Question
1 ) public class ShoppingCenter{ /**This is the instance variable we will use to store the user's balance. It is the only instance variable we
1 )
public class ShoppingCenter{
/**This is the instance variable we will use to store the user's balance. It is
the only instance variable we will need.
**/
private double userBalance;
/**This is the constructor. We will need to give the initial balance to the user.
We're running a special promotion where new users start with $100 of store credit.
**/
public ShoppingCenter(){
}
/**In this method we will return the balance of the user. This method takes no parameters
and returns a double representing the balance of the user.
**/
public double getUserBalance(){
return -1;
}
/**In this method we handle the case where the user wants to deposit money into their account.
We take in the value they want to add as the double amountToAdd and return a boolean value
signifying if the deposit was successful or not. We want to make sure that amountToAdd is
greater than zero. If it is, we want to add the value to the account and return true. However
if it is not, we don't want to do anything and instead return false.
**/
public boolean addToUserBalance(double amountToAdd){
return false;
}
/**This method allows the user to purchase T-Shirts. It takes two parameters: amount, which represents
the number of T-shirts being purchased; and size, which represents the size of the shirts being purchased.
The prices for T-Shirts are as follows:
smalls (represented by the character 's' or 'S'): $10 each
medium (represented by the character 'm' or 'M'): $20 each
larges (represented by the character 'l' or 'L'): $24 each
Before allowing the purchase to go through, make sure it is a valid purchase first. Purchases can be invalid
for a number of reasons. If the user does not have enough money, it is invalid. If the size character is invalid,
the purchase is invalid. If they try to buy zero or a negative number of shirts, it is invalid.
If a purchase is invalid, we will return false so that the error message can be shown by the main method. Otherwise
we will subtract the cost from the user's balance and return true to signify that it is a valid purchase.
**/
public boolean purchaseTShirts(int numTShirts, char size){
return false;
}
}
2 )
import java.util.*;
public class ShoppingCenterMain{
public static void main(String[] args){
Scanner scan = new Scanner(System.in);
int choice = -1;
ShoppingCenter shop = new ShoppingCenter(); //Make our shopping center
while(choice != 4){
System.out.println("Welcome to the UMass Shopping Center! What would you like to do?");
System.out.println("\t1) Check Balance \t2) Deposit Money \t3) Purchace T-Shirts \t4) Quit");
choice = Integer.parseInt(scan.nextLine());
if(choice == 1){ //User wants to get balance
System.out.println("Your balance is $" + shop.getUserBalance());
} else if (choice == 2){ //User wants to add to balance
System.out.println("How much would you like to deposit?");
double amount = Double.parseDouble(scan.nextLine());
if(shop.addToUserBalance(amount)){ //Is a valid amount
System.out.println("Deposit succeeded! Your balance is $" + shop.getUserBalance());
} else { //Is not a valid amount
System.out.println("Deposit failed, try another amount");
}
} else if (choice == 3){ //User wants to purchace T-Shirts
System.out.println("How many T-Shirts would you like to buy?");
int shirts = Integer.parseInt(scan.nextLine()); //get count
System.out.println("What size would you like them to be? \tsmall ($10 each) \tmedium ($20 each) \tlarge ($24 each)");
char size = scan.nextLine().charAt(0); //get size
if(shop.purchaseTShirts(shirts, size)){ //Successful purchase
System.out.println("Thank you for your purchase. Your new balance is $" + shop.getUserBalance());
} else { //User doesn't have enough
System.out.println("We're sorry, we were unable to complete your purchase at this time. Please try again later");
}
}
}
System.out.println("See you next time!");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
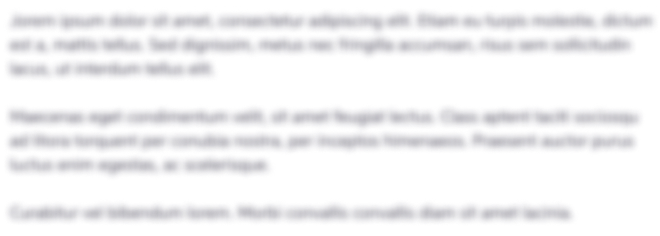
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started