Question
1. (Extra) Write and test a program that instantiates a function template that implements a binary search of a sorted array of objects. 2. (Extra)
1. (Extra) Write and test a program that instantiates a function template that implements a binary search
of a sorted array of objects.
2. (Extra) Implement and test a template for generating Queue classes. A queue works like a stack,
except that insertions are made at one end of the linear structure and removed from the other end.
3. Enhance the Vector
a. Overload operator string() to return a string of all items.
b. Overload operator<<() to print out the content of each item in the items.
c. Add T& findMax() to return the biggest item
d. Add T& findMax() to return the smallest item
4. Implement a Book class that can be used to create a template of instance of Vector
have other member functions if needed. The Book class has at least data members: title and price.
a. Overload operator<<() to print out the contents of book.
5. Implement a Student class that can be used to create a template of instance of Vector
the Student class has at least these data members id, name, gpa, and Vector
get function Vector
a. Overload operator<<() to print out the contents of student.
b. Overload operator>() to check if this students GPA is bigger than others.
c. Overload operator<() to check if this students GPA is smaller than others.
6. Implement a Course class that can be used to create a template of instance of Vector
data members: course title and Vector
GetStudents(); It may have other member functions if needed.
a. Add Student& getBestStudent() to return the student who has the highest GPA.
b. Add Student& getWeakestStudent() to return the student who has the lowest GPA.
7. Write a main function that simulates a school model. The school has 2 courses, each course has 2 to
10 students, and each student has 1 to 5 books. So, you can create an instance of Vector
represent the school, e.g.. Vector
8. Fill the school object with some sample data (i.e. Since the school object has 2 courses, you will need
to assign data for these two courses, and each course should have 10 students data and their books).
Call the print function that you created in step 3a to print out the entire the school object information.
#include
template
protected: T* items;
unsigned int size;
void copy(const Vector
{
int minSize = (size < v.size)? size : v.size; for (int i = 0; i < minSize; i++)
items[i] = v.items[i];
}
public:
Vector(unsigned int n = 10) : size(n) { items = new T[size];
}
Vector(const Vector
}
Vector
items = new T[size]; copy(v);
return *this;
}
T& operator[] (unsigned int i) const { return items[i];
}
unsigned int getsize() { return size;
}
~Vector() { delete [] items;
}
};
int main()
{
Vector
for (int i = 0; i < v.getsize(); i++)
{
v[i] = rand() % 100;
}
for (int i = 0; i < v.getsize(); i++)
{
cout << v[i] << '\t';
}
cout << endl; return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
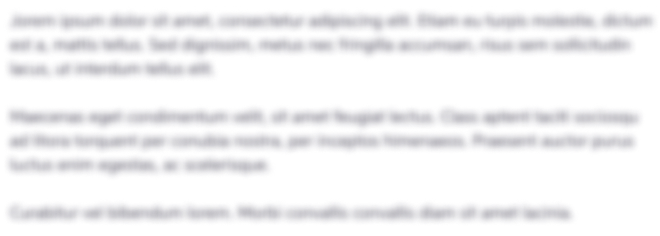
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started