Question
1. Please answer the follwing questions using Python 3. Also, please use the format below and share your code and show alll outputs. 2. If
1. Please answer the follwing questions using Python 3. Also, please use the format below and share your code and show alll outputs.
2. If there are any questions please answer them. Please use the format given below.
This assignment has you play with such things as random data generation, and computation on data stored in a Python list.
We're gonna use functions to do as much of it as we can, because functions are totally awesome.
Might I recommend the use of Numpy for random numbers and other useful things?
Problem 1. Revenue models.
You're an analyst for Farm2Table, a chain restaurant that sources its food locally whenever possible. You are tasked to forecast the financial payoff of an ad campaign.
Currently, average monthly revenue at each store is $100,000, with a standard deviation of $12,000. An advertising firm claims that with clever advertisement, they can increase monthly revenue for Farm2Table to $120,000, but the standard deviation will be increased as well, to $25,000. Assume a normal distribution.
Write a Python function simulate_revenue(average, std_dev, months).
it produces simulated revenue data according to a normal distribution with shape parameterized by average and std_dev, for a given number of months.
It returns a list of length months.
Round each item to the nearest cent. No fractions of a cent allowed.
Use simulate_revenue to generate two lists of random numbers which model potential revenue:
one list before with 24 months of revenue using the current mean and standard deviation,
another list after with 12 months of revenue using the predicted mean and standard deviation.
Then, concatenate before and after to produce a third list all_months containing the revenue of all 48 months.
Write a function print_monthly_revenue(revenue, name) that prints an arbitrary list to the screen, with these formatting requirements:
round each number when printing to the nearest $100.
do not modify the original list.
prints a two-column output, with month: revenue (the month is implicitly given). Pad the month value so it is always of width 2.
right-align the revenue value
Example: print_monthly_revenue(before, "before") produces
Revenue for period 'before' Mo: revenue ----------- 01: 123100 02: 98288 ...
Call print_monthly_revenue on each of your concatenated list, and be sure to commit the output.
Problem 2(a). Bus arrival times.
Shuttle buses arrive at an airport to fetch passengers with an average interval of 15 minutes. Their actual interarrival times follow an exponential distribution.
Write a function simulate_busses(mean, num_busses) that simulates bus arrival times
Use a random module to generate the exponentially distributed bus intervals.
Round your raw data to the nearest tenth of a minute. Realize that rounding is generally scary, and can cause serious problems downstream if not done only when appropriate.
Call your function to generate a list of 50 arrival times with mean 15; capture the result in a variable called bus_times. Print your list, and be sure to commit the output.
For example, your list might begin [11.2, 34.1, 18.8, 23.5, ....
Use Python to answer the following questions:
What is the shortest waiting time in your list?
What is the longest waiting time?
These answers must be programmatically determined and the output that proves you computed them (namely, the values) must be committed.
When answering the previous question, did you write a function? Why or why not?
Problem 2(b). Cumulative waiting times.
In this problem, you'll interpret the data you generated in Problem 2(a) as a sequence of consecutive arrival times. Suppose the first bus arrives at the measured number of minutes after midnight. The bus company wants to track the time each bus arrived, measured in minutes after midnight.
Write a function that transforms the bus arrival times into cumulative times. The function takes in a list of arrival times, and returns a list of the number of minutes after midnight that each bus arrived at, using the list you generated in part (a).
This assumes the first bus arrived at the airport terminal at midnight plus its arrival time (the time at bus_times[0]). The second arrived at the arrival time of the first plus its arrival time, etc.
I'm deliberately not naming your function for you, here. You get to choose! Make it descriptive!
With the data from our example in part (a), the answer would start [11.2, 45.3, 64.1, 87.6, ...], where 45.3 = 11.2+34.1. Call your function on your variable that you already have in memory. Print your cumulative waiting time list.
Using the list generated in 2(a), at what time does the 50th bus arrive? Print the time in the format HH:MM AM/PM where HH is the hour and MM is the minute.
On the printing of the times
Note that HH should be between 01 and 12.
