Question
Your tasks for this lab include a. Writing classes InternetThing, SmartPhone and LightBulb b. Editing in the SmartHome class to refer to newly defined classes
Your tasks for this lab include a. Writing classes InternetThing, SmartPhone and LightBulb b. Editing in the SmartHome class to refer to newly defined classes c. Editing the Refrigerator class to override the getPowerUse method.
Write code for the InternetThing class to complete: a. A constructor that accepts a manufacturer and a serial number as its parameter. This constructor performs the following actions: i Store the serial number as a string ii Set a unique id number as an integer(hint- use the nextId). iii Set the IP address to the value 192.168.0.+idnumber iv Set a local representation of a the power used in mW to the integer 1. v Set the password to admin. vi Prints the value Created + the result of the toString() method after assigning all instance data. vii Increment the number of things on record. b. Accessors for all private variables Ensure the accessors for id, password and powerUse are named getId(), getPassword() and getPowerUse() c. A method setPassword that accepts a string argument and sets the instance password to the argument. All instance variables, except the password, should be private. The password should be protected.
In class SmartHome, method addThing, Create a refrigerator object by calling the relevant constructor with the following arguments (in order... manufacturer, serialNo, basePower, powerRating, capacity) Set the value in array things, at the position that matches the ID of the refrigerator ie (rf.getId()), to the refrigerator object.
Write code for SmartPhone class which is an InternetThing that includes: a. A constructor which accepts a manufacturer(string), ), a serial number(String) , a model(String) and the number of associated megapixels(int) as its parameters. This constructor shall store the each argument appropriately and will set the phones status to locked. After assigning all instance data, the constructor should print the value Created + the result of the toString method. b. A method named setPassword that accepts two strings (old password and new password) as its parameters. The method shall change the password to the new password value only if the old password given as a parameter matches the password stored on the phone, and if the phone is unlocked. If the password is successfully changed, the method will print the value Successfully changed password for + the result of the toString method c. A method named lock that will lock the phone(), and print the value Locked + the result of the toString method. d. A method named isLocked that returns the value true is the phone is locked. e. A method named unlock that accepts a string (the password) as its parameter and unlocks the phone only if the password is correct. If the phone becomes unlocked the method should print Unlocked + the result of the toString method. f. A toString method that returns data in the format "Thing#"+getId()+"::PHONE made by "++":Model="++@IP:+getIPAddress();
Write code for a LightBulb class that is an InternetThing which includes: a. A constructor that accepts a manufacturer(String), a serial number(String), and a lumenCount(int) then sets values in the arguments parameter appropriately, and sets a property that reflects whether the light is on to false. After assigning all instance data, the constructor should print the value Created + the result of the toString method. b. A method named turnOn() that turns on the light. The method should then print Turned on + the result of the toString method. c. A method named turnOff() that turns off the light. . The method should then print Turned off + the result of the toString method. d. In class SmartHome, method addThing, at the secton where the argument is checked for LIGHTBULB, instantiate a LightBulb with a reference called lb, and then uncomment the lines in the below that add lb to things, then return the id.
In class LightBulb, override the method named getPowerUse() that evaluates current power used by the lightbulb as the product of its lumenCount and the power use of its base class if the light is on. If the light is off, getPowerUse() returns 0.
In the Refrigerator class, override the method getPowerUse() so that it returns a value that is equal to basePowerUse+contentSize*rating.
In the SmartHome class, Observe the operation of the method showAllItems and then complete the method sumAllPower(). For each item that is assessed while evaluating the total power, the method should print the power used by the item, a tab, and then the result of the toString method. At the end of the calculation, the method should output its result in the format "TOTAL POWER = "+sumPower+"mW",where sumPower represents the aggregated power use.
