Question
1. A bear is an animal and a zoo contains many animals, including bears. Three classes Animal, Bear, and Zoo are declared to represent animal,
1. A bear is an animal and a zoo contains many animals, including bears. Three classes Animal, Bear, and Zoo are declared to represent animal, bear and zoo objects. Which of the following is the most appropriate set of declarations?
Question 1 options:
public class Animal extends Bear { ... } public class Zoo { private Animal[] myAnimals; ... }
| |
public class Animal extends Zoo { private Bear myBear; ... } | |
public class Bear extends Animal, Zoo { ... } | |
public class Bear extends Animal implements Zoo { ... } | |
public class Bear extends Animal { ... } public class Zoo { private Animal[] myAnimals; ... } |
2. Consider the following static method.
private static void recur(int n) { if (n != 0) { recur (n - 2); System.out.println (n + " " ); } }
What numbers will be printed as a result of the call recur(7)?
Question 2 options:
No numbers will be printed because of infinite recursion | |
7 5 3 1 | |
Many numbers will be printed because of infinite recursion. | |
-1 1 3 5 7 | |
1 3 5 7 |
3. Assume that class Vehicle contains the following method.
public void setPrice(double price) { /* implementation not shown */ }
Also assume that class Car extends Vehicle and contains the following method.
public void setPrice(double price) { /*implementation not shown */}
Assume Vehicle v is initialized as follows.
Vehicle v = new Car(); v.setPrice(1000.0);
Which of the following is true?
Question 3 options:
The code above will cause a compile-time error because of type mismatch. | |
The code above will cause a run-time error, because a subclass cannot have a method with teh same name and the same signature as its superclass. | |
The code above will cause a compile-time error, because a subclass cannot have a method with the same name and the same signature as its superclass. | |
The code v.setPrice(1000.0); will cause the setPrice method of the Car class to be called. | |
The code v.setPrice(1000.0); will cause the setPrice method of the Vehicle class to be called. |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
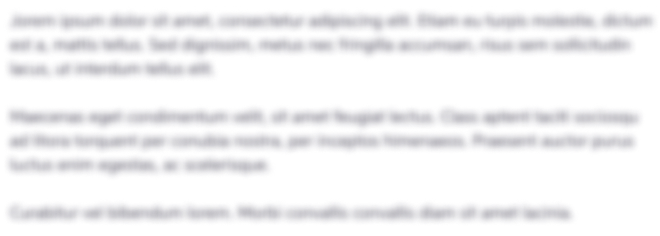
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started