Question
1. Re-implement the same calculations for area and volume in A3 in 3 new functions and not in the main function. We will not re-implement
1. Re-implement the same calculations for area and volume in A3 in 3 new functions and not in the main function. We will not re-implement calculating the volume of a sphere like in A3. The new functions takes parameters such as radius and length (length for the dimensions of a cube should be twice the radius):
double calcAreaCircle(int radius)
double calcVolCylinder(int radius, int height)
double calcVolCube (int length)
2. In the main function, call your new functions with different hard coded inputs (and a printf statement to see what they return). You will comment out this code (dont delete) when you are confident all 3 functions work as expected.
Example: double circleArea1 = calcAreaCircle(0);
printf(circleArea1 with radius %i has area %f , 0, circleArea1);
3. In the main function, ask for user input to get the radius, height and length values (use scanf or scanf_s) so you can pass them in the three calculation functions when calling them.
4. In the main function, call the three functions to calculate the circle area, cylinder volume, and cube volume for the input radius or length.
5. In the main function, print out meaningful information (use printf or printf_s) to indicate the circle area, cylinder volume, and cube volume.
Notice that:
We will need MY_PI. Since MY_PI is a constant, you can make this a global constant. This means you put it by itself outside of any function (put it before main). We did this in A3.
o Rhetorical question (i.e. dont write an answer): why am I allowed to use global constants but global variables are a big no-no?
Your function calcVolCylinder must use/call function calcAreaCircle() instead of you copying and pasting code from the other function. In other words, you need a call to calcAreaCircle inside the body of calcVolCylinder.
The three functions you implement should NOT have a printf statement in them nor do they take user input. Data goes into the function and data is passed out. The scanf or printf statements should only be used in the main function to take user input or show results.
You should test your code with a variety of values. These test values can be hard coded (write the number directly instead of asking the user) for testing and then commented out when you submit your code.
Task 2: Absolute Value
The absolute value of a number is the magnitude of the number. or more simply put, the always positive version of a number. |4.2| == 4.2 and |-4.2| == 4.2.
Write a function myAbs that takes a variable named origVal as the input parameter and outputs a double that is always >= 0.0. The data type of origVal should be double.
You are NOT allowed to use the math librarys absolute value function in your myAbs function. *HINT* You can use other math functions like sqrt (square root) to calculate the absolute value. Also, what is -22 ? How about 22 ?
In the main function, call your function myAbs and test it with different numbers and print out the testing results (no need to get user input unless you want to show that). These inputs should test that your function works.
Test the SAME inputs with the math librarys absolute value function: abs(x). Rhetorical question: Are the values returned the same? Almost the same?
Task 3: Round
Without using the math function round, write a function called simpleRound that takes a double as input and returns an integer as output. You should be able to do this purely with math (no if statements). You only need to test positive doubles (but please feel free to show off that your function works with negative values as well.no points but you get extra bragging rights).
In our case, any decimal number X.Y (like 12.325) will be rounded up to X+1 if Y is >= 5. Values will be rounded down to X if Y < 5. I think some examples will make it more obvious:
Eg. simpleRound(4.6) returns 5,
simpleRound(6.4) returns 6, and
simpleRound(5.5) returns 6 (just to keep things simple .5 just rounds up).
Hint: What happens if I double 4.6 and then make it an integer? What about 6.4*2 as an integer??
Remember that you can make a double an integer by casting: int x = (int) 2.6; will set x to be 2. Make sure you think through the problem a bit before you code.
What happens if 2*x is an odd number? For example, (int)(5.6*2) = 11. What I really want is to get the number 6 in the end, not the number 5 yet 11/2 = 5 (integer math).
o Can I use the remainder (%) somehow to make the value 6 instead of 5?
o Again, play with some numbers: 0.2, 0.5 and 1.7 should return 0, 1 and 2.
Make sure you call simpleRound with different test values (at least 3 different tests) and printf what the results are (in the main method, not in the function).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
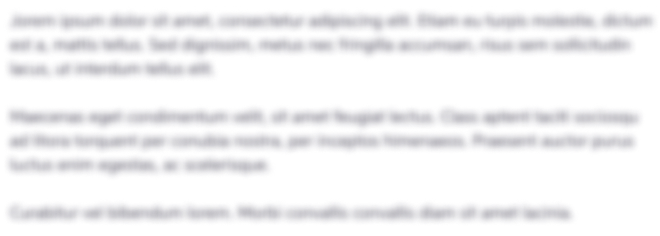
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started