Question
1. Add exception class objects to the Account classes from week 3 assignment, and add try-catch blocks to the code of all member functions where
1. Add exception class objects to the Account classes from week 3 assignment, and add try-catch blocks to the code of all member functions where you think they may be needed.
2. Using exception handling semantics show what exceptions should be thrown from your class above if you try to withdraw more than there is in the account or above the accounts overdraft limit. Add code to throw the exception
3. Catch the exception thrown in 2 and handle it by printing an appropriate message to the screen informing the user of the exceeding the balance availability or overdraft limit.
The following is week 3 assinment
#include
using namespace std;
//Base class class Account { private: //Private instance variables string account_number; string customer_name;
public: //Constructor Account(string accNo, string name) { account_number = accNo; customer_name = name; }
//Destructor ~Account() { cout<<" Base class destructor "; } };
//Derived class class CheckingAccount : public Account { private: //Private instance variables double interestRate; double overDraftLimit; double balance;
public: //Constructor CheckingAccount(string accNo, string name, double bal, double overLimit, double intRate):Account(accNo, name) { interestRate = intRate; overDraftLimit = overLimit; balance = bal; }
//Method that returns balance double getBalance() { return balance; }
//Method that returns interest rate double getInterestRate() { return interestRate; }
//Method that withdraw amount void withdraw(double amt) { //Checking for overdraft limit if((balance - amt) < overDraftLimit) { cout<<" Error!! Amount exceeds the Overdraft limit...."; return; }
balance = balance - amt; }
//Method that deposits amount void deposit(double amt) { if(amt <= 0) { cout<<" Error!! Amount can't be less than or equal to zero "; return; } balance = balance + amt; }
//Method that returns monthly interest double getMonthlyInterest() { return (interestRate/12.0)*balance; } };
//Derived class class SavingsAccount : public Account { private: //Private instance variables double interestRate; double balance;
public: //Constructor SavingsAccount(string accNo, string name, double bal, double intRate):Account(accNo, name) { interestRate = intRate; balance = bal; }
//Method that returns balance double getBalance() { return balance; }
//Method that returns interest rate double getInterestRate() { return interestRate; }
//Method that withdraw amount from account void withdraw(double amt) { if(amt > balance) { cout<<" Error!! Insufficient funds ...."; return; }
balance = balance - amt; }
//Method that deposits amount void deposit(double amt) { if(amt <= 0) { cout<<" Error!! Amount can't be less than or equal to zero "; return; } balance = balance + amt; }
//Method that returns monthly interest rate double getMonthlyInterest() { return interestRate/12.0; } };
//Main function int main() { int ch; double amt;
//Object instances CheckingAccount checkObj("C1234","Anil",100,50,9.2); SavingsAccount saveObj("S8955","Manu",100,8.5);
while(1) { //Display menu cout<<" ******** Menu ********* "; cout<<" 1 - Withdraw \t 2 - Deposit \t 3 - Balance 4 - Quit "; cout<<" Enter your choice: "; cin>>ch;
//User functions switch(ch) { case 1: cout<<" Enter amount to Withdraw: $"; cin >> amt; checkObj.withdraw(amt); break;
case 2: cout<<" Enter amount to Deposit: $"; cin >> amt; checkObj.deposit(amt); break;
case 3: cout<<" Current Balance: $" << checkObj.getBalance(); break;
case 4: exit(0);
default: cout<<" Error!! Invalid Option "; break; } }
cout << " "; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
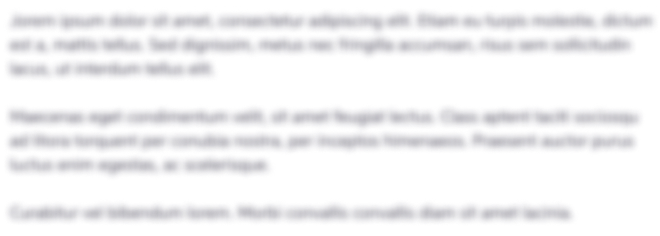
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started