Question
1 Introduction Your task for this assignment is to design, code (in C89), test and debug a program to draw terminal-based turtle graphics. In short,
1 Introduction Your task for this assignment is to design, code (in C89), test and debug a program to draw terminal-based turtle graphics. In short, your program will: Read a series of graphics commands from a file; Convert angles and distances to (x, y) coordinates as needed; Record these coordinates in a log (output) file; Use pre-defined functions to execute the commands; i.e. drawing lines on the screen. There is a lot of detail here. Read it carefully before you start anything. 2 Documentation You must thoroughly document your code using C comments (/* ... */). For each function you define and each datatype you declare (e.g. using struct or typedef), place a comment immediately above it explaining its purpose, how it works, and how it relates to other functions and types. Collectively, these comments should explain your design. (They are worth substantial marks see Section 7.) 3 Task Details Read this section carefully, there is a lot of detail, you may need to read it several times to ensure you do not miss anything. Input file Your program should accept a command-line parameter: the name of the file containing graphics commands. Your program should read the contents of this file into a linked list.
The file will consist of a sequence of commands, one on each line. There is no length indicator. Each command may be one of the following: ROTATE MOVE d DRAW d FG i BG i PATTERN c is a real-valued angle, d is a real-valued distance, i is an integer-valued colour code and c is a non-space character. Command names may be in lowercase or uppercase (or any combination). An example file might look like this: rotate -45 move 30 FG 1 Pattern # DRAW 10 Rotate 90 draw 10 ROTATE 90 dRAW 10 ROTATE 90 DRAW 10 (I have deliberately scrambled the casing here; this is still a valid input file.) Error Handling You must deal with any invalid file, this includes, but is not limited too: The file not existing; The file being empty; Lines that do not start with one of the specified commands; The incorrect number of parameters after a command; The incorrect data type after a command. Turtle graphics Your program must keep track of several changing pieces of information: Current position: for each command, you start at a particular position on the screen, described by real-valued (x, y) coordinates, where x begins at zero on the left of the screen, and y begins at zero at the top, increasing downwards. The MOVE and DRAW commands will change the current position. The initial position is (0, 0) (the top-left screen corner). Current angle: for each command, you start at a particular real-valued angle, expressed in degrees, from 0 to 360 . 0 means right; 90 means up. The ROTATE command will add or subtract from the current angle; i.e. rotating it anticlockwise or clockwise. The initial angle is 0 . Current foreground and background colours: for each command, you start with two colour codes representing the foreground and background colours. The terminal has 16 foreground colours (015) and 8 background colours (07). These are used every time any character is displayed. The FG command changes the foreground colour, and BG the background. The initial foreground colour is 7 (white) while the initial background colour is 0 (black). Current pattern: for each command, you start with a character that will be used to make up any lines you plot. The PATTERN command changes the current pattern(character). The initial pattern(character)4 is the + character. Your program must interpret and execute the sequence of commands, keeping track of the above information. Most importantly, the DRAW d command will draw a line d units long, starting at the current position and heading at the current angle. After the line is drawn, the current position will move to the other end of the line. The MOVE command works in the same fashion, except it does not actually draw a line. To do this, your program must calculate real-valued (x, y) coordinates from angles and distances. To this end, you will need to apply basic trigonometry (using math.h): x = d cos y = d sin When outputting lines, your program must round its real-valued coordinates to the nearest integer values, as required below. Existing Functions You must use the existing functions in the files effects.c and effects.h. The most important of these is line(), declared as follows: void line(int x1, int y1, int x2, int y2, PlotFunc plotter, void *plotData); This will draw a line from integer-valued coordinates (x1, y1) to (x2, y2). It has no idea of the current position, current angle, etc. The PlotFunc type is defined as follows: typedef void (*PlotFunc)(void *plotData); Page 3 of 8 UCP Semester 2, 2018 For each discrete point on the line, the line() will position the cursor at that point, then call the *plotter function. You must define this function yourself, and supply it to line(). The plotter function must generate the appropriate terminal output (i.e. the current pattern(character)). The final parameter to line() is a void*, which is simply passed on to the plotter function. This allows you to pass any required data between your algorithm and the plotter function. Several other functions are also available: void setFgColour(int) changes the foreground colour to a given colour code. void setBgColour(int) changes the background colour to a given colour code. void clearScreen() blanks the terminal. void penDown() moves the cursor to the bottom of the screen (for when drawing is complete). Log file (Consider this when developing test cases!) Your program must also append to (not overwrite) a log file called graphics.log. The first line written to the log file, after its existing contents, should be ---. This will serve to separate the new contents from the old. The log file must show the actual, real-valued (x, y) coordinates calculated for the DRAW and MOVE commands, in the following format: command (x1,y1)-(x2,y2). All coordinate values should be printed such that they line up vertically. An example graphics.log file (generated after only one execution) would be: --- MOVE ( 0.000, 0.000)-( 50.000, 33.333) DRAW ( 50.000, 33.333)-(100.000, 50.000) DRAW (100.000, 50.000)-( 50.000, 66.667) DRAW ( 50.000, 66.667)-( 50.000, 33.333) (This is not related to the previous example.) If the program were run a second time with the same input, graphics.log would become: --- MOVE ( 0.000, 0.000)-( 50.000, 33.333) DRAW ( 50.000, 33.333)-(100.000, 50.000) DRAW (100.000, 50.000)-( 50.000, 66.667) DRAW ( 50.000, 66.667)-( 50.000, 33.333) --- MOVE ( 0.000, 0.000)-( 50.000, 33.333) DRAW ( 50.000, 33.333)-(100.000, 50.000) Page 4 of 8 UCP Semester 2, 2018 DRAW (100.000, 50.000)-( 50.000, 66.667) DRAW ( 50.000, 66.667)-( 50.000, 33.333) The second half of the log file would be added on the second execution. Makefile Targets You must provide a makefile for your assignment. Failure to provide a makefile will result in zero (0) marks being awarded for compilation/execution/test cases. Your makefile must provide the following targets. TurtleGraphics The compiled assignment as described above. TurtleGraphicsSimple This version of the assignment should generate all line art with a white background and black foreground. That is, any FG or BG commands should be ignored. TurtleGraphicsDebug This version of the assignment should print the log file entries to the terminal as well as the file. The log file entries should be interspersed with the turtle graphics, that is, each call to line(...) should be preceded by any relevant log file entries.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
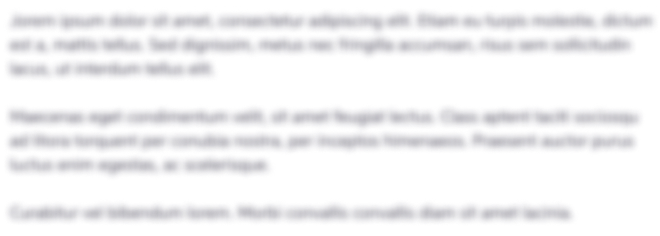
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started