Question
1) Create the program to let the user practice their multiplication tables. Print a multiplication question using two random integers between 1 and 12 as
1) Create the program to let the user practice their multiplication tables. Print a multiplication question using two random integers between 1 and 12 as shown in the sample run. Then allow the user input an int as their answer to the multiplication of the two numbers. If they enter the correct product print "Correct!" otherwise print "Wrong". It must use an else statement in the code.
Sample run 1:
4 * 7 = ? 27 Wrong
Sample run 2:
8 * 2 = ? 16 Correct!
My code is:
public static void main(String[] args){ Scanner scan = new Scanner(System.in); double x = Math.random(); int range = 12; int min = 1; int y = (int)(x * 12) + 1; System.out.print(y); double z = Math.random(); int b = (int)(z * 12) + 1; System.out.print(b); int a = (b * y); int ab = scan.nextInt(); if (a == ab) { System.out.println ("Correct!"); } else System.out.println("Wrong"); }
}
Can someone help me to fix code to perform correctly, based on my mistake that I make:
Make sure have called the Math.random method and used the correct formula to generate random numbers that are between 1 and 12.
Make sure have called the Math.random method and used the correct formula to generate random numbers that are between 1 and 12.
Make sure program prints a multiplication question as shown in the sample run.
Short Circuit Evaluation:
2) Debug the code provided in the starter file. This program is supposed to print "Ratio OK" if the ratio of two integers entered by the user is between 4 inclusive and 6 exclusive (i.e. if the second divided by the first is less than 6 and greater than or equal to 4). The debugged code should not produce any error messages as long as two integers are entered by the user.
public static void main(String[] args){
Scanner scan = new Scanner(System.in);
System.out.println("Enter 2 integers");
int x = scan.nextInt();
int y = scan.nextInt();
if(x >= 4 && y < 6 || x/y != 0) {
System.out.println("Ratio OK");
}
}
}
I fixed a bunch of them but need someone help me find out the main mistake called: "Make sure the corrected boolean condition will be true if the value of the ratio y/x is between 4 and 6."
3) Debug the code in the starter file so it functions as intended and does not cause any errors. This program is intended to take two integer inputs and determine whether the second is a multiple of the first or not. The numberbis a multiple of the numberaifbcan be divided byawith no remainder. It must though that no number can be divided by 0 - so no numbers should be considered a multiple of 0 for the purpose
public static void main(String[] args){
Scanner scan = new Scanner(System.in);
System.out.println("Enter two numbers");
int a = scan.nextInt();
int b = scan.nextInt();
if(b % a != 0 || a == 0)
System.out.println(b + " is not a multiple of " + a);
else {
System.out.println(b + " is a multiple of " + a);
}
}
}
For some reasons, this code still throw some errors when compile, can someone help me fix it?
De Morgan's Law:
For this problem, I don't actually how the law actually work in this case, is it similar to the short circuit one? How it is different?
4) The template code provided is intended to check whether an integer entered by the user is outside of the range 20-29 (inclusive). If it is outside of this range the program should print a warning and change the number to 25. However, when using De Morgan's law to simplify this code, the programmer has made some mistakes. Can it correct the errors so the code functions as intended?
Sample runs of the program functioning properly are below:
Sample run 1:
Enter a number in the twenties 28 Your number is 28
Sample run 2
Enter a number in the twenties 33 That's not in the twenties! Your number is 25
public static void main(String[] args){
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number in the twenties");
int num = scan.nextInt();
if(num >= 20 && num <= 30)
{
System.out.println("Your number is " + num);
}
else if(num < 20 || num > 30){
System.out.println("That's not in the twenties!");
}
}
}
5) The template code provided is intended to take two inputs, x and y, from the user and print "pass" if one or more of the following is true:
- x is not less than 4
- y is not greater than 5 and x + y is less than 7
However, when using De Morgan's law to simplify this code, the programmer has made some mistakes. Can you correct the errors so the code functions as intended?
public static void main(String[] args){
Scanner scan = new Scanner(System.in);
int x = scan.nextInt();
int y = scan.nextInt();
if((x < 4 || y > 5) && x + y > 7) {
System.out.println("pass");
}
}
}
I cannot find my main mistake when debug them when I have something: "Make sure your program prints 'pass' for any values of x and y which meet the conditions in the description." and " Make sure your program prints 'pass' for any values of x and y which meet the conditions in the description."
6) Write the code which prints "Access granted!" if the correct password, "swordfish" is entered, and "Access denied!" otherwise.
Sample runs:
Enter password: marlin Access denied! Enter password: swordfish Access granted!
7) Write the program that asks the user to enter two words, then prints "Great!" if the two words are identical, "Close enough" if they are the same length and all but the last letter matches, and "Try again" otherwise.
Sample runs:
Enter 2 strings: the the Great! Enter 2 strings: that than Close enough Enter 2 strings: their there Try again
Hint - for the "Close enough" condition then it will need to see whether substrings of the two input String are the same. It can use the length and substring methods together to get the relevant substrings (all but the last character of each String).
8) Write code which takes inputs and creates two Rectangle objects (using the edhesive.shapes.Rectangle class) and compares them using the equals method.
The first Rectangle should use a single user input and the single-parameter constructor, making a square Rectangle object. The second Rectangle should should use two user inputs and the second rectangle constructor. The code should then check the two objects for equality, printing "Congruent Rectangles" if they are identical according to the Rectangle equals method and "Different Rectangles" if they are not the same.
Sample run 1
Enter length: 12.6 Enter 2 lengths: 12.6 12.6 Congruent Rectangles
Sample run 2
Enter length: 18.1 Enter 2 lengths: 11.6 18.1 Different Rectangles
To reference the documentation for the Rectangle class:
https://amooc.github.io/edhesiveshapes/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
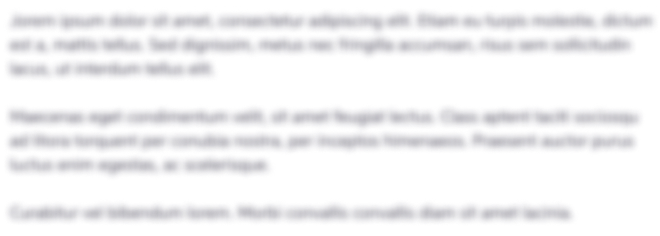
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started