Question
1 . Priority Encoder Implement the priority encoder in priEnc.sv 2. Population Count Implement the popCount unit in popCount.sv 3. Argument Maximum Implement the argMax
1 . Priority Encoder Implement the priority encoder in priEnc.sv 2. Population Count Implement the popCount unit in popCount.sv
3. Argument Maximum Implement the argMax unit in argMax.sv
Here is the code for priEnc.sv please complete:
module priEnc
#( bW = 8 )
(
input logic [bW-1:0] a,
output logic [bW-1:0] z
);
// Implement an 8bit priority encoder
// z should be a one hot bit string were the
// hot bit is equivelant to the position
// of the most significant 1
// for example:
//
// 01011100 --> 01000000
// 01011011 --> 01000000
// 10001001 --> 10000000
// 01001001 --> 01000000
// 11010000 --> 10000000
// 11010111 --> 10000000
// 01010001 --> 01000000
// 10010110 --> 10000000
// 00001100 --> 00001000
// 11000010 --> 10000000
// 11001000 --> 10000000
// 01110111 --> 01000000
// 00111101 --> 00100000
// 00010010 --> 00010000
// 01111110 --> 01000000
// 01101101 --> 01000000
// 00111001 --> 00100000
// 00011111 --> 00010000
// 11010011 --> 10000000
// 10000101 --> 10000000
//
// If you are interested in exploring this implementation thoroughly
// you might try to implement it using the ripple-carry design pattern
endmodule
Here is the code for popCount.sv please complete:
module popCount
#( bW = 32, aW = 6 )
(
input logic [bW-1:0] a,
output logic [aW-1:0] z
);
// Implement the popCount for a
// for example:
/*
01000010011010010001101100010101 --> 13
11001100000001001000000100101011 --> 11
10101000100100111001111100011010 --> 16
00100010110111110111011001011101 --> 19
00010010010011010101000001101011 --> 13
00010010000010001001000010000000 --> 6
00010101111000100010011001100110 --> 14
00011001000000000100000000001010 --> 6
00000100000000000010000001000000 --> 3
00000000000000001000000000000000 --> 1
11000000001000000010000001000001 --> 6
00010100000001001001001000001000 --> 7
10111011101100110101000010000011 --> 16
01000110000010000011101111010101 --> 14
10101011100001000010000100001101 --> 12
10010101001111110111011110011000 --> 19
00000111010101001000001100001001 --> 11
00000010011100000001100111000000 --> 9
01010110111010110101000011110111 --> 19
00100111110000001110011000001100 --> 13
01101110001001110100000000001011 --> 13
00000110001000100011010000111001 --> 11
00101000001010001000000000001010 --> 7
*/
// If you are interested in exploring this thoroughly,
// you might try to implement this using the full adder cell
endmodule
Here is the code for argMax please complete:
module argMaxTwo
#( bW=16, aW=3 )
(
output logic [bW-1:0] mVal ,
output logic [aW-1:0] mIdx ,
input logic [bW-1:0] val[1:0],
input logic [aW-1:0] idx[1:0]
);
// You might use this module to build your tree
// mval and mIdx should correxpond to the largest val idx for the largest v\
al
endmodule
module argMax
#( bW=16, aW=3 )
(
input logic [bW-1:0] A[7:0] ,
output logic [aW-1:0] idx
);
// Return the index of the largest value in the array
// such that A[idx] should be greater than equal to A[i] for all i
// for example:
/*
61708 31289 37634 48661 63749 17230 10498 20920 --> 4
45576 21389 36527 12776 2448 44021 36940 48507 --> 7
42560 805 46605 4208 63789 55337 20924 2096 --> 4
11712 26960 27297 16205 12021 45045 52317 4756 --> 6
42602 26044 2232 40094 1786 2886 25607 48275 --> 7
29781 56247 61765 2659 57433 52774 30312 58398 --> 2
62608 42330 34076 26553 8107 55760 25967 28120 --> 0
34885 42893 9099 37372 8156 2724 36121 13221 --> 1
36414 49395 11352 27588 17244 17670 20634 6424 --> 1
26309 53674 794 54723 59826 25356 3817 64963 --> 7
29675 47608 1010 44425 29551 19227 10413 49512 --> 7
4645 22479 36267 53787 16000 55645 64898 16900 --> 6
42327 35638 818 10361 44772 60438 60586 26048 --> 6
34862 14220 49777 36859 8636 26844 22295 1217 --> 2
8683 15285 11913 57999 63167 10928 39635 726 --> 4
52354 29978 42865 1239 36366 5361 7629 17157 --> 0
60188 51096 35052 54826 19045 1335 13827 40702 --> 0
56573 3646 13544 14452 35248 56326 19730 64616 --> 7
197 12030 3382 2547 44027 31851 48919 13839 --> 6
46612 1322 28326 9940 4299 49771 60164 13044 --> 6
24973 28897 48517 29881 5833 36448 12870 52028 --> 7
24375 21848 22387 53774 9180 49968 62928 58624 --> 6
3907 37368 39585 8019 36760 15711 37511 23290 --> 2
53549 624 59499 14385 49170 57100 27405 4885 --> 2
1856 36244 41812 640 5395 34499 57069 59100 --> 7
4216 3367 9823 23331 60331 5944 19746 48585 --> 4
19655 25218 55246 8903 42020 28635 5128 28533 --> 2
7605 29210 4920 14978 41048 27216 39587 58276 --> 7
57189 6122 14598 32852 16475 61640 14664 62007 --> 7
62456 12981 43345 54659 58267 56629 21424 33531 --> 0
*/
// If you are interested in exploring this implementation thoroughly
// you might try to implement it as a reduction tree
logic [bW-1:0] mVal_0[3:0];
logic [aW-1:0] mIdx_0[3:0];
argMaxTwo am_0_0(mVal_0[0],mIdx_0[0], A[1:0], '{3'd1,3'd0} );
endmodule // end maxSix
Step by Step Solution
There are 3 Steps involved in it
Step: 1
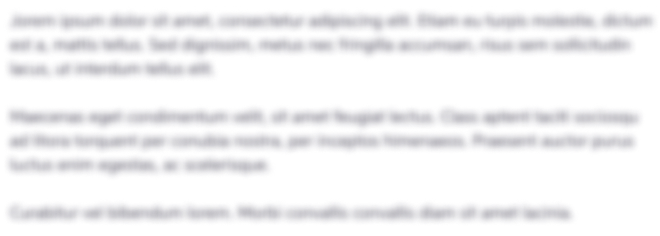
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started