Answered step by step
Verified Expert Solution
Question
1 Approved Answer
1 package Shopping; 2 3 import DataStructures.*; 4 import java.io.FileNotFoundException; 5 import java.util.ArrayList; 6 import java.util.Scanner; 7 8 /** 9 * @version Fall 2019 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
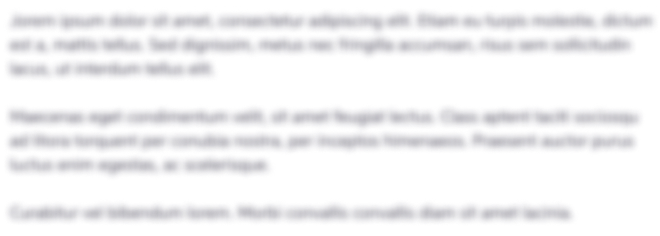
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started