Question
1. Include the appropriate file stream header file 2. Delete the global wordBank array declaration and numWords declaration 3. Modify the definition of main() to
1. Include the appropriate file stream header file
2. Delete the global wordBank array declaration and numWords declaration
3. Modify the definition of main() to allow for command line arguments to be accessed and add a check to ensure the user has entered enough command line arguments (i.e. provided something that can be used as the filename of their wordbank)
4. Add code to open the file using the command line argument provided as the filename. Check to ensure you could successfully open the file. If you fail to open the file, just output a nice error message and quit the program.
5. Attempt to read an integer from the file which is the number of subsequent words in the file. If you fail to read an integer successfully, close the file and exit gracefully with an appropriate message to the user.
6. Dynamically allocate an array of char*'s named 'wordBank' (one per word that you expect to read from the file) that will point at each character array (i.e. word) that you are about to read in.
7. Declare a character array (a.k.a 'buffer') of 41 characters max to hold the strings that you read in (one by one) from the file [You may assume the maximum word length will be 40 characters].
8. In a loop, read in each word from the file into the character array you just allocated, then dynamically allocate a new character array that is just the right size to hold that word. Copy the word from the buffer to your new array, and make sure your wordBank has a copy of the pointers to the array you just allocated (reference the code give in our slides about "Dealing with Text Strings" in the Pointer Unit Slides 53-57)
9. Close your input file
At this point the original word scramble code should be able to be reused (make sure variable names used in your code match those needed for the remainder of the code).
10. At the end of main deallocate all the memory you allocated with new
//need to create a worldbank.txt
the original code
// wscramble.cpp
// Word Scramble guessing game
// Illustrates string library functions, character arrays,
// arrays of pointers, etc.
#include
#include
#include
#include
using namespace std;
// Prototype
void permute(char items[], int len);
// Define an array of strings (since a string is just a char array)
// and since string are just char *'s, we really want an array of char *'s
const char *wordBank[] = {"computer","president","trojan","program","coffee",
"library","football","popcorn","science","engineer"};
const int numWords = 10;
int main()
{
srand(time(0));
char guess[80];
bool wordGuessed = false;
int numTurns = 10;
// Pick a random word from the wordBank
int target = rand() % numWords;
int targetLen = strlen(wordBank[target]);
// More initialization code
char* word = new char[targetLen+1];
strcpy(word, wordBank[target]);
permute(word, targetLen);
// An individual game continues until a word
// is guessed correctly or 10 turns have elapsed
while ( !wordGuessed && numTurns > 0 ){
cout << " Current word: " << word << endl;
cin >> guess;
wordGuessed = (strncmp(guess, wordBank[target], targetLen+1) == 0);
numTurns--;
}
if(wordGuessed){
cout << "You win!" << endl;
}
else {
cout << "Too many turns...You lose!" << endl;
}
delete [] word;
return 0;
}
// Scramble the letters
void permute(char items[], int len)
{
for(int i=len-1; i > 0; --i){
int r = rand() % i;
int temp = items[i];
items[i] = items[r];
items[r] = temp;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
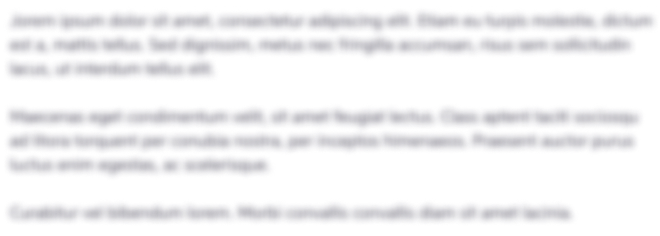
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started