You must print that leading 0 in the hour and minute, if it is less than 10.
Do not generate a new list of times; be sure to re-use the list of intervals you already generated in 2(a).
I strongly suggest you write a function to stringify the floating point number.
It eats the floating point number, interpreted as number of minutes past midnight.
It returns a string, composed of the hours, minutes, and morning/afternoon indicator.
To solve this problem, take a random number of minutes, and do the computation yourself, and write down the steps you take to do it. That's what you should make the computer do!
Test the function as you develop it, with some known inputs and the times they should map to; e.g., 125.0 is "02:05 AM", and so is 125+24*60.
Problem 3. Chocolate and the Nobel
This problem also uses generation of random numbers to simulate.
Researchers have observed a (presumably spurious) correlation between per capita chocolate consumption and the rate of Nobel prize laureates: see Chocolate Consumption, Cognitive Function, and Nobel Laureates. In this problem, we will create some sample data to simulate this relationship.
I have not told you what to name your functions, or even when to write them. But know that the person who is authoring this assignment often writes one-line functions with descriptive names. There's power in naming your actions, no matter how simple!!!
Problem 3(a). A first pass at simulation
Write Python code to produce a list of 50 ordered pairs (c,n)(c,n), where cc represents chocolate consumption in kg/year/person and nn represents the number of Nobel laureates per 10 million population, for some country. The values for cc should be random numbers (not necessarily integers!) uniformly distributed between 0 and 15. You may assume that cc and nn are related by
n=0.4c0.8n=0.4c0.8.
However, it is impossible for a nation to have a negative number of Nobel laureates, so if your predicted value of nn is less than 0 for a country, replace that value by 0.
Print your list of ordered pairs; report your values of cc and nn to 2 decimal places.
Problem 3(b). Error term.
Our list of data from part (a) is not a good simulation of real-world data, because it is perfectly linear. That is, even though the per capita chocolate variable is random, the number of Nobel laureates is 100% predicted from that value. So, we'll randomly perturb the number of laureates for each country.
Using the 50 cc and nn values you generated in part (a), generate new nene values, using the following formula:
ne=n+.ne=n+.
Here should be a random variable with normal distribution, mean 0, and standard deviation 1. Using the list of ordered pairs generated in 3(a), create a new list of 50 ordered pairs (c,ne)(c,ne). Each nn should be perturbed by a distinct and randomly generated value -- do not use the same for all nn.
Again, your simulated data should not predict negative numbers of Nobel laureates. Again, do not generate a new list of (c,n)(c,n) values; you must re-use the list of ordered pairs already generated in 3(a). Data you create as the result of evaluating a cell is available for use in other cells. Check it out -- try running the Python command who if you want to prove it to yourself (a good habit to be in!).
Print your new list of ordered pairs.
Problem 3(c). Winners and losers.
Make a new list consisting of all of the ordered pairs (c,ne)(c,ne) from your list from part (b) such that ne>0.4c0.8ne>0.4c0.8 (the nn value increased upon perturbation).
Print this new (shorter) list. Use Python to calculate how many items are in your list of winners. Hint to help you know when you have the answer correct: since the perturbation has mean 0, we expect about half to have gone up...
Problem 3(d). Preparing data to transfer to R.
For future use, split your list of ordered pairs from Problem 3(b) (not 3(c)!) into two lists.
The first list should contain the cc values, and
the second list should contain the nene values.
To actually transfer data to R, we would write it to a file, perhaps as a csv file. We'll leave that for later.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
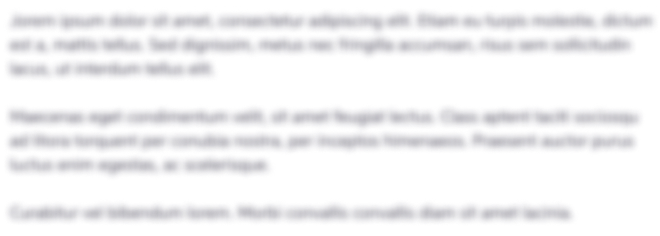
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started