Question
1 import java.util.Scanner; 2 import java.util.Random; 3 /* This class manages the playing of human vs. computer games. In this version, only the RPS game
1 import java.util.Scanner; 2 import java.util.Random; 3 /* This class manages the playing of human vs. computer games. In this version, only the RPS game is 4 used (although imagine that other games could be implemented in the future). 5 The GameMain class handles all of the communication between the player and the game instance. 6 There are two possible instances of the Random class that can be passed to the game instance, 7 one seeded for debugging and one unseeded for random (normal) play. 8 9 The code below first prints a welcome message. 10 Then it creates a Scanner instance for console input as well as an instance of the RPS game (passing an 11 instance of Random to its constructor). It then enters a loop as long as the player wants to play a round. 12 When the player is done they enter an "X" and the loop stops. A good bye message is printed. 13 */ 14 public class GameMain { 15 16 public static void main(String[] args){ 17 18 System.out.println("Welcome to Rock, Paper, Scissors. You will play against the computer ;-) "); 19 /* Create the Scanner, Random, and RPSGame instances. 20 You will also want variables to keep the player and computer choice 21 and any other variables needed. 22 */ 23 Scanner scan = new Scanner(System.in); 24 boolean keepPlaying = true; 25 String playerStr = "", computerStr = ""; 26 // using a seed means the same sequence of numbers will be generated. 27 int seed = 123456; 28 Random rnd = new Random(seed); 29 // use this for truly random play: 30 //Random rnd = new Random(); 31 RPSGame game = new RPSGame(rnd); 32 33 // The looping section 34 while(keepPlaying) { 35 System.out.println("Enter R for rock, P for paper, or S for scissors. Enter X to quit."); 36 playerStr = scan.nextLine(); 37 System.out.println("You entered: "+playerStr); 38 if(playerStr.equalsIgnoreCase("X")) 39 keepPlaying = false; 40 else if (game.isValidInput(playerStr)){ 41 // play a round 42 game.playRound(playerStr); 43 computerStr = game.getComputerChoice(); 44 System.out.println("The computer chose: "+computerStr); 45 if(game.playerWins()) 46 System.out.println("You win!"); 47 else if(game.computerWins()) 48 System.out.println("Computer wins!"); 49 else 50 System.out.println("It's a tie!"); 51 System.out.println(game.getScoreReportStr()); 52 } 53 else // invalid input 54 System.out.println("Your input should be R, P, or S. Please enter again."); 55 } 56 System.out.println("Bye for now."); 57 } 58 }
MODIFY THE FOLLOWING --- MODIFY THE FOLLOWING--- MODIFY THE FOLLOWING
1 import java.util.Random; 2 public class RPSGame { 3 4 private Random rand; 5 private int playerScore; 6 private int computerScore; 7 private int rounds; 8 private String computerChoice; 9 private boolean playerWins; 10 private boolean computerWins; 11 12 /* The constructor assigns the member Random variable, rand, to 13 the instance passed in (either a seeded or non-seeded instance). 14 It also initializes the member variables to their default values: 15 rounds, player and computer scores are 0, the playerWins and computerWins 16 variables are set to false. The computer choice is initialized to the empty string. 17 */ 18 public RPSGame(Random r) { 19 } 20 21 /* This method returns true if the inputStr passed in is 22 either "R", "P", or "S", false otherwise. 23 */ 24 public boolean isValidInput(String inputStr) { 25 return false; 26 } 27 28 /* This method carries out a round of play of the RPS game. First, it calls resetRound so 29 that all variables from the last round are reset. Then, it gets the computer's choice 30 (by calling the getRandomChoice method), compares it to the player's choice and determines 31 who wins a round of play according to the rules of the game. 32 The player and computer scores are updated accordingly. 33 The computerWins and playerWins variables are updated accordingly. 34 The number of rounds of play is incremented. 35 */ 36 public void playRound(String playerChoice) { 37 } 38 39 // Returns the choice made by the computer for the current round of play. 40 public String getComputerChoice(){ 41 return null; 42 } 43 44 // Returns true if the player has won the current round, false otherwise. 45 public boolean playerWins(){ 46 return false; 47 } 48 49 // Returns true if the computer has won the current round, false otherwise. 50 public boolean computerWins() { 51 return false; 52 } 53 54 // Returns true if neither player nor computer has won the current round, false otherwise. 55 public boolean isTie(){ 56 return false; 57 } 58 59 // Returns the number of rounds, the player's current score, and the computer's current score in a String format. 60 public String getScoreReportStr(){ 61 return null; 62 } 63 64 /* This "helper" method uses the instance of Random to generate an integer 65 which it then maps to a String: "R", "P", "S", which is returned. 66 This method is called by the playRound method. 67 */ 68 private String getRandomChoice() { 69 return null; 70 } 71 72 /* Resets the variables that record state about each round: 73 computerChoice to the empty string and the playerWins and computerWins to false. 74 This method is called by the playRound method. 75 */ 76 private void resetRound(){ 77 } 78 }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
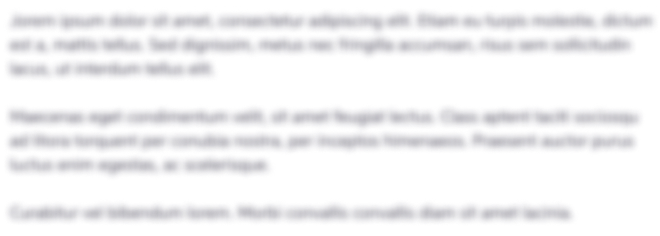
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started