Question
1 Introduction NOTE: read the whole assignment brief first before implementing it contains very important information In this assignment you will be tasked with doing
1 Introduction NOTE: read the whole assignment brief first before implementing it contains very important information In this assignment you will be tasked with doing four things: Create a simulation of a sample stock market based on 5 years of S&P 500 stock data with a portfolio that can take a maximum of 10 stocks at any time. You will simulate buying and selling of stocks with the aim of producing the largest return value at the end of 5 years of trading A mapping onto the simanneal library that will use Simulated Annealing algorithm to determine the best strategy A mapping onto the deap library that will use genetic evolution to determine the best strategy Using both mappings to determine the best strategy in a number of situations different stock metrics In terms of effort the vast majority of your time will be spent on the first part in creating the simulation. The mappings to both libraries will not take long, and the experimentation to find the best strategy will take about 3 to 5 minutes per run using either annealing or evolution. It is recommended that when you have completed your simulations that you test them with the test case given below. if you get the same results at the end then your simulation is matching the simulation that we are using. Remember if you produce a bad simulation your results from numerical optimisation cannot be trusted. Read the details of the simulation carefully and if you have any questions do not hesitate to ask. You will need to have full clarity on the simulation in order for implementation to be correct and by consequence your numerical optimisation to be correct
2 Simulation Details The reason why simulations of this nature is needed is that strategies for trading stocks can be trialled with virtual money without risk of losing money. With the stock market there are two things that we are trying to predict at all times. 1. When is the best time to purchase a stock 2. When is the best time to sell a stock In the ideal case you should purchase a stock when its value is lowest and sell it when its value is highest. The difference in share price between when the stock was purchased and when it was sold is the profit or loss that was made on that stock. Thus a lot of people are highly interested in modelling the stock market to see if there is a clear cut strategy that can determine in advance when the right time to buy and sell will occur in order to maximise profit. The two main methods that are used are: 1. Techincal Analysis 2. Fundamental Analysis Techincal analysis which is used here basically looks at the historical stock price and tries to use that alone to predict how the stock will change. Fundamental analysis requires that you look at the profile of a company (assets etc) and their balance sheets to see if they are worth investing in. We will not use the latter approach. In this particular case we will have a portfolio of 10 slots. For each slot on day one you will start with an amount of $2,000. meaning you will have a maximum of $20,000 to invest in this version of the stock market. On day 1 (Day 128 in simulation time, will explain this later) you will purchase as many shares as possible in the 10 most desirable stocks. Every 30 days you will then decide if you should sell any of these stocks. Should one or more stocks be sold you will then purchase alternative stocks using a similar mechanism to day 1 to ensure you still have 10 stocks purchased at all times. The process will then repeat where you hold onto those shares for another 30 days and determine again if you need to replace shares. The reason for the 30 day limit is to ensure that when you apply Simulated Annealing and Genetic Algorithms to this problem it will complete all iterations/generations in 3 to 5 minutes. If this was reduced to every day the numerical optimisation could easily take several hours to complete. Once you apply optimisation to this problem using either approach you should be aiming to get a final portfolio value between $120,000 and $170,000 after the 5 year period of the dataset has completed.
