Question
You're working a software engineering team that has been contracted to reprogram your universities database management system. Your team has decided to use Object Oriented
You're working a software engineering team that has been contracted to reprogram your universities database management system. Your team has decided to use Object Oriented Programming to represent the entities that exist within the database. Your colleague has written the abstract class Academic and the enum Title (both provided) as a starting point for this.
Abstract classes are similar to interfaces in that they contain unimplemented methods that must then be implemented by all subclasses. Unlike interfaces, however, abstract classes may also contain already implemented methods and fields. Methods that are not implemented are denoted by the abstract modifier. Abstract classes are useful if you want to specify a contract (like an interface), but also implement some general behaviour at the superclass level (because such behaviour is common to all subclasses anyway). Here, your colleague has decided that getName(), getID(), and getTitle() are methods that all subclasses would use, and so he has already implemented them. However, getWeeklyPay() and toString() are more complex and the details of such behaviour are only known at the subclass level (as discussed below).
While utilizing the provided Title enum, you task is to create two subclasses of Academic:
Student(String name, int id, Title title, double stipend)
Staff(String name, int id, Title title)
Because these are subclasses, they can call their superclass's constructor via the super keyword. Super construction should be used where possible to reduce code redundancy. This is because subclasses "extend" superclasses, and therefore have access to all the fields and methods of their superclass. After super is called, you may write additional code within the subclass constructor to express behaviour that is specific to the subclass.
Notice how, when you create these subclasses, Eclipse will automatically populate them with the necessary methods getWeeklyPay() and toString() as these methods are required by the Academic contract. You do not need to create any more methods (public or private) to complete this exercise, you should take advantage of the already implemented methods in Academic when required.
toString()
toString() should return a string representation of the object based on its class, id, and title; formatted as:
[class] [id] (studies a) / (works as a) [title]
For example:
Student 4 studies a Bachelor degree.
Student 16 studies a Masters degree.
Student 8 studies a PhD.
Staff 32 works as a tutoor.
Staff 64 works as a lecturer.
To get the [title] component necessary for the string, you can simply use this.getTitle().toString();
Notice how we can use this.getTitle() without needing to write a getTitle() method in the current class, because it has already been implemented in the superclass Academic! This requires that you have implemented the constructor correctly using the super keyword (ask your tutorr for guidance with this if you're not sure!).
You should also inspect how the toString() method in Title works. It is very similar to PizzaType in oop.PizzaModified. Each of the enum values (e.g. BACHELOR_STUDENT) call the constructor Title(String text) which then assigns an internal field that can be retrieved with toString().
getWeeklyPay()
getWeeklyPay() is calculated based on the following information:
Students get paid a fixed weekly salary known as a stipend (see the Student constructor).
Lecturers get paid an annual salary of $80,000.
Tutoors get paid an hourly rate of $40.
Because tutoors are paid hourly, you must implement an additional method public void setHours(int hours) in the Staff subclass that will allow administrators to set weekly hours for tutoors.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
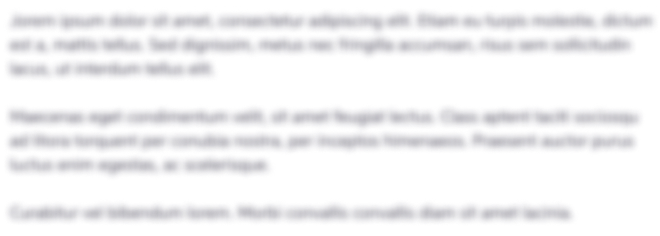
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started