Question
1. Java provides output facilities through various classes. You are likely very familiar with System.out by now (using its output methods print/println/printf). Inspecting the System
1. Java provides output facilities through various classes. You are likely very familiar with System.out by now (using its output methods print/println/printf). Inspecting the System class you will see that out, a static field, is an instance of thePrintStream class. This endows it with many methods such as print/println/printf.
To write to a file, we will use the java.io.PrintWriter class. It is quite similar to System.out in that PrintWriters haveprint/println/printf methods. When constructing a PrintWriter, the constructor determines the destination of output. Should a File be passed in, all output is directed the file.
PrintWriter pw1 = new PrintWriter(System.out); PrintWriter pw2 = new PrintWriter("myfile.txt");
Note: PrintWriters do not always push their output to the destination immediately.
When printing to a file, call close() to ensure all output is pushed to the file
When printing to the screen, one may need to call flush() to get text to appear (especially when you've written part of a line but not the newline character). In subclasses, flush() can be added to print/println to do this.
Make sure NOT to call close() on a PrintWriter that is writing to the screen: this will close System.out and prevent ALL further output from being printed to the screen.
2. We will create variants of the PrintWriter class by inheriting from it and creating small changes in behavior. Specifically, we will create a CensoredWriter class that selectively finds and replaces given words with %!^*#@ characters to clean up the text.
Goals
Making CensoredWriter a child class of PrintWriter
CensoredWriter will thus inherit print and println methods
CensoredWriter will change what the print and println methods do: it will transform input to them.
Transformations will be done in a special transform() method of the CensoredWriter. This is a method that the parent classPrintWriter does not have.
Transformations of output will utilize String class methods which will replace matched string patterns with the censored text
Transformed text will be passed to the parent class print and println methods which will perform the printing.
Several constructors for CensoredWriter will be provided so that output can be sent to the screen or to a file.
All other methods of CensoredWriter will be inherited from PrintWriter and will behave identically to the parent class.
Sample Sessions
The intended use for CensoredWriter is demonstrated below in an interactive session.
> CensoredWriter out = new CensoredWriter(System.out, "frack"); > out.println("When looking for fossil fuels, these days it is efficient to frack for natural gas.") When looking for fossil fuels, these days it is efficient to %!^*#@ for natural gas. > out.println("Wait, why can't I say frack now?") Wait, why can't I say %!^*#@ now? > out.println("What the frack!?!") What the %!^*#@!?! > out.println("Hey, frack you, you stupid fracking censor. What about free fracking speech?") Hey, %!^*#@ you, you stupid %!^*#@ing censor. What about free %!^*#@ing speech? > out.println("Poop. Didn't see that one coming did you?"); Poop. Didn't see that one coming did you? > out = new CensoredWriter(System.out, "poop"); > out.println("Big steaming piles of poop on you."); Big steaming piles of %!^*#@ on you. > out.println("Frack. :-p"); Frack. :-p > out = new CensoredWriter(System.out, "[Pp]oop|[Ff]rack"); > out.println("What's the matter? Can't you censor frack and poop at the same time?") What's the matter? Can't you censor %!^*#@ and %!^*#@ at the same time? > out.println("Fracking regular expressions") %!^*#@ing regular expressions
The last few lines demonstrate that the censored string is actually a regular expression allowing for a variety of patterns to be captured. You do not need to know anything about regular expressions to complete this lab. Instead, replacements will be done using String methods which will handle regular expressions without any effort on your part. We may discuss RegExs later in the course as they are very useful as evidenced by the above ability to replace multiple matching words. Investigate on your own if you are curious.
It is a simple matter to redirect output for a CensoredWriter to a file as well. Here is an example.
// Writing to a file > out = new CensoredWriter("somefile.txt", "frack"); > out.println("When looking for fossil fuels, these days it is efficient to frack for natural gas."); > out.close(); // somefile.txt now contains the censored text
3. public class CensoredWriter extends PrintWriter
fields
No fields are explicitly required, but you'll probably need to make your own fields to track the string pattern to be censored and the string to replace the censored string: %!^*#@
constructors
Note that PrintWriter has similar constructors to these, so each simple to write by invoking the parent constructing using super.
public CensoredWriter(OutputStream o, String c).
you have to call the parent class's constructor first; which one will you choose?
what other work remains after the parent constructor call - do you have any fields that were introduced in this class that still need assigning?
Sample uses of this constructor are:
CensoredWriter out = new CensoredWriter(System.out, "frack"); out.println("Some text which contains frack and frack.");
This constructor and the other two costructors should be very short, on the order of 1-2 lines.
public CensoredWriter(File f, String c) throws Exception. Same as above, but writes to the given file. Which parent-class constructor will you call?
public CensoredWriter(String filename, String c)throws Exception. Same as above, but writes to the file with the given name. Which parent-class constructor will you call?
methods
public String transform(String s). Performs the censoring by replacing all targeted censor-text matches with the censored string. The String::replaceAll() method is handy here, take a look! This method is public to allow for easy testing.
Here is an example use in DrJava.
> CensoredWriter out =new CensoredWriter(System.out, "poop"); > String s = out.transform("Everbody poops"); > s "Everbody %!^*#@s" > out.transform("Everbody poops") "Everbody %!^*#@s" out.transform("I'd like to see the poop deck. Can you arrange a poop deck tour?") "I'd like to see the %!^*#@ deck. Can you arrange a %!^*#@ deck tour?"
@Override public void print(String s). transform the given input first, then invoke the inherited version of printing. Go ahead and also call the inherited flush() method at the end, to ensure all output is sent now.
@Override public void println(String s). transform the given input first, then invoke the inherited version of printing. Go ahead and also call the inherited flush() method at the end, to ensure all output is sent now.
Examples:
> CensoredWriter cw = new CensoredWriter(System.out, "whine|whining"); > cw.println("No whining") No %!^*#@ > cw.println("Only wimps whine when the assignment is hard so stop whining and start coding") Only wimps %!^*#@ when the assignment is hard so stop %!^*#@ and start coding > cw.transform("Only wimps %!^*#@ when the assignment is hard so stop %!^*#@ and start coding") "Only wimps %!^*#@ when the assignment is hard so stop %!^*#@ and start coding" > CensoredWriter cw = new CensoredWriter("somefile.txt", "whine|whining"); > cw.println("Only wimps whine when the assignment is hard so stop whining and start coding"); > cw.close();
Step by Step Solution
There are 3 Steps involved in it
Step: 1
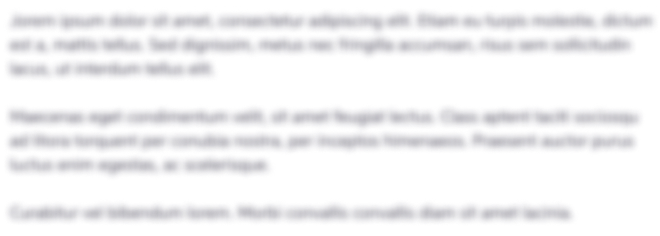
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started