Question
1. CargoStrength Enum Write a simple Enum named CargoStrength, which lists the strength values a cargo container may have. If you are unfamiliar with Java
1. CargoStrength Enum
Write a simple Enum named CargoStrength, which lists the strength values a cargo container may have. If you are unfamiliar with Java Enum types, you can read about them in this Java tutorial. Java Enums are very similar to Java classes, though they are typically used to encapsulate a set of related constants. In this case, we use it to represent the strength of the cargo, which can be either FRAGILE, MODERATE, or STURDY.
The rules for the CargoStrength type is described as follows:
FRAGLIE containers can only support other FRAGILE containers.
MODERATE containers can support other MODERATE containers, as well as FRAGILE containers.
STURDY containers can support all other containers.
The number of containers above any given containers does not matter. The only restrictions are the three rules above (Though this is a naive assumption, the simplification will make the assignment easier).
2. Cargo Class
Write a fully documented class named Cargo. This class represents a container which holds the cargo the program will place on the ship. It contains the following private member variables: name (String), weight (double) and strength (CargoStrength).
The Cargo class itself is actually immutable. This means once it has been constructed, no data within can be changed. From the UML diagram, note that the public constructor takes all the member variables as arguments. Data can still be read via the getter methods.
private String name
private double weight
private CargoStrength strength
public Cargo(String initName, double initWeight, CargoStrength initStrength)
Brief:
Default Constructor.
Parameters: initName
Non-null name for the cargo item.
initWeight
The weight for the cargo. The weight should be greater than 0, so an exception should be thrown if initWeight 0 (We do not deal with massless cargo).
initStrength
Either FRAGILE, MODERATE, or STURDY.
Preconditions:
initName is not null.
initWeight > 0.
Postconditions:
This object has been initialized to a Cargo object with the given name, weight, and strength.
Throws: IllegalArgumentException
If initName is null.
If initWeight 0.
Getters ONLY for name, weight, strength
3. CargoStack class (or java.util.Stack)
Since Cargo objects can be physically stacked on top of one another, we can store our Cargo objects within a Stack ADT. In this assignment, you can write your own Stack class or you may use the standard Stack class that is in the java.util package. Go to the HELP section and view the online documentation for Stack from the Java API.
CAUTION: Although the Stack class has push and pop methods, the Stack class is a subclass of Vector. Therefore, all of the methods of Vector are also accessible in the Stack class. However, if you use any of the inherited Vector methods in your solution, you will be penalized in this assignment, since some of the Vector methods are not supposed to apply to a Stack ADT in general. (That is, the designers of Java basically define that a Stack is a special type of Vector, but it really isn't.)
4. CargoShip class
Write a fully documented class named CargoShip. This class represents a container ship which holds stacks of containers. It must contain the following private member variables: stacks (CargoStack[]), maxHeight (int), and maxWeight (double). The CargoShip class must also feature the following public methods: void pushCargo(Cargo cargo, int stack), Cargo popCargo(int stack), and void findAndPrint(String name).
private CargoStack[] (or java.util.Stack[]) stacks
Array of container stacks on the ship. The array should be initialized in the constructor to the size indicated by the parameter numStacks.
private int maxHeight
private double maxWeight
public CargoShip(int numStacks, initMaxHeight, initMaxWeight)
Brief:
Default Constructor.
Parameters: numStacks
The number of stacks this ship can support. This parameter should be used to initialize the stacks array to a fixed size.
initMaxHeight
The maximum height of any stack on this ship.
initMaxWeight
The maximum weight for all the cargo on the ship.
Preconditions:
numStacks > 0.
initMaxHeight > 0.
initMaxWeight > 0.
Postconditions:
This object has been initialized to a CargoShip object with the indicated number of stacks, maxHeight, and maxWeight.
Throws: IllegalArgumentException
if either of the parameters are now within the appropriate bounds.
public void pushCargo(Cargo cargo, int stack) throws FullStackException, ShipOverweightException, CargoStrengthException
Brief:
Pushes a cargo container to the indicated stack on the cargo ship
Parameters: cargo
The container to place on the stack.
stack
The index of the stack on the ship to push cargo onto.
Note: you may choose to do your actual indexing starting at 0, but from the user's perspective, the leftmost stack must be number 1.
Preconditions:
This CargoShip is initialized and not null.
cargo is initialized and not null.
1 stack stacks.length.
The size of the stack at the desired index is less than maxHeight.
The total weight of all Cargo on the ship and cargo.getWeight()is less than maxWeight.
Postconditions:
The cargo has been successfully pushed to the given stack, or the appropriate exception has been thrown, in which case the state of the cargo ship should remain unchanged.
Throws: IllegalArgumentException
If cargo is null or stack is not in the appropriate bounds.
