Answered step by step
Verified Expert Solution
Question
1 Approved Answer
1. After looking over the code in Exercise 1, please broadly explain that the code in the driver class does.(please describe what the code is
1. After looking over the code in Exercise 1, please broadly explain that the code in the driver class does.(please describe what the code is doing in these images )
2. After looking over the code in Exercise 2, please broadly explain that the code in the driver class does.(what is this code doing?)
3. Please make linked list box and arrow diagrams to explain how the
addNode(DLLNode newNode) and toString() methods in the DLList.java class work.
4. Explain how this code works using box and arrow diagrams.
public class DLLDriver { public static void main(String[] args) { DLList myList = new DLList("Isaac"); DLLNode friend1 = new DLLNode("Mikey"); DLLNode friend2 = new DLLNode("Julia"); myList.addNode(friend1); myList.addNode(friend2); myList.addNode(new DLLNode("Tim")); myList.addNode(new DLLNode("Jon")); // Testing reverse method 1 System.out.println(myList.toString()); String val = myList.reverseListString(); System.out.println(val); System.out.println(); // Testing reverse method 2 System.out.println(myList.toString()); myList.reverseListv2(); System.out.println(myList.toString()); System.out.println(); } }
//DLList.java
public class DLList { private DLLNode front; private DLLNode rear; public DLList(String name) { this.front = new DLLNode(name); this.rear = front; this.front.setNext(null); this.front.setPrev(null); } public void reverseListv2(){ String firstName = front.getValue(); DLList reversedList = new DLList(firstName); DLLNode frontCursor = new DLLNode(); frontCursor = this.front; this.rear = frontCursor; while (frontCursor.getNext()!= null){ frontCursor = frontCursor.getNext(); String currName = frontCursor.getValue(); DLLNode currNode = new DLLNode(currName); reversedList.addNodeFront(currNode); } this.front = reversedList.front; } private void addNodeFront(DLLNode currNode) { front.setPrev(currNode); currNode.setNext(front); front = currNode; } @Override public String toString() { String toReturn = "front-->"; DLLNode currentF = this.front; while (currentF!=null){ toReturn = toReturn + currentF.nodeString() + "--->"; currentF = currentF.getNext(); } toReturn = toReturn + "back"; return toReturn; } public void addNode(DLLNode node) { if(front==null){ front = new DLLNode(node.getValue()); rear = front; } else{ DLLNode tmp = front; while (tmp.getNext()!=null){ tmp = tmp.getNext(); } node.setPrev(tmp); tmp.setNext(node); } } public String reverseListString(){ String toReturn = ""; toReturn = go(front)+"-->back"; return toReturn; } private String go(DLLNode node) { if(node==null){ return "front"; }else{ return go(node.getNext()) +"--->" + node.nodeString(); } } }
//DLLNode
public class DLLNode { private String value; private DLLNode next; private DLLNode prev; public DLLNode(String value) { this.value = value; this.next = null; this.prev = null; } public DLLNode() { this.value = null; this.next = null; this.next = null; } public DLLNode getPrev() { return prev; } public void setPrev(DLLNode prev) { this.prev = prev; } public String getValue() { return value; } public void setValue(String value) { this.value = value; } public DLLNode getNext() { return next; } public void setNext(DLLNode next) { this.next = next; } public String nodeString() { return "DLLNode{" + "value='" + value + '\'' + ", next=" + next + ", prev=" + prev + '}'; } }} } // CHECK BACK EDGE CASE // Check if currfood, nextfood is null -- if it is, place newNode after currfood. if (currfood.nextfood == null && newNodePlaced == false) { currfood.nextfood = newNode; newNode. nextfood = null; //reset currfood to front of food list .currfood = front2; newNodePlaced = true; current = current.nextname; } } // Task 10: Print out the list in alphabetical FOOD order. current = front2; System.out.println("In FOOD order: " + foodNodesString(front2)); // AND in NAME order current = front; System.out.println("In NAME order: + nameNodesString(front)); } // The code starting at Task 7 was long and repetitive. Not ideal to read // or to work with. While it is POSSIBLE to write code this way (and tempting // when