Question
1) Left uncaught, a thrown exception will cause a compile error in Visual Studio. Select one: a. FALSE b. TRUE 2) Only a pointer variable
1) Left uncaught, a thrown exception will cause a compile error in Visual Studio.
Select one:
a. FALSE
b. TRUE
2) Only a pointer variable can be set to have the value NULL.
Select one:
a. FALSE
b. TRUE
3) Only a class method can be marked virtual.
Select one:
a. FALSE
b. TRUE
4) For a class Foo, one difference between a variable declared Foo * and a variable declared Foo & is that only the variable declared Foo * can potentially have the value NULL.
Select one:
a. FALSE
b. TRUE
5) Whenever they are passed as parameters to a function, the IO classes istream and ostream must be passed using a pass-by-reference parameter passing scheme.
Select one:
a. TRUE
b. FALSE
6) When no class constructors are supplied by the author of a class, that class will generated errors when compiled in C++.
Select one:
a. TRUE
b. FALSE
7) The body of a do { ... } while(...); loop will always executes atleast once, no matter what test condition is stated.
Select one:
a. FALSE
b. TRUE
8) The body of a while(...) { ... } loop will always executes atleast once, no matter what test condition is stated.
Select one:
a. TRUE
b. FALSE
9) A throw(...) statement is an alternative way to return from a function or method that indicates some kind of failure occurred.
Select one:
a. FALSE
b. TRUE
Please consider the following code fragment when answering the next few questions:
char[] var1 = Hello; | // a c-string variable |
char * var2 = new char( 20 ); strcpy( var2, Hello ); | // a c-string variable |
char var3[ 5 ] = { H, e, l, l, o }; | // an array |
std::string var4( Hello ); | // a string variable |
10) The variable named var2 declared above will include an ending NULL character.
Select one:
True
False
11) The variable named var4 declared above is an object and therefore has callable methods driver code can call.
Select one:
a. FALSE
b. TRUE
12) A function is allowed to have more than one return statement.
Select one:
True
False
13) When a function changes the value of reference parameter, the value of the actual parameter will get changed as well.
Select one:
True
False
14) In C++, a class' constructor is allowed to accept parameters.
Select one:
True
False
15) When authoring a friend function, the keyword friend only appears in the .h file, NOT the .cpp file.
Select one:
True
False
16) When a class method is marked with the keyword friend in a .h file,
Select one:
a. it will be a compile error if C++ programmers mark that class method with the keyword friend in the class' .cpp file.
b. it will be required for C++ programmers to mark that class method with the keyword friend in the class' .cpp file.
c. it will be a link error if C++ programmers mark that class method with the keyword friend in the class' .cpp file.
d. it will be a run-time error if C++ programmers mark that class method with the keyword friend in the the class' .cpp file.
e. None of the choices here are correct.
17) The expression:
( x > y && a < b )
will evaluate to true at run-time if x > y is true or a < b is true.
Select one:
True
False
18) When a class method is marked with the keyword const in a .h file,
Select one:
a. it will be a compile error if C++ programmers mark that class method with the keyword const in the class' .cpp file.
b. it will be a run-time error if C++ programmers mark that class method with the keyword const in the class' .cpp file.
c. None of the choices here are correct.
d. it will be a link error if C++ programmers mark that class method with the keyword const in the class' .cpp file.
e. it will be required for C++ programmers to mark that class method with the keyword const in the class' .cpp file.
19) In addition to redefining existing operators, C++ allows programmers to create their own new operators.
Select one:
True
False
20) If operator+ is supported by a class, how many parameters should it be defined to accept?
Select one:
a. 3
b. None
c. 1
d. 2
e. 1 or 2
21) In an infinite while loop, the expression controlling the loop will initially evaluate to false, but after the first iteration it will always evaluate to true.
Select one:
True
False
22) If operator >> is supported by a class, how many parameters should it be defined to accept?
Select one:
a. None
b. 1 or 2
c. 1
d. 3
e. 2
23) A member of the class Sample that is marked private
Select one:
a. is accessible by any subclass of Sample
b. is not accessible outside the class Sample
c. is accessible by driver code
d. is accessible by any parent class of Sample
24) The access modifiers public and private can be listed many times within a class' header (.h) file.
Select one:
a. FALSE
b. TRUE
25) The following code:
SpaceShip s; cout << s; calls the operator<< defined as: friend SpaceShip operator<<( ostream& outs, const SpaceShip & s );.
Select one:
a. FALSE
b. TRUE
26) In C++, the class string is part of the namespace cs52 and requires that programmers #include
Select one:
a. TRUE
b. FALSE
27) When declaring an array of classtype such as:
Student myStudents[ 10 ]; a programmer can tell C++ which constructor to use to initialize each of the elements in this array.
Select one:
a. TRUE
b. FALSE
28) The data members of a class are used to represent the "actions" that thing knows how to perform.
Select one:
a. TRUE
b. FALSE
29) Consider the following function declaration:
int foo( int a, int b, int c, int d=12, int e=20 );
What is the fewest number of parameters that must be passed at run-time to call this function?
Select one:
a. 5
b. 1
c. 2
d. 3
e. 4
30) Typically, class constructors are overloaded to provide many different and convenient ways to create an object.
Select one:
a. TRUE
b. FALSE
31) When a recursive function hits its base case, it returns an actual value without calling itself anymore to determine the answer.
Select one:
a. TRUE
b. FALSE
32) A constructor always gets called when a class is asked to create an object.
Select one:
a. FALSE
b. TRUE
33) With the declarations:
int i = 10; int* myArray = new int[ i ]; the correct way to delete the variable myArray is to say: delete [] myArray;
Select one:
a. TRUE
b. FALSE
34) Given an object o, C++ programmers use the symbol -> to reference the members and methods of that object.
Select one:
a. FALSE
b. TRUE
35) In C++, cin, the thing we have been using to process input data, is actually an example of a class.
Select one:
a. TRUE
b. FALSE
36) Typically in C++, most classes have their methods marked private.
Select one:
a. FALSE
b. TRUE
37) In C++, arrays can be passed as parameters to a function either by value or by reference.
Select one:
True
False
Step by Step Solution
There are 3 Steps involved in it
Step: 1
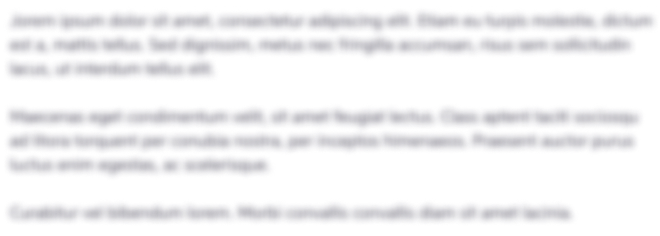
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started