Question
1- Create a checked exception called, FacebookException, and make it extend java.lang.Exception. Override all the inherited constructors from java.lang.Exception. public class String FacebookException extends Exception
1- Create a checked exception called, FacebookException, and make it extend
java.lang.Exception. Override all the inherited constructors from java.lang.Exception.
public class String FacebookException extends Exception
// override all constructors in the Exception class
2- Change the UserAccount class that you created in assignment one to be an abstract class
And define the below abstract method:
/**
* Displays the password help text for this user to the console.
* @return returns the password help text.
*/
public abstract String getPasswordHelp();
Create a subclass of UserAccount called FacebookUser. Define the following fields and methods
On the FacebookUser class:
Fields
private String passwordHint;
private List
Methods
/**
* Applies the supplied password hint text to this user.
*
* @param hintText the text.
*/
public void setPasswordHint(String hintText)
/**
* Adds the supplied friend to the list of friends for this user.
*
* @param friend the friend to add.
* @throws FacebookException thrown if the supplied user
* is already a friend to this user.
*/
public void addFriend(FacebookUser friend) throws FacebookException
// This method should add the FacebookUser argument to the friends List.
// If the supplied friend already exists in the friends List, then throw
// a new FacebookException with the message,
// You are already friends with this user.
/**
* Removes the supplied friend from the list of friends for this user.
* @param friend the friend to remove.
* @throws FacebookException thrown if the supplied user
* is not a friend of this user.
*/
public void removeFriend(FacebookUser friend) throws FacebookException
// Removes the supplied friend from the friends List.
// If the supplied user is not a friend of this user, then throw
// a new FacebookException with the message,
// You are NOT friends with this user.
/**
* Returns a list of Facebook users that are friends with this user.
* @return the list or empty list if this user has no friends.
*/
public List
// return a copy of this users friends list create a new List
// with the same FacebookUsers in it as this users friends.
// Do not return the friends List directly, since this violates
// the principle of encapsulation.
3 - Make FacebookUser class implement the Comparable interface. Ensure that the natural order for the FacebookUser is by username, ignoring capitalization.
Use the getUsername() method to compare two Facebook users.
4 - Create a class called, FacebookUserTest that contains JUnit test methods (annotated with @Test), to test the following:
Add, retrieve, and delete friends
o Create a unit test that creates three facebook users. Assign the first username as han, the second username as luke, and the last username as leia
o Assign luke and leia as friends of han
o Assert that han has two friends and that they are luke and leia
o Remove leia as hans friend o Assert that han has one friend that that it is luke
Help text
o Create a unit test which creates a facebook user. Call the setPasswordHint method, passing enter a valid password
o Assert that the getPasswordHelp method returns the text, enter a valid password
Error check adding a friend
o Create a unit test which creates two facebook users. Assign the first username as han and the second username as luke
o Assign luke as hans friend
o Assign luke again as hans friend
o Assert that the addFriend method threw a FacebookException with the message, You are already friends with this user on the second assignment of luke to han.
Error check removing a friend
o Create a unit test which creates three facebook users. Assign the first username as han, the second username as luke, and the last username as leia
o Assign luke as a friend of of han
o Call the removeFriend method, passing leia, and assert that the method throws a FacebookException with the message, You are NOT friends with this user
Sorting
o Create a unit test which creates three facebook users. Assign the first username as Han, the second username as LUKE, and the last username as leia (make lukes user name uppercase and leias lowercase)
o Assign LUKE and leia as Hans friends. Be sure to assign luke first and leia second to test the sort functionality
o Call the java.util.Collections.sort method, passing hans friends List to the sort method o Assert that the friends list is sorted alphabetically by user name First element should be leia and the second element should be
Step by Step Solution
There are 3 Steps involved in it
Step: 1
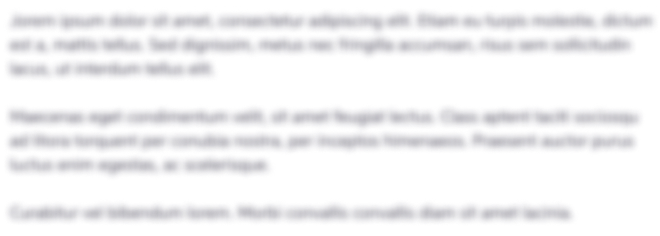
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started