you are starting out), any non-trivial program will soon become // difficult to manage. Look at the second example now. // Can you add a person twice? Is this a good idea? myList.addNode(new PersonFoodNode(new PersonFood ("Isaac", "Mac & Cheese"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); myList.addNode(new PersonFoodNode(new PersonFood ("Eloise", "Croissants"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); // Can a person be added to the front? myList.addNode(new PersonFoodNode(new PersonFood ("Amy","Fish Chews"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); System.out.println("DONE ADDING NAMES!"); System.out.println(); System.out.println(); // Fill in the missing code to make the following commands work properly: // (including the command that is currently commented out) System.out.println("Removing ISAAC"); myList.removeByName("Isaac"); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); } } frontCursor = this. front; while(frontCursor.nextode. != null) { frontCursor = frontCursor.nextnode; String currName = frontCursor.name; DLLNode currNode. = new DLLNode (currName); reversedList.addNode(currNode); this.front = reversedList.front; } public String toString() { String toReturn = "front-->"; DLLNode current F = this. front; while(currentF!= null) { toReturn = toReturn + currentF.nodeString() + ". current = currentF..nextnode; } toReturn = toReturn + "back"; return toReturn; } // COMPLETE THE FOLLOWING METHOD THAT RATHER THAN // REVERSING THE LIST, JUST PRODUCES A STRING THAT // ALLOWS THE LIST TO BE PRINTED IN REVERSE ORDER. public String reverseListString() { String toReturn = ""; // YOUR CODE HERE. return toReturn; } } } // CHECK BACK EDGE CASE // Check if currfood, nextfood is null -- if it is, place newNode after currfood. if (currfood.nextfood == null && newNodePlaced == false) { currfood.nextfood = newNode; newNode. nextfood = null; //reset currfood to front of food list .currfood = front2; newNodePlaced = true; current = current.nextname; } } // Task 10: Print out the list in alphabetical FOOD order. current = front2; System.out.println("In FOOD order: " + foodNodesString(front2)); // AND in NAME order current = front; System.out.println("In NAME order: + nameNodesString(front)); } // The code starting at Task 7 was long and repetitive. Not ideal to read // or to work with. While it is POSSIBLE to write code this way (and tempting // when you are starting out), any non-trivial program will soon become // difficult to manage. Look at the second example now. // Can you add a person twice? Is this a good idea? myList.addNode(new PersonFoodNode(new PersonFood ("Isaac", "Mac & Cheese"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); myList.addNode(new PersonFoodNode(new PersonFood ("Eloise", "Croissants"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); // Can a person be added to the front? myList.addNode(new PersonFoodNode(new PersonFood ("Amy","Fish Chews"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); System.out.println("DONE ADDING NAMES!"); System.out.println(); System.out.println(); // Fill in the missing code to make the following commands work properly: // (including the command that is currently commented out) System.out.println("Removing ISAAC"); myList.removeByName("Isaac"); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); } } frontCursor = this. front; while(frontCursor.nextode. != null) { frontCursor = frontCursor.nextnode; String currName = frontCursor.name; DLLNode currNode. = new DLLNode (currName); reversedList.addNode(currNode); this.front = reversedList.front; } public String toString() { String toReturn = "front-->"; DLLNode current F = this. front; while(currentF!= null) { toReturn = toReturn + currentF.nodeString() + ". current = currentF..nextnode; } toReturn = toReturn + "back"; return toReturn; } // COMPLETE THE FOLLOWING METHOD THAT RATHER THAN // REVERSING THE LIST, JUST PRODUCES A STRING THAT // ALLOWS THE LIST TO BE PRINTED IN REVERSE ORDER. public String reverseListString() { String toReturn = ""; // YOUR CODE HERE. return toReturn; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
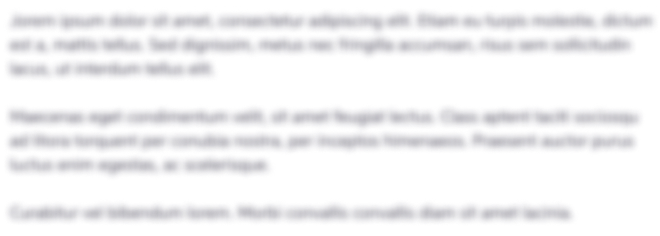
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started