Question
1. Define a class Circle , which is-a Shape and contains following unique data/methods: Data: radius (double); Two constructors: public Circle(double radius) public Circle (double
1. Define a class Circle, which is-a Shape and contains following unique data/methods:
Data: radius (double);
Two constructors:
public Circle(double radius)
public Circle (double radius, String color, boolean filled)
Getter and Setter methods for radius
Methods to calculate area and perimeter
getArea()
getPerimeter()
toString() that returns "(Filled/Unfilled) circle with color = ?, radius = ?, area = ?, perimeter = ?". (You should override the Shape's toString() method; call getArea() and getPerimeter() methods to get area and perimeter )
2. Define a driver class (with main method) called TestCircle to test the class Circle. You are asked to create two circle objects: one is with radius 1.0; the other one is a red filled circle with radius 3.0. Print out both circles information.
3. Define another class Rectangle, which is-a Shape and contains following unique data/methods:
Data: length (double), width (double);
Two constructors:
public Rectangle(double length, double width)
public Rectangle(double length, double width, String color, boolean filled)
Getter and Setter methods for length and width
Methods to calculate area and perimeter
getArea()
getPerimeter()
toString() that returns "(Filled/Unfilled) Rectangle with color = ?, length = ?, width = ?, area = ?, perimeter = ?". (You should override the Shape's toString() method; call getArea() and getPerimeter() methods to get area and perimeter )
4. Define a driver class (with main method) called TestRectangle to test the class Rectangle. You are asked to create two rectangle objects: one is with width 1.0 and length 1.0; the other one is a blue unfilled rectangle with width 3.0 and length 5.0. Print out both rectangles information.
*****
public class Shape {
private String color;
private boolean filled;
public Shape()
{
color = "black";
filled = true;
}
public Shape (String color, boolean filled)
{
this.color = color;
this.filled = filled;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public boolean isFilled() {
return filled;
}
public void setFilled(boolean filled) {
this.filled = filled;
}
@Override
public String toString() {
return "Shape [color=" + color + ", filled=" + filled + "]";
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
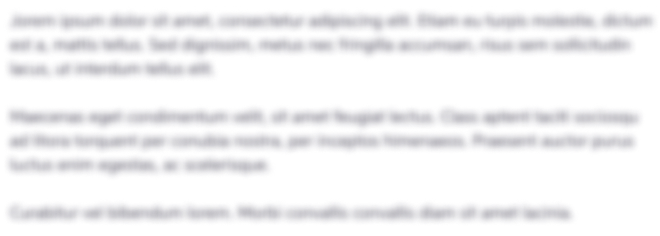
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started