Question
1. Output of the following code is: delegate int NumberChanger(int n); namespace DelegateApp { class TestDelegate { static int num = 10; public static int
1. Output of the following code is:
delegate int NumberChanger(int n);
namespace DelegateApp
{
class TestDelegate
{
static int num = 10;
public static int AddNum(int p)
{
num += p;
return num;
}
public static int MultNum(int q)
{
num *= q;
return num;
}
static void Main(string[] args)
{
//create delegate instances
NumberChanger nc;
NumberChanger nc1 = new NumberChanger(AddNum);
NumberChanger nc2 = new NumberChanger(MultNum);
nc = nc1;
nc = nc2;
//Invoke
num = nc(5);
Console.WriteLine("Value of Num: {0}", num);
Console.ReadKey();
}
}
}
Value of Num: 55 |
Value of Num: 50 |
Value of Num: 15 |
Value of Num: 75 |
2. What modifier should be used with a method if you have a class which can be used directly, but for which you want inheritors to be able to change certain behavior.
abstract |
override |
virtual |
protected |
3. When you create an abstract method, you provide __________.
curly braces |
method statements |
all of these |
the keyword abstract |
4. Abstract classes and interfaces are similar in that __________.
you can instantiate concrete objects from both |
you cannot instantiate concrete objects from either one |
all methods in both must be abstract |
neither can contain nonabstract methods |
5. When you create any derived class object, __________.
the base class and derived class constructors execute simultaneously |
the derived class constructor must execute first, then the base class constructor executes |
the base class constructor must execute first, then the derived class constructor executes |
neither the base class constructor nor the derived class constructor executes |
6. In a program that declares a derived class object, you __________ assign it to a variable of its base class type.
cannot |
can |
must |
should not |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
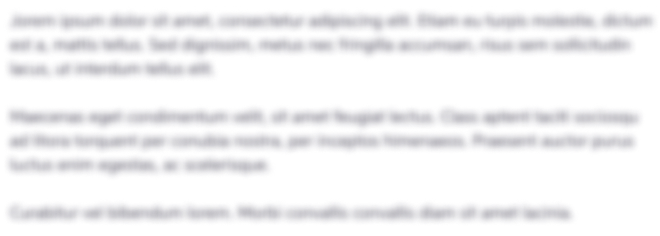
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started