Question
Write a program that will determine the letter grade of students using understanding of java class, objects, exception handling, and collection in java Your program
Write a program that will determine the letter grade of students using understanding of java class, objects, exception handling, and collection in java Your program will need to accept two command line arguments. The first argument withe name of a disk file that contains the names of students, and their test scores, separated by commas follows by one or more spaces. Each line in the file will contain scores for one student. The second argument argument will be the name of an output disk file. Your program will create a new output disk file using that name. The output file will have grade information of all students whose information was read from input file, in a sorted order. You will be writing one line in the output file for one student. You will write name of one student and the letter grade he/she got in the class in each line (you should format the texts in the output file). Calculation: The test scores are weighed. There are four quizzes, 40% total, midterm I is 20%, midterm II is 15% and the final is 25%. All scores in the input file are recorded out of 100. You need to apply the weight for each score in the program to calculate the final score. The final score is tabulated as follows: Final Score = quiz1*.10 + quiz2*.10 + quiz3*.10 + quiz4*.10 + quiz1*.10 + mid1*.20 + mid2*.15 + Final*.25. Final Score >= 90% letter grade is A, 80%--89% B, 70%--79% C, 60--69%D, <= 59% F
Sample input.txt file : name, quiz1, quiz2, quiz3, quiz4, mid1, mid2, final
Rock Smith, 100, 90, 80, 100, 89, 99, 88
Baker Adam, 90, 90, 100, 100, 99, 100, 95
John Khuu, 83, 77, 88, 76, 79, 72, 76
..
<<more if there are more students>>
Sample output file : output.txt (the output format is : name, letter grade, sorted by name) e.g Letter grade for three students given in input.txt file is:
Rock Smith: A
Baker Adam: A
John Khuu: C
..
<<more if there are more students>>
Sample Run of the program (name of the application is letterGrader): Remember there are two sets of outputs. Letter grade is written in the output disk file (which is not shown in the screen), and score averages are displayed on the console (and are not written in the disk file).
Here is an example of run in the command line
C:>java letterGrader input.txt output.txt
Letter grade has been calculated for students listed in input file input.txt and written to output file output.txt
Here is the class averages:
Q1 Q2 Q3 Q4 Mid1 Mid2 Final
Average 82.25 80.38 82.25 83.88 81.38 84.13 78.63
Minimum 62.25 60.38 62.25 63.88 61.38 64.13 68.63
Maximum 99.25 98.38 97.25 96.88 95.38 94.13 98.63
Press ENTER to continue . .
C:>_
An simplified pseudo code for the driver class (letterGrader.java), the application, may look like this:
public class letterGrader { public static void main (String args[]) {
Grader grader = new Grader(args[0], args[1]);
grader.readScore(); // reads score and stores the data in member variables
grader.calcGrade();// determines letter grade and stores information
grader.printGrade();//writes the grade in output file
grader.displayAverages();//displays the averages in console
grader.doCleanup(); //use it to close files and other resources
}
}Step by Step Solution
3.55 Rating (172 Votes )
There are 3 Steps involved in it
Step: 1
TestLetterGraderjava import javaioFile import javaioIOException import javaioPrintWriter import javautilArrayList import javautilScanner class LetterGrader private String inputFileName private String ...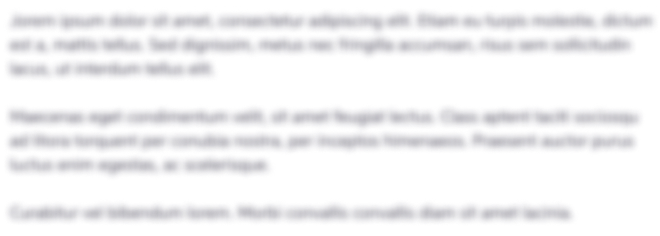
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started