Question
1. Please write a c++ program and show all outputs. SEE EXAMPLE FILES BELOW implement the VectorGraphic and Point classes. There is no need to
1. Please write a c++ program and show all outputs. SEE EXAMPLE FILES BELOW
implement the VectorGraphic and Point classes. There is no need to provide more features than those already outlined, so these classes will be fairly easy to implement. Create the classes from an XML file that defines a single VectorGraphic (use this example Vector XML file seen below). This XML file should be passed as the first parameter to your program. The second parameter should be a destination file. Parse the sourcefile and create the VectorGraphic instance, then persist that instance to the destination file; reuse as many of the tools and techniques you used for reading it in as possible.
To implement the parsing elegantly, make use of stringstreams and getline where they are convenient. You may also find you need to perform two algorithms: eating white space from a stream, and trimming string tokens. Write trim and eat functions as general tools for parsing and include them in a separate header and namespace that makes it clear they're generic, reusable, and primitive parsing tools. This collection of toolsbits of ultimately generic algorithmsoften grows throughout the life of a system, and keeping them separate from the beginning makes them easy to refer to and promotes their use as a helpful library. The eat and trim functions should be generic; that is, they should work on more than just whitespace. Their signatures should be as follows:
void trim(std::string& sourceString, std::string const& trimmables);
void eat(std::istream& sourceStream, std::string const& edibles);
Note that white space can be defined by any character in the string " \t"(a space is the last character).
Make sure you consistently use exceptions as your only means of reporting exceptional circumstances. Remember, you should only catch an exception if you are going to do something particularly useful with it; otherwise, it should propagate until it hits a boundary point (such as main). For example, an std::bad_alloc exception thrown by operator new needn't be handled necessarily by the code that invoked new, but might be best handled in main and treated as an unrecoverable failure. Make sure to report problems with malformed XML cleanly, with specific information about the offending line and the type of offense.
example Vector Graphic XML File:
closed="true"> x="0" y="0"/> x="10" y="0"> x="10" y="10"/> x="0" y="10"/>
Example Unit Tests:
example parse test:
#include "Parse.h" #include "TestHarness.h"
TEST(trimBeginning, Parse) { std::string actual{" \tHello"}; Parse::trim(actual, " \t "); CHECK_EQUAL("Hello", actual); }
TEST(trimEnd, Parse) { std::string actual{"Hello \t"}; Parse::trim(actual, " \t "); CHECK_EQUAL("Hello", actual); }
TEST(trimBeginningAndEnd, Parse) { std::string actual{" Hello \t"}; Parse::trim(actual, " \t "); CHECK_EQUAL("Hello", actual); }
TEST(trimNone, Parse) { std::string actual{"Hello"}; Parse::trim(actual); CHECK_EQUAL("Hello", actual); }
TEST(trimEmpty, Parse) { std::string actual; Parse::trim(actual); CHECK_EQUAL("", actual); }
TEST(trimEverything, Parse) { std::string actual{"Hello 1234"}; std::string trimmables{"Hello 0123456789"}; Parse::trim(actual, trimmables); CHECK_EQUAL("", actual); }
TEST(eatNothing, Parse) { std::istringstream stream{"Hello"}; Parse::eat(stream, "123456789"); std::ostringstream actual; actual << stream.rdbuf(); CHECK_EQUAL("Hello", actual.str()); }
TEST(eatSomething, Parse) { std::istringstream stream{"4320Hello"}; Parse::eat(stream, "1234567890"); std::ostringstream actual; actual << stream.rdbuf(); CHECK_EQUAL("Hello", actual.str()); }
TEST(eatSomeLeadingWhitespace, Parse) { std::istringstream stream{" I had leading whitespace"}; Parse::eat(stream); std::ostringstream actual; actual << stream.rdbuf(); CHECK_EQUAL("I had leading whitespace", actual.str()); }
example point test:
