Question
1 Introduction Nim is a two player game where players alternate removing stones from piles, trying to force the other player to take the last
1 Introduction
Nim is a two player game where players alternate removing stones from piles, trying to force the other player to take the last poison stone. There are three piles of stones each starting with between 5 and 11 stones (chosen randomly when the game begins). The game alternates players. If a players turn begins with only one stone left (one in one pile, none in the other piles), that player loses. Otherwise on a players turn they select a (non-empty) pile of stones and in dictate how many stones they wish to remove. They can remove all of the stones (from that pile) if they wish but they must remove at least one stone. Those stones are removed from the pile and play now moves to the next player. The game continues until a player is left with one pile containing a single stone and two empty piles. As mentioned before, that player is the loser. Note: No player is allowed to actually remove the last stone.
2 Files
We will develop this game in two pieces. First we will create a nim class that implements the rules of the game. It will keep track of which players turn it is, the number of stones in each pile, and will inform us when a player loses. Next we will create a driver. This will include a main function but may have as many other functions as you like. This driver will allow two players to interactively play the game. Create three les: nim.h (the header le), nim.cpp (the implementation le) and nim game.cpp. Get these les started as I have done in class several times (macro guard, includes, an empty main function in the nim game.cpp le etc).
3 Instance variables
A Nim game needs to know: Which players turn it is. An int players turn makes sense for this variable. The number of stones in each pile. An array of three ints would work here. I suggest declaring this as int piles[3] in the appropriate place in your header le.
Add these in stance variables to your class declaration in your header le. These are the only instance variables your class will have.
1
4 Constructor, displaying the board and a test driver
Each pile starts with between 5 and 11 stones, according to our rules (there are actually lots of games called Nim which differ quite a bit). Create a constructor that takes three integer arguments, one for each pile indicating the starting number of stones in that pile. Use assert to check that the arguments are valid. In addition to setting your piles variables, your constructor should set your players turn variable to 1, since the game always starts on player ones turn. Add a void print() method that displays the number of stones in each pile and which players turn it is. In your drivers main function, create a nim game and print the board as follows: nim game (9 ,7 ,10); game. print ();
This should produce output resembling:
Player 1: 9 7 10
Next add a constructor that takes no arguments and creates a valid random nim game (documentation on the rand function is available in the C++ reference). For example: nim game; game. print ();
might produce
Player 1: 11 5 8
Moving the game along
Add the following methods to nim (both header and implementation les) and test them in your driver. Dont worry about making the driver actually play the game, just use it to automatically step through a game scenario.
int get players turn() will return a 1 or a 2, depending on whose turn it is bool take turn(int pile, int stones to remove) If the pile and stones to remove are valid, the stones should be removed from the appropriate pile, turn should be advanced to the next player and this method should return true. Note that the pile numbers are 1, 2 and 3. If the player tries to remove an invalid number of stones or an invalid pile number, their request should be ignored (no stones removed) and false should be returned. Please be careful to check special cases such as the player trying to take all of the stones from the last pile (which is not allowed). bool is game over() return true if there is only a total of one stone left. Note that you can call get turn() to nd out who lost.
Heres some driver code to show you how I worked through creating the methods above.
void test () { // I can take a few turns in this game nim game(11 ,10 ,5); cout << game. is game over () << endl ; // should be false cout << game. get players turn () << endl ; // should be 1 game. take turn (1 , 10); // remove 10 stones from pile 1 cout << game. get players turn () << endl ; // should be 2 game. print (); // Player 2: 1 10 5 game. take turn (2 , 10); // remove 10 stones from pile 2 game. print (); // Player 1: 1 0 5 game. take turn (3 , 5); // remove 5 stones from pile 3 game. print (); // Player 2: 1 0 0 cout << game. is game over () << endl ; // should be true
// . . .
} int main () { test (); return EXIT SUCCESS; } We arent actually playing the game yet, just making sure that the nim class is working.
Interactive Game
Now we want to play the game by taking input from the user. I kept my test code (above) but modied main to call a different function, play(), where I wrote the interactive game. You might want to do this as well so that if you need to use your tests later, you can. So, what should play look like? Heres some pseudo-code to get you going (Note: the interactive game should start with a random board):
play: create a nim game with random piles while the game isnt over display the game board ask the current player for their move tell the game to make the move and if the move fails, display an error display the winning and losing player
Implement this in C++ :)
3
7 Play the game
Makesureyourgameisworking. Asksomeonetoplaywithyousothattheycanhelpyoundanybugsinyourgame.
To hand in Submit a zip le of your complete solution (all source code)
I Have this need to make it have the correct files random number and including having a class set up and a testing method and what ever I missed in the instructions
#include
using namespace std;
int main()
{
int numOfMarbles, turn, marblesToPick;
cout<<"Enter the initial number of marbles in the pile: ";
cin>>numOfMarbles;
cout<<"Enter the number of player with first turn 1. Player-1. 2. Player-2: ";
cin>>turn;
while(turn < 1 || turn > 2)
{
cout<<"This game has only 2 players. ";
cout<<"Enter the number of player with first turn 1. Player-1. 2. Player-2: ";
cin>>turn;
}
while(numOfMarbles != 1)
{
cout<<"Player-"< cin>>marblesToPick; while(marblesToPick < 1 || marblesToPick > numOfMarbles/2) { cout<<"The marbles you're trying to pick is out of range. "; cout<<"Player-"< cin>>marblesToPick; } numOfMarbles -= marblesToPick; turn = turn % 2 + 1; } cout<<"Only one marble left. And its Player-"< cout<<"Player-"< return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
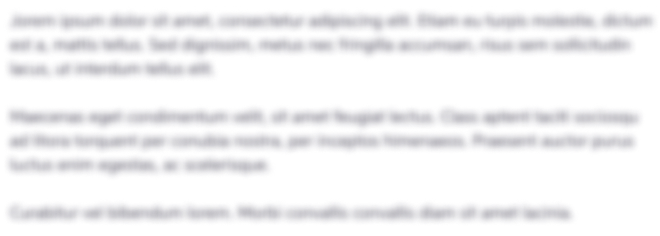
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started