FullStackException
If the stack being pushed to is at the max height.
ShipOverweightException
If cargo would make the ship exceed maxWeight and sink. (imaginary bonus points for "yellow submarine" refrences in the exception message).
CargoStrengthException
If the cargo would be stacked on top of a weaker cargo (see the CargoStrength rules above for reference).
public Cargo popCargo(int stack) throws EmptyStackException
Brief:
Pops a cargo from one of the specified stack.
Parameters: stack
The index of the stack to remove the cargo from.
Note: Again, from the user's perspective, the leftmost stack must be indexed 1.
Preconditions:
This CargoShip is initialized and not null.
1 stack stacks.length.
Postconditions:
The cargo has been successfully been popped from the stack, and returned, or the appropriate exception has been thrown, in which case the state of the cargo ship should remain unchanged.
Throws: IllegalArgumentException
If stack is not in the appropriate bounds.
EmptyStackException
If the stack being popped from is empty.
public void findAndPrint(String name)
Brief:
Finds and prints a formatted table of all the cargo on the ship with a given name. If the item could not be found in the stacks, notify the user accordingly. (see sample I/O for an example).
Parameters:
name:The name of the cargo to find and print.
Preconditions:
This CargoShip is initialized and not null.
Postconditions:
The details of the cargo with the given name have been found and printed, or the user has been notified that no such cargo has been found.
The state of the cargo ship should remain unchanged.
5. ShipLoader class
Write a fully-documented class named ShipLoader which allows a user to create an instance of the CargoShip class, and also provides an interface for a user to manipulate the ship by creating, adding, and moving Cargo objects. In addition to the CargoShip, the ShipLoader utilizes another CargoStack called dock, which is the loading/unloading stack for Cargo being moved to/from the ship. The following functionality should be provided:
public static void main(String[] args)
Brief:
The main method runs a menu driven application which allows the user to create an instance of the CargoShip class and then prompts the user for a menu command selecting the operation. The required information is then requested from the user based on the selected operation. Following is the list of menu options and their required information:
A sample menu is provided below indicating the functionality your ShipLoader program should support:
C) Create new cargoL) Load cargo from dock U) Unload cargo from ship M) Move cargo between stacks K) Clear dock P) Print ship stacks S) Search for cargo Q) Quit
A few notes for the above operations:
When cargo is created, it is initially placed on the dock to be moved to the ship. Note that this dock is just another instance of the CargoStack and is thus subject to the same CargoStrength rules listed above (i.e. a STURDY Cargo cannot be created if a FRAGILE Cargo is currently at the top of the dock stack). However, the dock is not limited to the maxHeight limitation of the CargoShip. The dock can stack as many items as necessary, as long as the CargoStrength rules are not violated. Please see the sample I/O for an example.
Any time cargo is created or moved between stacks, the program should automatically print the ship stacks immediately after performing the reqested operation.
For option S), the program should only search the stacks on the ship (the dock should not be included in the search).
6. Exception classes
You should create whatever relevant exception classes are required to express invalid operations performed by the user. Below are a list of the Exception classes which you should use for the assignment:
FullStackException
Should be thrown if the user attempts to push a Cargo object onto a stack which is currently at the maximum height.
EmptyStackException
Should be thrown if the user attempts to pop a CargoStack that currently has no Cargo items on it.
ShipOverweightException
Should be thrown if the user attempts to push a Cargo object on to any stack of the CargoShip which would put it over it's maximum weight limit.
CargoStrengthException
Should be thrown if the user attempts to push a Cargo object onto another Cargo object that violates the CargoStrength rules indicated above.
Note: You should make sure that you catch ALL exceptions that you throw anywhere in your code. Exceptions are used to indicate illegal or unsupported operations so that your program can handle unexpected events gracefully and prevent a crash. Your program should NOT crash from any of the above exceptions (it should not crash from any exception, but especially not one that you throw yourself).
Extra Credit:
For extra credit, you can implement an optional menu function, R) Remove
For this operation, you can make the following assumptions:
The ship will have 3 stacks and a max height of 3, for a potential maximum of 9 cargo objects on the ship.
All instances of the cargo with the indicated name will have the same CargoStrength (i.e. they can all be stacked on top of one another).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
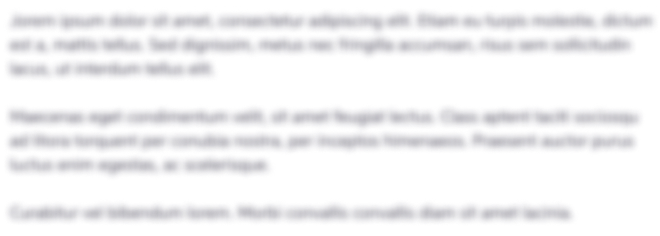
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started