Question
1. At start , your program will first call the chooseWord method that selects a word from a text file and returns the word selected
1. At start, your program will first call the
chooseWord
method that selects a word from a text file and
returns the word selected (i.e., a String is returned from the method). This method is already implemented
in the starter code. You must NOT modify the method but instead will call it from the
main
method or
from a method you create. The
chooseWord
method takes two parameters. When you call the method, use
f
and
rand
(already initialized in the
main
method) as the parameters.
The word selected from the
chooseWord
method should be validated by calling the
isValidWord
method,
which returns,
false
if the word does not satisfy any of the following constraints:
No alphabet occurs three or more times (e.g.,
sadness
is invalid).
At least 6 alphabets in the word (e.g.,
enjoy
is invalid).
Only alphabets in the word (e.g.,
bona fide
is invalid).
The selection-validation process should be repeated until a word satisfying the constraints is selected.
2. After a secret word is selected, your program will display a prompt message for a game mode. The player
will enter either i (or I) for the
case insensitive
mode or s (or S) for the
case sensitive
mode. Then the
player will type the enter key to start to game. The following shows an example interaction with the
player in the console. In the example, the player chooses the case insensitive mode. (We will use this mode
in the running example.) If the player enters any other key (e.g., k), repeat displaying the prompt again
and receive the user input.
Choose the game mode i/I for case insensitive mode, s/S for case sensitive mode): i
You selected case insensitive mode.
3. The program will then display a prompt message to receive a guess from the player. It will display the
selected word in console by a row of underscore characters, where each underscore character represents
each letter of the word (say this
hidden word
). Now, the program is ready to interact with the player. For
example, if the word is
practice
(7 unique alphabets),
________ 10 Remaining guesses
Enter a letter or string:
4. If the guessing player enters a letter or a string, followed by an enter key,
(a) If the letter or string occurs in the word, your program shows it in all its correct positions. In specific,
the program prints one more line that reveals the correct letter(s) or string(s). Set the initial number
of guesses to 3 more than the number of unique alphabets in the selected word. For example, if the
word is
practice
(7 unique alphabets) and the player first enters
ice
, the following message should be
printed:
_____ice 10 Remaining guesses
Enter a letter or string:
In this example, the number of guesses left is unchanged because
ice
occurs in the word. When you
write code that prints the messages, put 3 space characters between the word and the remaining guess
message, and put a space after : in the second line. Note that this example assumes the
case-insensitive mode. Even though the player enters
ICE
, the output message should be identical.
The second line will only be displayed if the word is not fully revealed after the guess.
1
(b) If the suggested letter or string does not occur in the word, the number of allowed guesses will be
decreased by 1. In the example above, if the player first enters
k
, the following message should be
printed:
k does not occur in the word.
________ 9 Remaining guesses
Enter a letter or string:
5. Repeat the step 3 and 4 until all alphabets of the word is fully revealed or until the number of allowed
guesses is decreased to 0.
6. If all guesses are used up and the word is still not fully revealed, display the following message. Again, the
word we use in the example is
practice
.
You lost! (The word was practice)
7. If there are 0 or more remaining guesses and if the word is fully revealed, display a message.
Great! You saved the man!
8. After the player uses up all guesses or the word is revealed, your program asks if the player wants to play
again. If the answer is yes, the game begins again. Otherwise, the game ends. Use the following messages:
Do you want to play again? (y/n):
The player will enter y (or Y) for another game, or n (or N) to end the game. Again, put a space after
: in the message. If the player wants to play another game, repeat the flow from the step 1. If the player
enters any other key (e.g., k), repeat displaying the prompt again and receive the user input.
Invalid command. Do you want to play again? (y/n):
and here's my source code
import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; import java.util.Random; import java.util.Scanner;
public class Hangman {
final static int nWords = 2266;
/** * Do NOT modify this method This method chooses a word from a text file * randomly. * * @param f * @return * @throws FileNotFoundException */ public static String chooseWord(File f, Random r) throws FileNotFoundException { Scanner fileIn = new Scanner(f); try { int randInt = r.nextInt(nWords); int i = 0; while (i != randInt) { fileIn.nextLine(); i++; } return fileIn.nextLine(); } catch (Exception e) { return ""; } finally { fileIn.close(); } }
/** * This method returns a string that contains underscore characters only. The * number of underscore characters must be same to the number of word passed as * the input parameter. * * @param word * the word selected from the chooseWord method * @return */ public static String createDisguisedWord(String word) { throw new UnsupportedOperationException("Remove this line and replace with your implementation."); }
/** * This method returns true if the guess entered by the player occur in the * secret word. Otherwise it return false. * * @param guess * a string that contains one or more characters * @param secretWord * the word selected from the chooseWord method * @return */ public static boolean isValidGuess(String guess, String secretWord) { throw new UnsupportedOperationException("Remove this line and replace with your implementation."); }
/** * This method finds all occurrences of guess in secretWord and returns new * disguisedWord with letters revealed after guess * * @param guess * @param secretWord * @param disguisedWord * @return */ public static String makeGuess(String guess, String secretWord, String disguisedWord) { throw new UnsupportedOperationException("Remove this line and replace with your implementation."); }
/** * This method returns true if the (partially) revealed word (disguisedWord) is * identical to the secret word. Otherwise it returns false. If all letters in * the secret word are revealed, those two should be identical. * * @param secretWord * @param disguisedWord * @return */ public static boolean isWordRevealed(String secretWord, String disguisedWord) { throw new UnsupportedOperationException("Remove this line and replace with your implementation."); }
/** * This method computes and returns an integer of which value is 3 greater than * the number of unique letters in the secret word. * * @param word * the word selected from the chooseWord method * @return */ public static int initGuesses(String word) { throw new UnsupportedOperationException("Remove this line and replace with your implementation."); }
/** * This method returns true if the word selected from the word list satisfies * the constraints specified in the project document. Otherwise it returns * false. * * @param word * the word selected from the chooseWord method * @return */ public static boolean isValidWord(String word) { throw new UnsupportedOperationException("Remove this line and replace with your implementation."); }
public static void main(String[] args) throws IOException { File f = new File("listOfWords.txt"); Random rand = new Random(2018); Scanner reader = new Scanner(System.in); // Do NOT modify the lines above in this method
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
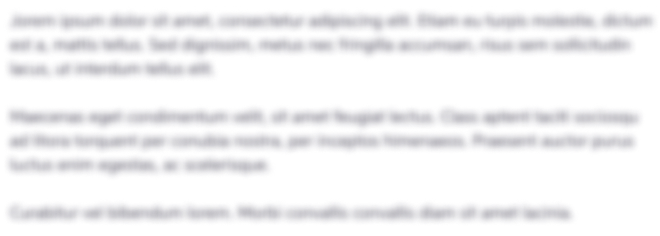
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started