Question
1 INFSCI 0017 Assignment 4 You are doing an internship in a company specialized in create role-playing computer games. As a part of the programming
1 INFSCI 0017 Assignment 4 You are doing an internship in a company specialized in create role-playing computer games. As a part of the programming team, you are asked to implement and test the first version of a RolePlayer class. In the game, a RolePlayer is a knight with the mission of fight and kill monsters: vampires and werewolves. As the player defeat monsters, he/she gains points. The player is considered trainee if he/she has less than 1000 points, a "beginner" with more than 1000 points and less than 3000, and a "master" when he/she reaches 3000 points. Each time the player wants to fight a monster, he/she toss a dice one or more times, depending on the level. A trainee toss the dice twice. A beginner toss the dice 3 times, and a master toss the dice 4 times. To defeat a vampire, the player needs at least 11 points. To defeat a warewolf, the player needs at least 7 points. A defeated vampire makes 300 points. A defeated werewolf makes 150 points. 1. Create a Eclipse project named [your pitt id]_assignment4 2. Create a package edu.pitt.is17.[your pitt id].assignment4 3. Add the class Dice to your package. It represent a cube dice (6 possible values.) This class is already implemented and you have to use it as is (do not modify it!!) public class Dice { int sides; public Dice(){ sides = 6; } public int roll(){ return (int) (sides*Math.random() + 1); } } 4. Implement the class Monster following the class diagram. Add a constructor which receives a string contaning "vampire" or "werewolf" and sets the attribute specie. Make the constructor validate that the specie is "vampire" or "werewolf" (regardless case -uppercase or lowercase) and set it as a "werewolf" as a default value if specie parameter is not valid. Add a getter for attribute specie, but do not add a setter method. 5. Implement the class RolePlayer as defined by the design team of the company in the following diagram. Methods are described in the side. fight: depending on the score of the player, it rolls the dice 2, 3 or 4 times. Depending on the sum of the dice tosses and the opponent specie ("vampire" or "werewolf") the fight is won or lost. See the rules described in the first paragraph. Make sure it can recognize "vampire" or "werewolf" no mater if lowercase or uppercase. If the figth is won, points are added to the attribute score of the player (makes 300 points per vampire and 150 points per werewolf.) The method returns true if the fight is won, and false if not. level: returns "trainee", "beginner" or "master" depending on the value of the attribute score. See rules described in the first paragraph. getScore: returns the attribute score value. Add a constructor receiving the name. Also, in the constructor initialize score in 0 and create the dice. Add a getter method for name attribute. 2 6. You know that good practices of programming are quite important, so consider to define and use constants while implementing the RolePlayer class. For example, 1000 points defines the lower bound for being a "beginner". Thus, a constant can be defined to be used: private final int BEGINNER_LOWER_BOUND = 1000; Folow a similar approach for all literal values you use in your program. 7. Test your RolePlayer class with the following program. Make sure the methods in the class RolePlayer work well. public class RoleGameTest { public static void main(String[] args) { RolePlayer player = new RolePlayer("John Snow"); battle(player,new Monster("vampire")); battle(player, new Monster("werewolf")); battle(player, new Monster("werewolf")); battle(player, new Monster("werewolf")); battle(player, new Monster("vampire")); battle(player, new Monster("werewolf")); battle(player, new Monster("vampire")); battle(player, new Monster("vampire")); battle(player, new Monster("vampire")); battle(player, new Monster("werewolf")); } public static void battle(RolePlayer p, Monster opponent){ if (p.fight(opponent)) { System.out.println(p.getName() + " fought a " + opponent.getSpecie()+" and win (level: " + p.level() + ", score: "+p.getScore() + ")"); }else{ System.out.println(p.getName() + " fought a " + opponent.getSpecie()+" and lost (level: " + p.level()+ ", score: "+p.getScore() + ")"); } } } General aspects: Write meaningful comments for every variable and every step in your solutions make sure to explain what each variables represents. The goal is to make you explicitly reflect about every step in your program. Follow the name conventions for naming your classes, variables and constants. Make sure your indentation is correct. You can use Correct Indentation menu option in Eclipse before submitting your code. Export your project and compress it in a file named [your pitt id]_assignment4.zip. Due date is Monday Feb 29th 23:59 PM. Submit using courseweb submission tool.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
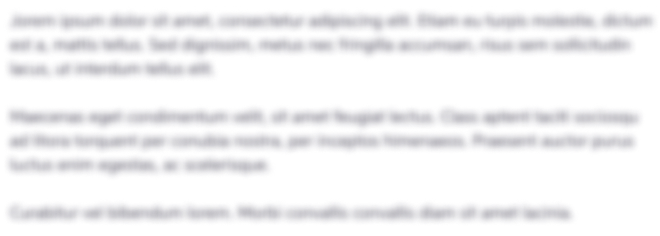
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started