Question
1. Area Class Write a class that has three overloaded static methods for calculating the areas of the following geometric shapes: - circles - rectangles
1. Area Class Write a class that has three overloaded static methods for calculating the areas of the following geometric shapes: - circles - rectangles - cylinders Here are the formulas for calculating the area of the shapes. Area of a circle: Area = r2 where p is Math.PI and r is the circle's radius Area of a rectangle: Area = Width x Length Area of a cylinder: Area = r2 h where p is Math.PI, r is the radius of the cylinder's base, and h is the cylinder's height Because the three methods are to be overloaded, they should each have the same name, but different parameter lists. Demonstrate the class in a complete program.
This is what I have so far
public class area {
public static double Area (double rad){
return Math.PI*rad*rad;
}
public static double Area (double length,double width){
return length*width;
}
public static double Area (double radi,double height){
return Math.PI*rad*rad*height;
}
}
import java.util.Scanner;
public class main{
public static void main(String args[]){
Scanner input = new Scanner();
System.out.println("Enterradiustocalculatecirclearea:");
double rad = input.nextDouble();
System.out.println("Enterwidthtocalculaterectanglearea:");
double width = input.nextDouble();
System.out.println("Enterlengthtocalculaterectanglearea:");
double length = input.nextDouble();
System.out.println("Enterbaseradiustocalculatecylinderarea:");
double radi = input.nextDouble();
System.out.println("Enterheighttocalculatecylinderarea:");
double height = input.nextDouble();
System.out.println("Theareaofthecircleis:"+ area.Area(double radi);
System.out.println("Theareaoftherectangleis:"+ area.Area(double length,double width);
System.out.println("Theareaofthecylinderis:"+ area.Area(double radi,double height);
}
}
This is the error I am getting
Step by Step Solution
There are 3 Steps involved in it
Step: 1
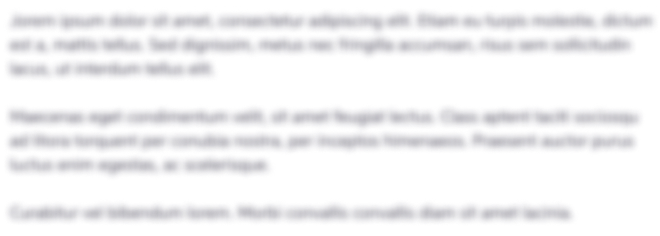
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started