Submission (You will not get marks for this submission. The submission is practice for actions you will need to perform when submitting project1 your marks for the lab are earned from the Hackerrank exercise and from the scores assigned by lab techs) a. Save all classes in a separate file that matches the name of the class b. Zip the files, and make the name of your zip file Lab3..zip. c. Submit the zip file to the submission link for Lab 3.
Input Format
The first line of input represent t, the number of InternetThings to be added. Each InternetThing is a member of the set {REFRIGERATOR,SMARTPHONE, LIGHTBULB}. For each InternetThing the parameters sufficient to describe it are placed on the same line, and the following line specifies an integer a, the number of actions to be carried out on the InternetThing. Each action specifies it's required parameters.
After all InternetThings have been defined, the testcase closes with a symbol SMARTHOME, and a set of subsequent actions. The line following SMARTHOME contains an integer s, which describes the number of actions that will be applied to the SmartHome.
Constraints
t<10, a<10, s<10
Output Format
See test cases
Sample Input 0
0 SMARTHOME 1 showAllThings
Sample Output 0
Created Thing#0:made by BASENET@IP:192.168.0.0 Created Thing#1:made by BASENET@IP:192.168.0.1 Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1 ===============SHOWING ALL 2 THINGS=============== Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1
Sample Input 1
1 REFRIGERATOR MABE MB01 100 5 500 4 Insert 90 Insert 100 Remove 50 Insert 250 SMARTHOME 0
Sample Output 1
Created Thing#0:made by BASENET@IP:192.168.0.0 Created Thing#1:made by BASENET@IP:192.168.0.1 Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1 Created Thing#2::REFRIGERATOR, made by MABE:BasePower=0.0:Rating=0.0@IP:192.168.0.2 Created Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Removed from Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2
Sample Input 2
3 SMARTPHONE Samsung SM01 Galaxy 20 0 SMARTPHONE Motorola MT01 Storm 5 7 unlock admin setpassword admin moto1 setpassword moto1 pass2 lock setpassword moto admin unlock admin setpassword admin pass3 SMARTPHONE Alcatel AC01 Lynx 3 1 unlock admin
Sample Output 2
Created Thing#0:made by BASENET@IP:192.168.0.0 Created Thing#1:made by BASENET@IP:192.168.0.1 Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1 Created Thing#2::PHONE made by Samsung:Model=null@IP:192.168.0.2 Created Thing#2::PHONE made by Samsung:Model=Galaxy@IP:192.168.0.2 Created Thing#3::PHONE made by Motorola:Model=null@IP:192.168.0.3 Created Thing#3::PHONE made by Motorola:Model=Storm@IP:192.168.0.3 Unlocked Thing#3::PHONE made by Motorola:Model=Storm@IP:192.168.0.3 Successfully changed password for Thing#3::PHONE made by Motorola:Model=Storm@IP:192.168.0.3 Successfully changed password for Thing#3::PHONE made by Motorola:Model=Storm@IP:192.168.0.3 Locked Thing#3::PHONE made by Motorola:Model=Storm@IP:192.168.0.3 Created Thing#4::PHONE made by Alcatel:Model=null@IP:192.168.0.4 Created Thing#4::PHONE made by Alcatel:Model=Lynx@IP:192.168.0.4 Unlocked Thing#4::PHONE made by Alcatel:Model=Lynx@IP:192.168.0.4
