Question
1. import java.util.* public class Exercises18HPQ { public static void main(String[] args) { // BJP HeapPriorityQueue modified: HeapPriorityQueue pq18 = new HeapPriorityQueue(100); pq18.add(88); pq18.add(-1); pq18.add(35);
1.
import java.util.*
public class Exercises18HPQ {
public static void main(String[] args) { // BJP HeapPriorityQueue modified: HeapPriorityQueue pq18 = new HeapPriorityQueue(100); pq18.add(88); pq18.add(-1); pq18.add(35); pq18.add(42); pq18.add(42); System.out.println(pq18); // uses PQ toString() // Some more Oracle Java methods: System.out.println(Arrays.toString(pq18.toArray())); // PQ return and Array System.out.println(pq18.remove(42)); // removes just a single Object System.out.println(pq18); // uses PQ toString() System.out.println(pq18.remove(99)); // removes just a single Object System.out.println(pq18); // uses PQ toString() System.out.println(pq18.poll()); // Oracle PQ actually uses poll to remove System.out.println(pq18); // uses PQ toString() pq18.clear(); // clears the Queue System.out.println(pq18); // noting left at this point // Some CONSTRUCTOR options with Oracle Java HeapPriorityQueue: // Build a Collection to use in testing below Collection storage = new ArrayList(); storage.add(42); storage.add(17); storage.add(9); storage.add(42); storage.add(35); storage.add(-1); storage.add(88); System.out.println(storage); // output simply in order of above input // Oracle Java HeapPriorityQueue has constructor to accept Collection: HeapPriorityQueue pq = new HeapPriorityQueue(storage); System.out.println(pq); // prints as internal array order (KNOW what this is!!!) while (!pq.isEmpty()) { System.out.print(pq.remove() + " "); // prints in "natural" order } System.out.println();
// Oracle Java HeapPriorityQueue has constructor to accept a different Comparator: HeapPriorityQueue pqMax = new HeapPriorityQueue(Collections.reverseOrder()); pqMax.add(88); pqMax.add(-1); pqMax.add(35); pqMax.add(42); pqMax.add(9); pqMax.add(17); pqMax.add(42); System.out.println(pqMax); // prints as internal array order while (!pqMax.isEmpty()) { System.out.print(pqMax.remove() + " "); // prints in Comparator's order } System.out.println();
} }
2.
import java.util.Arrays; import java.util.Collection; import java.util.Comparator; import java.util.NoSuchElementException;
public class HeapPriorityQueue // move rightmost leaf to become new root elementData[1] = elementData[size]; size--; // "bubble down" root as necessary to fix ordering int index = 1; boolean found = false; // have we found the proper place yet? while (!found && hasLeftChild(index)) { int left = leftChild(index); int right = rightChild(index); int child = left; if (hasRightChild(index) && elementData[right].compareTo(elementData[left]) 0) { swap(elementData, index, child); index = child; } else { found = true; // found proper location; stop the loop } } return result; } // Returns the number of elements in the queue. public int size() { return size; } // Returns a string representation of this queue, such as "[10, 20, 30]"; // The elements are not guaranteed to be listed in sorted order. public String toString() { String result = "["; if (!isEmpty()) { result += elementData[1]; for (int i = 2; i 1; } // returns true if the node at the given index has a non-empty left child private boolean hasLeftChild(int index) { return leftChild(index) }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
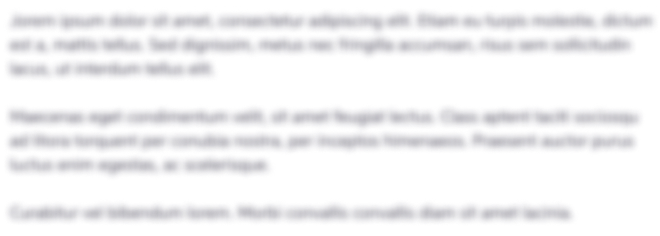
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started