Question
1. Complete assignment P-7.60 on page 305 of the textbook that implements the CardHand class with the additional requirements as listed . When arranging your
1. Complete assignment P-7.60 on page 305 of the textbook that implements the CardHand class with the additional requirements as listed. When arranging your cards:
a. you should not worry about the relative values of the suites (i.e. does a Heart come before a Diamond)
b. Rather you should arrange the suits so that the first suit you are dealt is to the left (first) and additional suits are added to the right (later) as they are received
c. Implement your CardHand class so that adding a new card can be done in constant time, O( 1 ).
d. You must use a LinkedPositionalList to represent the card hand.
e. You must use the four fingers method suggested in the textbook.
2. Implement a Card class that represents a playing card.
a. A card has a suit ( Club, Diamond, Heart, or Spade )
b. A card has a value ( 2 through 10, Jack, Queen, King, or Ace) with 2 being the lowest value and Ace being the highest value.
c. You do not need to support Jokers
3. Implement a Deck class that represents a deck of cards. This Deck class must include the following methods:
a. Deck( ): the constructor that creates a deck of 52 cards, one of each possible suit-value combination.
b. card( ): deals a random card from the deck, where: 1) cards are dealt on a non-replacement basis (i.e. once a card is dealt it cannot be dealt again), and 2) on each deal all cards (remaining) in the deck have an equal probability of being dealt.
c. Selecting the correct data structure should make this easy to implement.
4. Implement a Game class that represents a card game. This Game class must include:
a. An instance variable that represents the number of players in the game (should be passed in as a parameter).
b. An instance variable that represents the maximum number of cards that can be in each players hand (should be passed in as a parameter).
c. An instance variable for the deck of cards used in the game.
d. An instance variable that is an array of the players card hands.
e. A method getCard() that deals one card to each player in the array of players (item c). When a player gets a new card they should immediately add this card to their hand so that they are ordered correctly by suit and value (item 1).
f. Note that the player must immediately insert each new card into the correct position in their hand. The player cannot wait until they have received all of their cards and then sort the cards.
5. A client that tests your classes by dealing a card game. Your client should:
a. Create a new instance of a game with four ( 4 ) players, and 13 cards per player (e.g. like a game of Bridge).
b. Have cards dealt to each player one card at a time and display the hands of each player after each round is dealt. You output should look something like the following (Keep in mind that the order of the suits in each players hand is determined by the order the suits are received):
Card 01:
Player 1: 2C
Player 2: KH
Player 3: 8H
Player 4: 2D
Card 02:
Player 1: 2C 7D
Player 2: KH 9S
Player 3: 3H 8H
Player 4: 9D 2D
And so on until
Card 13:
Player 1: 7C QC 10C 9C 5C KC 2C 5D 7D 6D KS AH 4H
Player 2: 3H QH JH KH 4S 9S 7S JS 4D 8D 4D 3D 10C
Player 3: 5H 8H 6H 7H 10H 8C 3C AD KD QS 6S 5S 3S
Player 4: JD QD 2D 9D AS 8S 10S 2S 9H 2H 6C JC AC
The above list of methods represents the methods that must be present. You will most likely need additional methods in each of the classes to complete the assignment.
--> THIS IS WHAT WAS GIVEN IN P-7.60 ON PAGE 305
P-7.60 Implement a CardHand class that supports a person arranging a group of cards in his or her hand. The simulator should represent the sequence of cards using a single positional list ADT so that cards of the same suit are kept together. Implement this strategy by means of four fingers into the hand, one for each of the suits of hearts, clubs, spades, and diamonds, so that adding a new card to the persons hand or playing a correct card from the hand can be done in constant time. The class should support the following methods:
addCard(r, s): Add a new card with rank r and suit s to the hand.
play(s): Remove and return a card of suit s from the players hand; if there is no card of suit s, then remove and return an arbitrary card from the hand.
iterator( ): Return an iterator for all cards currently in the hand.
suitIterator(s): Return an iterator for all cards of suit s that are currently in the hand.
--> PLUS THERE IS AN ADDITIONAL INFORMATION GIVEN BY OUR INSTRUCTOR.
Based on the description of the Card class in the assignment it may make more sense to define the addCard methods as:
addCard( Card newCard)
instead of the
addCard( rank, suit ) described in the text.
You may choose which method you wish to implement.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
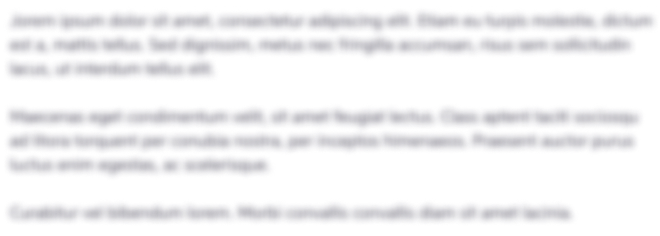
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started