Sample Input 3
2 LIGHTBULB BULBMAKER BM01 100 1 turnOn LIGHTBULB BULBMAKER BM02 50 4 turnOn turnOff turnOn turnOff SMARTHOME 0
Sample Output 3
Created Thing#0:made by BASENET@IP:192.168.0.0 Created Thing#1:made by BASENET@IP:192.168.0.1 Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1 Created Thing#2:made by BULBMAKER@IP:192.168.0.2 Created Thing#2:made by BULBMAKER@IP:192.168.0.2 Turned on Thing#2:made by BULBMAKER@IP:192.168.0.2 Created Thing#3:made by BULBMAKER@IP:192.168.0.3 Created Thing#3:made by BULBMAKER@IP:192.168.0.3 Turned on Thing#3:made by BULBMAKER@IP:192.168.0.3 Turned off Thing#3:made by BULBMAKER@IP:192.168.0.3 Turned on Thing#3:made by BULBMAKER@IP:192.168.0.3 Turned off Thing#3:made by BULBMAKER@IP:192.168.0.3
Sample Input 4
2 LIGHTBULB BULBMAKER BM01 100 2 turnOn showpower LIGHTBULB BULBMAKER BM02 50 1 showpower SMARTHOME 0
Sample Output 4
Created Thing#0:made by BASENET@IP:192.168.0.0 Created Thing#1:made by BASENET@IP:192.168.0.1 Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1 Created Thing#2:made by BULBMAKER@IP:192.168.0.2 Created Thing#2:made by BULBMAKER@IP:192.168.0.2 Turned on Thing#2:made by BULBMAKER@IP:192.168.0.2 100mW Thing#2:made by BULBMAKER@IP:192.168.0.2 Created Thing#3:made by BULBMAKER@IP:192.168.0.3 Created Thing#3:made by BULBMAKER@IP:192.168.0.3 0mW Thing#3:made by BULBMAKER@IP:192.168.0.3
Sample Input 5
1 REFRIGERATOR MABE MB01 100 5 500 5 Insert 90 Insert 100 Remove 50 Insert 250 showpower SMARTHOME 0
Sample Output 5
Created Thing#0:made by BASENET@IP:192.168.0.0 Created Thing#1:made by BASENET@IP:192.168.0.1 Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1 Created Thing#2::REFRIGERATOR, made by MABE:BasePower=0.0:Rating=0.0@IP:192.168.0.2 Created Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Removed from Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 2600mW Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2
Sample Input 6
6 REFRIGERATOR MABE MB01 100 5 500 5 Insert 90 Insert 100 Remove 50 Insert 250 showpower SMARTPHONE Samsung SM01 Galaxy 20 1 showpower SMARTPHONE Motorola MT01 Storm 5 7 unlock admin setpassword admin moto1 setpassword moto1 pass2 lock setpassword pass2 admin unlock admin setpassword admin pass3 SMARTPHONE Alcatel AC01 Lynx 3 2 unlock admin showpower LIGHTBULB BULBMAKER BM01 100 1 turnOn LIGHTBULB BULBMAKER BM02 50 4 turnOn turnOff turnOn turnOff SMARTHOME 2 showAllThings showAllPower
Sample Output 6
Created Thing#0:made by BASENET@IP:192.168.0.0 Created Thing#1:made by BASENET@IP:192.168.0.1 Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1 Created Thing#2::REFRIGERATOR, made by MABE:BasePower=0.0:Rating=0.0@IP:192.168.0.2 Created Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Removed from Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Inserted to Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 2600mW Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Created Thing#3::PHONE made by Samsung:Model=null@IP:192.168.0.3 Created Thing#3::PHONE made by Samsung:Model=Galaxy@IP:192.168.0.3 1mW Thing#3::PHONE made by Samsung:Model=Galaxy@IP:192.168.0.3 Created Thing#4::PHONE made by Motorola:Model=null@IP:192.168.0.4 