounters a triangle object, it should include the vertices of the AABB after the triangle. This is an opportunity to experiment with polymorphic code. When this method is complete, proceed to main validate command-line arguments for a single colored triangle and use the method you just completed to output the corresponding CSV to the terminal.
Here are example runs of a completed program:
$ java edu.wit.cs.comp1050.PA4b
Please supply correct inputs: color x1 y1 x2 y2 x3 y3
$ java edu.wit.cs.comp1050.solution.PA4b blue 0 0 0 1 1 0
"blue",0.000,0.000
"blue",0.000,1.000
"blue",1.000,0.000
"blue",0.000,0.000
"blue",0.000,1.000
"blue",1.000,1.000
"blue",1.000,0.000
Note: if you copy-paste the output into a file (typically with a .csv extension) and open it in Excel, you could then plot the last two columns using an XY plot!
-------------------------------------------------------------
THIS IS THE CODE OF THE PREVIOUS PROBLEM IN PART 1
public class Point2D { private double x; private double y; /** * Constructor to initialize coordinates * * @param x x value * @param y y value */ public Point2D(double x, double y) { this.x = x; this.y = y; } /** * Initializes to (0., 0.) */ public Point2D() { new Point2D(0.0, 0.0); } /** * Get the x coordinate * * @return x coordinate */ public double getX() { return x; // replace with your code } /** * Get the y coordinate * * @return y coordinate */ public double getY() { return y; // replace with your code } /** * Gets a String representation * of the coordinate in the form * "(x, y)" (each with three decimal * places of precision) * * #return "(x, y)" */ public String toString() { //String.format(format, args) return "(" + String.format("%.3f", x) + "," + String.format("%.3f", y) + ")"; // replace with your code } /** * Method to compute the Euclidean/L2 * distance between two points in 2D * space * * @param p1 point 1 * @param p2 point 2 * @return straightline distance between p1 and p2 */ public static double distance(Point2D p1, Point2D p2) { return Math.sqrt((p2.x - p1.x) * (p2.x - p1.x) + (p2.y - p1.y) * (p2.y - p1.y)); // replace with your code } /** * Method to compute the Euclidean * distance between this point * and a supplied point * * @param p input point * @return straightline distance between this point and p */ public double distanceTo(Point2D p) { return Math.sqrt((p.x - x) * (p.x - x) + (p.y - y) * (p.y - y)); // MUST be a single call to the static distance method } }
public static void main(String[] args) { Point2D p1 = new Point2D(); Point2D p2 = new Point2D(Double.parseDouble(args[0]),Double.parseDouble(args[1])); final Point2D p = new Point2D(); System.out.printf("Point 1: %s%n", o); System.out.printf("Point 2: %s%n", p); System.out.printf("Static method distance: %.3f%n", Point2D.distance(o, p)); System.out.printf("Distance from P1: %.3f%n", o.distanceTo(p)); System.out.printf("Distance from P2: %.3f%n", p.distanceTo(o)); } }public class PA4b Error if incorrect command-line arguments are supplied public static final String ERR USAGE"Please supply correct inputs: color x1 yl x2 y2 x3 y3" Number of command-line arguments public static final int NUM ARGS-7 Produces a string representing all vertex information in CSV format: "color"x.y For al1 shape vertices, including axis-aligned bounding boxes for any included triangles @param shapes array of shapes return string of CSV information public static String shapeVertice (Shape2D[] shapes) return 1/ replace with your code (use a StringBuilder for efficiency!) Outputs vertex information in CSV format about the triangle supplied via command-line arguments, including its axis-aligned bounding box @param args command-line arguments: color x1 y1 x2 y2 x3 y3 public static void main(String[] args) // replace with your code public class Point2D Constructor to initialize coordinates @param xx value @param y y value public Point2D (double x, double y) // replace with your code Get the x coordinate @return x coordinate public double getX) return ; // replace with your code : Get the y coordinate @return y coordinate public double getY) return ; // replace with your code Gets a String representation of the coordinate in the form "(x, y)" (each with three decimal places of precision) @return "(x, y)" @Override public String toString) return 17 replace with your code Returns true if provided another point that is at the same (x,y) location (that is, the distance is "close enoueh" accordine to @Override public String tostring) return " replace with your code Returns true if provided another location (that is, the distance Shape2D) param o another object *point that is at the same (x,y) shh according to @return true if the other object is a point and the same location (within threshold) @Override public boolean equals (Object o) return false; // replace with your code * Method to compute the Euslidean/L2 " distance between two points in 2D space @param p1 point 1 @param p2 point 2 @return stxaiahtilins distance between p1 and p2 public static double distance (Point2D p1, Point2D p2) return e // replace with your code * Method to compute the Eualidean " distance between this point " and a supplied point @param p input point @return stxaiahtilins distance between this point andp public double distanceTo (Point2D p) t return 0; /7 MUST call distance above with this point public class Rectangle extends Shape 2D Constructs a rectangle given two pointsl @param color rectangle color @param p1 point 1 @param p2 point 2 public Rectangle(String color, Point2D p1, Point2D p2) super(color, "); 17 replace with your code Returns true if provided another rectangle whose lower-left and upper-right points are equal to this rectangle @param o another object @return true if the same rectangle @Override public boolean equals (Object o) return false; 1/ replace with your code Gets the lower-left corner @return lower-left corner public Point2D getLowerLeft() return null; // replace with your code Gets the upper-right corner @return upper-right corner public Point2D getupperRight) return null; // replace with your code eparam o another object Oreturn true if the same rectangle Override public boolean equals (Object o) return false;// replace with your code Gets the lower-left corner @return lower-left corner public Point2D getLowerleft) return null;// replace with your code * Gets the upper-right corner @return upper-right corner public Point2D getupperRight) return null; // replace with your code Override public double getArea) return e; 17 replace with your code Override public double getPerimeter) return ; // replace with your code @Override public Point2D getCenter) ) t return null; // replace with your code Override public Point2D[] getVertices) return null; /7 replace with your code public abstract class Shape 2D f final private String color; final private String shapeName; final public static double THRESH-1.0E-5; Returns true if two numbers are considered close enough @param a value 1 @param b value 2 @return true if a and b are considered close enough public static boolean closeEnough(double a, double b) return Math.abs (a-b)THRESH; Constructs the shape @param color shape color @param shapeName name of the shape (e.g. Triangle, Rectangle, etc.) public Shape2D (String color, String shapeName) this.color-color; this.shapeName shapeName; * Gets the color of the shape @return shape color final public String getColor() return color; private String vTostring)t final StringBuilder sb-new StringBuilder); final Point2D[] v getVertices(); if (v.length>)f sb.append (v[]) for (int sb.append(string.format(". a];
Step by Step Solution
There are 3 Steps involved in it
Step: 1
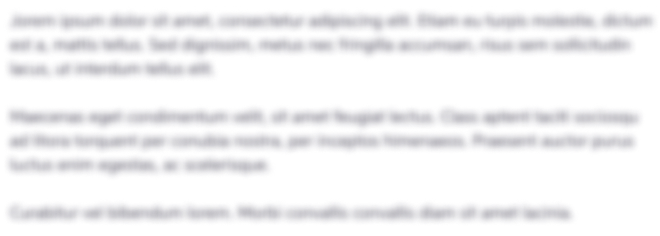
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started