Question
1. Create a folder named Assignment 3. Copy your Die and Histogram files into the folder. Make all changes to these new files, leaving the
1. Create a folder named Assignment 3. Copy your Die and Histogram files into the folder. Make all changes to these new files, leaving the originals intact. If you were unable to finish A1 or A2 successfully, you have permission use another students code for Assignment 1 or 2, provided proper documentation giving credit to the original programmer is included. 2. Within the same folder, create a Java file for a Histogram user interface object, named appropriately. (Examples: HistogramUserInterface, HistogramUI, HUI, etc) Also, create a tester-file for the object. 3. This program implements three different commands for gathering data. The first is manual entry. Devise a system where the user can simply enter integer numbers. The exact details on how to enter the numbers are up to you. They may be read in individually or all at once. You may request how many numbers are to be entered. 4. The second command for gathering data is from a file. Implement the command to read values from a filename entered by the user. See the file IO example below for how to read and parse integer data from a file. 5. The third command for gathering data is generated randomly using the Die object previously created. Implement the code for users to enter multiple dice of different sizes to generate the data. The dice do not need to be stored as attributes in the object, just the resulting data. Allow the user to enter up to five different dice to be added together to generate the data. 6. The resulting histogram data and the report can be saved into files. Implement both of these commands, allowing the user to enter a filename. See the file IO example below for how to write to a file. 7. Use the UI file to build a user interface around the Histogram and Die object. Use the tester file to instantiate and test the Histogram user interface. It should accept the following commands: E Enter data manually F File input for raw data G Generate data randomly with Dice D Display current data N Enter name for histogram C Enter comment for histogram H Create a histogram based on currently stored data S Save histogram data in external file R Save histogram report in external file Q Quit program ? Display help menu Anything else Display error message and get new command 8. Use the completed Histogram user interface to demonstrate three different histograms, one for each method of data entry. Needs to look like this Test Run Welcome to Deans Nifty-keen Histogram Program What is your name? => Gern Blanston Hello, Gern Blanston. How can I help you? Here is a list of potential commands: E - Enter raw data manually F - File input for raw data G - Generate data randomly with dice H - create Histogram based on data, name, and comment N - enter a Name for current Histogram C - enter a Comment for current Histogram D - display current Data S - Save histogram data in file R - save histogram Report in file Q - Quit program ? - Display help menu Enter command => N Enter name for histogram => Rising and falling pattern Name set to "Rising and falling pattern" Enter command => C Enter comment => Manually entered data, provided by Zeller Comment set to "Manually entered data, provided by Zeller" Enter command => E Entering data for histogram manually. Erasing current data. Enter numbers, with a blank for the last number. data #0 => 1 data #1 => 2 data #2 => 3 data #3 => 4 data #4 => 5 data #5 => 4 data #6 => 3 data #7 => 2 data #8 => 1 data #9 => 2 data #10 => 3 data #11 => 4 data #12 => 5 data #13 => 4 data #14 => 3 data #15 => 2 data #16 => 1 data #17 => 2 data #18 => 3 data #19 => 4 data #20 => 20 numbers entered. Enter command => D Displaying current data: 1 2 3 4 5 4 3 2 1 2 3 4 5 4 3 2 1 2 3 4 Enter Command => H Summary Report: Rising and Falling Pattern N = 20 min = 1 max = 5 sum = 48 avg = 2.9 1 | X X X 2 | X X X X X 3 | X X X X X 4 | X X X X X 5 | X X --------------- 1 2 3 4 5 Number of Occurrences Manually entered data, provided by Zeller Enter command => S Saving histogram data to file Enter filename => histo1data.txt Saved histogram data to histo1data.txt Enter command => R Saving histogram report to file Enter filename => histo1report.txt Saved histogram report to histo1report.txt Enter command => F File input for data Erasing current data Name of file => racecar.txt Reading data from racecar.txt Read in 127 numbers Enter command => N Entering name for histogram => Racecar Driver Injuries Name set to "Racecar Driver Injuries" Enter command => C Enter comment => Number of injuries for professional racecar drivers Comment set to "Number of injuries for professional racecar drivers" Enter command => H Summary Report: Racecar Driver Injuries N = 127 min = 1 max = 10 sum = 611 avg = 4.811 1 | X X X X X X X X X X X X X X 2 | X X X X X X X X X X X X 3 | X X X X X X X X X X X X X X X X X X X X X 4 | X X X X X X X 5 | X X X X X X X X X X X X X X X X X 6 | X X X X X X X X X X X 7 | X X X X X X X X X X X X X X X X X X X 8 | X X X X X X X X X X X X X X 9 | X X X X 10 | X X X X X X X X ------------------------------------------------- 1 1 1 1 1 1 1 1 1 1 2 2 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1 Number of Occurrences Number of injuries for professional racecar drivers Enter command => S Saving histogram data to file Enter filename => histo2data.txt Saved histogram data to histo2data.txt Enter command => R Saving report to file Enter filename => histo2report.txt Saved histogram report to histo2report.txt Enter command => G Generating data with dice. Erasing old data. How many dice for each roll => 2 Number of sides for die #1 => 6 Number of sides for die #2 => 6 Number of rolls? => 100 Result: 9 5 8 10 6 3 7 11 8 10 11 10 9 5 8 10 7 7 8 8 8 7 10 6 9 9 5 4 6 4 6 3 10 6 7 6 8 7 8 7 10 6 8 8 11 7 9 10 10 9 12 8 5 5 8 7 5 8 8 7 10 9 5 4 6 4 12 6 7 4 8 4 10 7 4 9 2 12 10 12 3 7 9 10 8 4 8 7 11 10 9 10 8 8 10 10 6 6 5 8 Saving data Enter command => N Enter name for histogram => 2D6 100 times Name set to "2D6 100 times" Enter command => C Enter comment => Two six-sided dice are rolled 100 times Comment set to "Two six-sided dice are rolled 100 times" Enter command => H Summary Report: 2D6 100 times N = 100 min = 2 max = 12 sum = 759 avg = 7.59 2 | X 3 | X X X 4 | X X X X X X X X 5 | X X X X X X X X 6 | X X X X X X X X X X X 7 | X X X X X X X X X X X X X X 8 | X X X X X X X X X X X X X X X X X X X X 9 | X X X X X X X X X X 10 | X X X X X X X X X X X X X X X X X 11 | X X X X 12 | X X X X ---------------------------------------------- 1 1 1 1 1 1 1 1 1 1 2 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 Number of Occurrences Two six-sided dice are rolled 100 times Enter command => S Saving histogram data to file Enter filename => histo3data.txt Saved histogram data to histo3data.txt Enter command => R Saving report to file Enter filename => histo3report.txt Saved histogram report to histo3report.txt Enter command => Q Exiting program. Goodbye! The i/o example IOTools.Java Demonstrating File IO import java.io.IOException; import java.nio.charset.Charset; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.io.PrintWriter; import java.util.*; public class IOTools { // Read a comma deliminated txt file into a string // and return that string to the user. If the file // does not exist the IOException will be caught and // printed to the user. Create the file beforehand // in the current working directory. public String ReadIn(String file) { try { String str = String.join(" ",Files.readAllLines(Paths.get(file))); return str; } catch(IOException e) { System.out.println(e); return e.getMessage(); } } // Split that string into a list and then copy that list // into an arrayList and return it to user public ArrayList ToArray(String s) { ArrayList data = new ArrayList(); List rawData = Arrays.asList(s.split(",")); for(String str : rawData) { data.add(Integer.valueOf(str)); } return data; } // Write the given ArrayList of data to the given file // This does not append data, it only writes over it. // A clever user would pull the file in as data, append that // data to a new list and rewrite all of the data to the file public void WriteData(ArrayList data, String file) { try { PrintWriter writer = new PrintWriter(file, "UTF-8"); for(Integer n : data) { writer.print(n+","); } writer.close(); } catch (IOException e) { System.out.println(e); } } } IOTester.Java Testing the IOTools object import java.util.*; public class IOTester { public static void main(String[] args) { IOTools test = new IOTools(); String strData = test.ReadIn("testInput.txt"); ArrayList data = test.ToArray(strData); data.add(99); data.add(201); test.WriteData(data, "testOutput.txt"); } } TestInput.txt initial file for testing 1,2,3,4,5,6,7,8,9,10,111, Already have die and histogram object just need help with creating commands.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
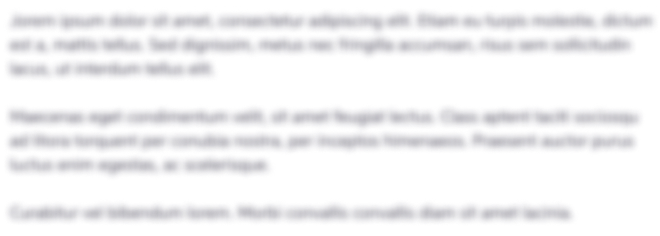
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started