// // PointTest.cpp // Assignment1 // // Created by Chris Elderkin on 4/26/15. // Copyright (c) 2015 Chris Elderkin. All rights reserved. //
#include "Point.h" #include "TestHarness.h"
TEST(equality, Point) { CHECK_EQUAL(VG::Point(1, 2), VG::Point(1, 2)); }
TEST(inequality, Point) { CHECK(VG::Point(1, 2) != VG::Point(3, 4)); }
TEST(constexprPoint, Point) { constexpr int i = VG::Point{4, 5}.getX(); CHECK_EQUAL(i, 4); }
example vector graphic test:
#include "Parse.h" #include "VectorGraphic.h" #include "VectorGraphicStreamer.h" #include "TestHarness.h"
TEST(ctor, VectorGraphic) { VG::VectorGraphic vg; CHECK_EQUAL(0, vg.getPointCount()); CHECK_EQUAL(true, vg.isClosed()); CHECK_EQUAL(false, vg.isOpen()); }
TEST(insertPoint, VectorGraphic) { VG::VectorGraphic vg; vg.addPoint(VG::Point{1, 1}); CHECK_EQUAL(1, vg.getPointCount()); vg.addPoint(VG::Point{2, 2}); CHECK_EQUAL(2, vg.getPointCount()); }
TEST(removePoint, VectorGraphic) { VG::VectorGraphic vg; vg.addPoint(VG::Point{1, 1}); vg.addPoint(VG::Point{2, 2}); vg.removePoint(VG::Point{1, 1}); CHECK_EQUAL(1, vg.getPointCount()); CHECK_EQUAL(VG::Point(2, 2), vg.getPoint(0)); }
TEST(erasePoint, VectorGraphic) { VG::VectorGraphic vg; vg.addPoint(VG::Point{1, 1}); vg.addPoint(VG::Point{2, 2}); vg.addPoint(VG::Point{3, 3}); vg.erasePoint(1); CHECK_EQUAL(2, vg.getPointCount()); CHECK_EQUAL(VG::Point(1, 1), vg.getPoint(0)); CHECK_EQUAL(VG::Point(3, 3), vg.getPoint(1)); }
TEST(erasePointOutOfRange, VectorGraphic) { VG::VectorGraphic vg; vg.addPoint(VG::Point{1, 1}); vg.addPoint(VG::Point{2, 2}); vg.addPoint(VG::Point{3, 3}); try { vg.erasePoint(5); } catch (std::out_of_range&) { CHECK_EQUAL(3, vg.getPointCount()); return; } CHECK(false); // should have caught exception }
TEST(equality, VectorGraphic) { VG::VectorGraphic vg1; vg1.addPoint(VG::Point{1, 1}); vg1.addPoint(VG::Point{2, 2}); vg1.addPoint(VG::Point{3, 3}); VG::VectorGraphic vg2; vg2.addPoint(VG::Point{1, 1}); vg2.addPoint(VG::Point{2, 2}); vg2.addPoint(VG::Point{3, 3}); CHECK(vg1 == vg2); }
TEST(inequality, VectorGraphic) { VG::VectorGraphic vg1; vg1.addPoint(VG::Point{1, 1}); vg1.addPoint(VG::Point{1, 2}); vg1.addPoint(VG::Point{1, 3}); VG::VectorGraphic vg2; vg2.addPoint(VG::Point{2, 1}); vg2.addPoint(VG::Point{2, 2}); vg2.addPoint(VG::Point{2, 3}); CHECK(vg1 != vg2); VG::VectorGraphic vg3; vg3.addPoint(VG::Point{1, 1}); vg3.addPoint(VG::Point{1, 2}); vg3.addPoint(VG::Point{1, 3}); vg3.openShape(); CHECK(vg3 != vg1); }
TEST(closeShape, VectorGraphic) { VG::VectorGraphic vg; vg.closeShape(); CHECK_EQUAL(true, vg.isClosed()); CHECK_EQUAL(false, vg.isOpen()); }
TEST(openShape, VectorGraphic) { VG::VectorGraphic vg; vg.openShape(); CHECK_EQUAL(false, vg.isClosed()); CHECK_EQUAL(true, vg.isOpen()); }
TEST(widthHeight, VectorGraphic) { VG::VectorGraphic vectorGraphic; vectorGraphic.addPoint(VG::Point{0, 2}); vectorGraphic.addPoint(VG::Point{4, 3}); vectorGraphic.addPoint(VG::Point{5, 8}); vectorGraphic.addPoint(VG::Point{2, 1}); CHECK_EQUAL(5, vectorGraphic.getWidth()); CHECK_EQUAL(7, vectorGraphic.getHeight()); vectorGraphic.erasePoint(2); CHECK_EQUAL(4, vectorGraphic.getWidth()); CHECK_EQUAL(2, vectorGraphic.getHeight()); }
// C++11 has a new "raw string literal" that is useful for // embedding long strings in a file for testing. Previously // this would have to be done with a "stringification" macro: // #define STR(a) #a
const std::string VectorGraphicXml = R"(
TEST(fromXml, VectorGraphic) { std::stringstream sstr(VectorGraphicXml); auto vg = VG::VectorGraphicStreamer::fromXml(sstr); CHECK_EQUAL(true, vg.isClosed()); CHECK_EQUAL(4, vg.getPointCount()); CHECK_EQUAL(10, vg.getPoint(2).getX()); CHECK_EQUAL(10, vg.getPoint(2).getY()); }
TEST(toXml, VectorGraphic) { VG::VectorGraphic vg1; vg1.addPoint(VG::Point(1, 1)); vg1.addPoint(VG::Point(2, 2)); vg1.addPoint(VG::Point(3, 3)); std::stringstream sstr; VG::VectorGraphicStreamer::toXml(vg1, sstr); auto vg2 = VG::VectorGraphicStreamer::fromXml(sstr); CHECK(vg1 == vg2); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
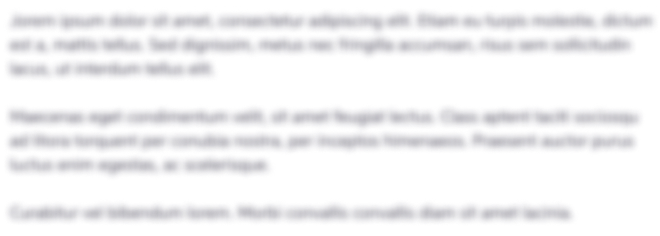
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started