Question
1. a) Complete the Set ADT by implementing intersect() and difference(). b) Modify the Set() constructor to accept an optional variable argument to which a
1.
a) Complete the Set ADT by implementing intersect() and difference().
b) Modify the Set() constructor to accept an optional variable argument to which a collection of initial values can be passed to initialize the set. The prototype for the new constructor should look as follows:
def Set( self, *initElements = None )
It can then be used as shown here to create a set initialized with the given values:
S = Set( 150, 75, 23, 86, 49 )
c) Add a new operation to the set ADT to test for a proper subset. Given two sets, A and B, A is a proper subset of B, if A is a subset of B and A does not equal B.
d) Add the _str() method to the Set implementation to allow a user to print the contents of the set. The resulting string should look similar to that of a list, except you are to use curly braces to surround the elements.
e) Add Python operator methods to the Set class that can be used to perform similar operations to those already defined by named methods:
Operator Method Current Method
__add__(setB) union(setB)
__mul__(setB) interest(setB)
__sub__(setB) difference(setB)
__lt__(setB) isSubsetOf(setB)
# Implementation of the Set ADT container using a Python list.
class Set :
# Creates an empty set instance.
def __init__( self ):
self._theElements = list()
# Returns the number of items in the set.
def __len__( self ):
return len( self._theElements )
# Determines if an element is in the set.
def __contains__( self, element ):
return element in self._theElements
# Adds a new unique element to the set.
def add( self, element ):
if element not in self :
self._theElements.append( element )
# Removes an element from the set.
def remove( self, element ):
assert element in self, "The element must be in the set."
self._theElements.remove( item )
# Determines if two sets are equal.
def __eq__( self, setB ):
if len( self ) != len( setB ) :
return False
else :
return self.isSubsetOf( setB )
# Determines if this set is a subset of setB.
def isSubsetOf( self, setB ):
for element in self :
if element not in setB :
return False
return True
# Creates a new set from the union of this set and setB.
def union( self, setB ):
newSet = Set()
newSet._theElements.extend( self._theElements )
for element in setB :
if element not in self :
newSet._theElements.append( element )
return newSet
# Creates a new set from the intersection: self set and setB.
# def interset( self, setB ):
# ......
# Creates a new set from the difference: self set and setB.
# def difference( self, setB ):
# ......
# Returns an iterator for traversing the list of items.
# def __iter__( self ):
# return _SetIterator( self._theElements )
Step by Step Solution
There are 3 Steps involved in it
Step: 1
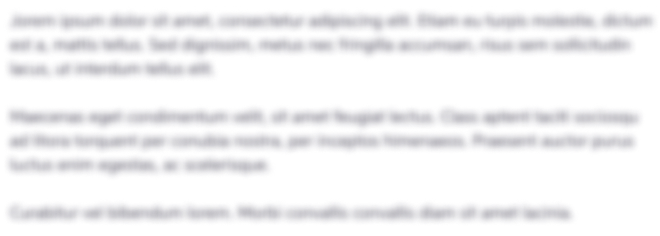
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started