3 Classes Discussion of the classes that you will need in order to write the simulation 2 3.1 StockFrame A class to represent a stock and its associated values on each given day in the dataset. This definition is provided for you in the base script and should not be modified. init : constructor for the class that takes in a number of properties that are set on the stock for this particular day self.closing: The value of a single share of this stock on this day. self.volume: How many shares of this stock were traded on this day. self.sma ema: A collection of four Simple Moving Averages, for the last 8, 16, 32, and 64 days. also 4 Exponential Moving Averages, for the same time periods self.sma ema slope: not to be used but present in the data self.sma ema curvature: not to be used but present in the data self.profit loss: The profit and loss of the stock compared to the value of the stock 1, 2, 4, 8, 16, 32, 64, and 128 ago. 3.2 Portfolio A class to represent a portfolio of stocks. Will also be responsible for simulating the stock market that we have as well as purchasing and selling stocks. init : takes in six parameters in addition to self: stocks: How many stocks are in the dataset days: How many days worth of stocks are in the dataset initial funds: The starting funds for each slot in the portfolio stock frames: The list of stock frames containing all stock data stock names: The ticker names of all stocks e.g. AAPL for Apple total stocks: How many slots this portfolio supports This function constructs and initialises a portfolio. It should take a copy or reference of all six parameters provided and then construct lists to store the funds available for each slot (defaulted to initial funds), a list to store how many shares of a stock was purchased, a list to store the share price of the stock when it was purchased, and finally a list of indexes to the stock itself. You should also initalise a list of 8 weights for a stock along with two thresholds one for purchasing a stock and one for selling a stock. haveStock(self, index): checks to see if the provided stock index exists in the list of stock indexes. If yes return true, false otherwise MACWeightsValues(self, weights, values): takes two lists exactly 8 elements long and multiplies each list element pairwise before adding it to a total that is returned. 3 purchaseStocks(self, day, buy indexes): takes in the current day of the simulation and a list of tuples containing a buy weight and a stock index. This list should be in reverse order from highest buy weight to lowest buy weight. The function should first remove any stocks from the list that have already been purchased after this for each empty slot the maximum amount of shares of the most desirable stock should be purchased (you may need to use math.floor() here as partial shares are not permitted). Once a stock has been purchased it should be removed from the list. Stocks should be purchased until either all slots or filled or buy indexes is emptied. Note a stock should only be purchased if it has a closing value of 0.01 or higher. reset(self): This should reset the portfolio to as it was on day one with all initial funds present and no stocks purchased. sellStock(self, day, slot): sells the stock in the given slot for whatever value the relevant stock has on that day. it should add these funds to the total funds available for that slot. shouldBuy(self, day): goes through all stocks on a given day and determines if they would be desirable to purchase. It will go through all stocks for a given day (the offset (day * self.stocks) will be useful to you to select the right set of stock frames for a given day) and by using MACWeightsValues on the buy weights and the profilt and loss values of a stock it should calculate a buy weight. if this is above the required threshold a tuple containing the stock index and the buy weight should be added to a list. Once evaluation of all stocks is finished this list should be sorted in reverse order of buy weight (highest weight first). An attempt to purchase stocks should then be made shouldSell(self, day): goes through the list of stocks currently held and applies the buy weights to the profit and loss of each stock for the current day. if any of the stocks fall below the sell threshold it should be sold. simulate(self): simulates the stock market starting from day 128 to ensure we have enough data for profit loss to calculate correctly. every 30 days it should decide if any stocks should be sold. If stocks are sold it should purchase new stocks in the sold stocks place. totalValue(self, day): determines the total value of the portfolio on the given day. for each slot take the funds remaining for that slot and add in the value of the shares for that stock on the given day. updateBuyThreshold(self, threshold): sets a new buy threshold on this portfolio will be used by both optimisation methods updateBuyWeights(self, weights): takes the list of 8 buy weights and sets them this portfolio will be used by both optimisation methods. updateSellThreshold(self, threshold): sets a new sell threshold on this portfolio. will be used by both optimisation methods
please reply if you need the sample code given to us as well
if someone can do it contact i will pay as well
Step by Step Solution
There are 3 Steps involved in it
Step: 1
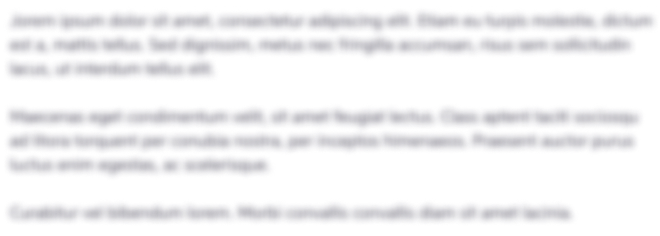
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started