Created Thing#4::PHONE made by Motorola:Model=Storm@IP:192.168.0.4 Unlocked Thing#4::PHONE made by Motorola:Model=Storm@IP:192.168.0.4 Successfully changed password for Thing#4::PHONE made by Motorola:Model=Storm@IP:192.168.0.4 Successfully changed password for Thing#4::PHONE made by Motorola:Model=Storm@IP:192.168.0.4 Locked Thing#4::PHONE made by Motorola:Model=Storm@IP:192.168.0.4 Created Thing#5::PHONE made by Alcatel:Model=null@IP:192.168.0.5 Created Thing#5::PHONE made by Alcatel:Model=Lynx@IP:192.168.0.5 Unlocked Thing#5::PHONE made by Alcatel:Model=Lynx@IP:192.168.0.5 1mW Thing#5::PHONE made by Alcatel:Model=Lynx@IP:192.168.0.5 Created Thing#6:made by BULBMAKER@IP:192.168.0.6 Created Thing#6:made by BULBMAKER@IP:192.168.0.6 Turned on Thing#6:made by BULBMAKER@IP:192.168.0.6 Created Thing#7:made by BULBMAKER@IP:192.168.0.7 Created Thing#7:made by BULBMAKER@IP:192.168.0.7 Turned on Thing#7:made by BULBMAKER@IP:192.168.0.7 Turned off Thing#7:made by BULBMAKER@IP:192.168.0.7 Turned on Thing#7:made by BULBMAKER@IP:192.168.0.7 Turned off Thing#7:made by BULBMAKER@IP:192.168.0.7 ===============SHOWING ALL 8 THINGS=============== Thing#0:made by BASENET@IP:192.168.0.0 Thing#1:made by BASENET@IP:192.168.0.1 Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 Thing#3::PHONE made by Samsung:Model=Galaxy@IP:192.168.0.3 Thing#4::PHONE made by Motorola:Model=Storm@IP:192.168.0.4 Thing#5::PHONE made by Alcatel:Model=Lynx@IP:192.168.0.5 Thing#6:made by BULBMAKER@IP:192.168.0.6 Thing#7:made by BULBMAKER@IP:192.168.0.7 ===============SHOWING ALL POWER=============== 1 Thing#0:made by BASENET@IP:192.168.0.0 1 Thing#1:made by BASENET@IP:192.168.0.1 2600 Thing#2::REFRIGERATOR, made by MABE:BasePower=100.0:Rating=5.0@IP:192.168.0.2 1 Thing#3::PHONE made by Samsung:Model=Galaxy@IP:192.168.0.3 1 Thing#4::PHONE made by Motorola:Model=Storm@IP:192.168.0.4 1 Thing#5::PHONE made by Alcatel:Model=Lynx@IP:192.168.0.5 100 Thing#6:made by BULBMAKER@IP:192.168.0.6 0 Thing#7:made by BULBMAKER@IP:192.168.0.7 TOTAL POWER = 2705mW
Contest ends in 5 days
refrigerator class below:
///* UNCOMMENT AFTER IMPLEMENTING InternetThing class Refrigerator extends InternetThing {
double basePower; double powerRating; int capacity; int contents =0;
public Refrigerator(String manufacturer,String serialNumber, double basePower,double powerRating, int capacity) { super(manufacturer, serialNumber); this.basePower = basePower; this.powerRating= powerRating; this.capacity = capacity; this.contents = 0; System.out.println("Created "+this.toString()); }
public void insertItem(int size) { if (contents+size public void removeItem(int size) { if (contents-size>0) { contents-=size; System.out.println("Removed from "+ this.toString()); } } public String toString() { return "Thing#"+getId() +"::REFRIGERATOR, made by "+getManufacturer()+":BasePower="+basePower+":Rating="+powerRating+"@IP:"+getIPAddress(); } //QUESTION 5. Overload getPowerUse() in Refrigerator //Pass case4 by completing getPowerUse here }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
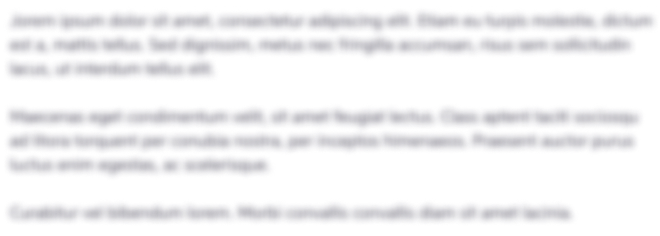
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started