Question
Your task This project is more like one of the accompanying homeworks. There are five problems. In the problems that ask you to change code,
Your task
This "project" is more like one of the accompanying homeworks. There are five problems. In the problems that ask you to change code, make the few changes necessary to fix the code without changing its overall approach. For example, don't fix the program in problem 1a by changing it to
int main() { cout << "1000 100 10 1" << endl; }
Problem 1: This problem involves errors related to the use of pointers.
a. This program is supposed to write 1000 100 10 1 , but it doesn't. Find all of the bugs and show a fixed version of the program:
int main() { int arr[4] = { 0, 1, 2, 3 }; int* ptr = arr;
*ptr = arr[ 1 ]; // set arr[0] to 1 *ptr + 1 = arr[ 0 ] * 10; // set arr[1] to 10 ptr += 2; ptr[0] = arr[ 1 ] * 10; // set arr[2] to 100 ptr[1] = 1000; // set arr[3] to 1000
while (ptr >= arr) { ptr--; cout << " " << *ptr; // print values } cout << endl; return( 0 ); }
b. The findLastZero function is supposed to find the last element in an array whose value is zero, and sets the parameter p to point to that element, so the caller can know the location of that element holding the value zero. Explain why this function won't do that, and show how to fix it. Your fix must be to the function only; you must not change the main routine below in any way. As a result of your fixing the function, the main routine below needs to work correctly.
void findLastZero(int arr[], int n, int* p) { p = nullptr; /// default value if there isn't a 0 in the array at all for (int k = n - 1; k >= 0; k--) { if (arr[k] == 0) // found an element whose value is 0 { p = arr + k; // change the value of p break; // stop looping and return } } }
int main() { int nums[6] = { 10, 20, 0, 40, 30, 50 }; int* ptr;
findLastZero(nums, 6, ptr); if (ptr == nullptr) { cout << "The array doesn't have any zeros inside it." << endl; } else { cout << "The last zero is at address " << ptr << endl; cout << "It's at index " << ptr - nums << endl; cout << "The item's value is " << *ptr << " which is zero!" << endl; } return( 0 ); }
c. The biggest function is correct, but the main function has a problem. Explain why it may not work, and show a way to fix it. Your fix must be to the main function only; you must not change the biggest function in any way.
#include
void biggest(int value1, int value2, int * resultPtr) { if( value1 > value2 ) { *resultPtr = value1; } else { *resultPtr = value2; } }
int main() { int* p; biggest(15, 20, p); cout << "The biggest value is " << *p << endl; return( 0 ); }
d. The match function is supposed to return true if and only if its two C-string arguments have exactly same text. Explain what the problems with the implementation of the function are, and show a way to fix them.
// return true if two C strings are equal bool match(const char str1[], const char str2[]) { bool result = true; while (str1 != 0 && str2 != 0) // zero bytes at ends { if (str1 != str2) // compare corresponding characters { result = false; break; } str1++; // advance to the next character str2++; } if (result) { result = (str1 == str2); // both ended at same time? } return( result ); }
int main() { char a[10] = "pointy"; char b[10] = "pointless";
if (match(a,b)) { cout << "They're the same!" << endl; } }
e. This program is supposed to write 1 1 2 3 5 8 13 21 but it probably does not. What is the program doing that is incorrect? (We're not asking you explain why the incorrect action leads to the particular outcome it does, and we're not asking you to propose a fix to the problem.)
#include
int fibonacci( int n ) { int tmp; int a = 1; int b = 1;
for (int i = 0; i < n-2; i++) { tmp = a+b; a = b; b = tmp; } return b; }
int* computeFibonacciSequence(int& n) { int arr[8]; n = 8; for (int k = 0; k < n; k++) { arr[k] = fibonacci( k ); } return arr; }
int main() { int m; int* ptr = computeFibonacciSequence(m); for (int i = 0; i < m; i++) { cout << ptr[i] << ' '; } return( 0 ); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
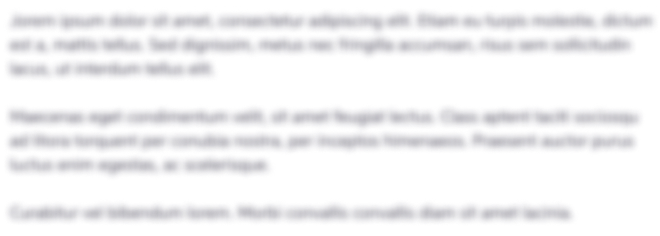
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started