Question
1. Test class DiskSpace for several exemplar folders import java.util.Scanner; import java.io.File; public class DiskSpace { /** * Calculates the total disk usage (in bytes)
1. Test class DiskSpace for several exemplar folders
import java.util.Scanner; import java.io.File;
public class DiskSpace {
/** * Calculates the total disk usage (in bytes) of the portion of the file system rooted * at the given path, while printing a summary akin to the standard 'du' Unix tool. * @param root designates a location in the file system * @return total number of bytes used by the designated portion of the file system */ public static long diskUsage(File root) { long total = root.length(); // start with direct disk usage if (root.isDirectory()) { // and if this is a directory, for (String childname : root.list()) { // then for each child File child = new File(root, childname); // compose full path to child total += diskUsage(child); // add child's usage to total } } System.out.println(total + "\t" + root); // descriptive output return total; // return the grand total }
/** * Computes disk usage of the path given as a command line argument. * Sample usage: java DiskSpace C:\Users\Michael */ public static void main(String[] args) { String start; if (args.length > 0) { start = args[0]; } else { System.out.print("Enter the starting location: "); start = new Scanner(System.in).next(); } diskUsage(new File(start)); }
}
2.Test class BinarySearch and make necessary changes on it so that it will print some outputs based on different data.
public class BinarySearch {
/** * Returns true if the target value is found in the indicated portion of the data array. * This search only considers the array portion from data[low] to data[high] inclusive. */ public static boolean binarySearch(int[] data, int target, int low, int high) { if (low > high) return false; // interval empty; no match else { int mid = (low + high) / 2; if (target == data[mid]) return true; // found a match else if (target < data[mid]) return binarySearch(data, target, low, mid - 1); // recur left of the middle else return binarySearch(data, target, mid + 1, high); // recur right of the middle } }
// Demonstration of a public wrapper function with cleaner signature /** Returns true if the target value is found in the data array. */ public static boolean binarySearch(int[] data, int target) { return binarySearch(data, target, 0, data.length - 1); // use parameterized version }
/** Returns true if the target value is found in the data array. */ public static boolean binarySearchIterative(int[] data, int target) { int low = 0; int high = data.length - 1; while (low <= high) { int mid = (low + high) / 2; if (target == data[mid]) // found a match return true; else if (target < data[mid]) high = mid - 1; // only consider values left of mid else low = mid + 1; // only consider values right of mid } return false; // loop ended without success }
/** Simple test, assuming valid integer given as command-line argument */ public static void main(String[] args) { final int N = 100; int[] data = new int[N]; for (int j=0; j < N; j++) data[j] = 1 + 2*j;
for (int j=0; j < N; j++) { if (!binarySearch(data, 1 + 2*j)) System.out.println("Recursive failure to find value " + (1+2*j)); if (!binarySearchIterative(data, 1 + 2*j)) System.out.println("Iterative failure to find value " + (1+2*j)); }
for (int j=0; j <= N; j++) { if (binarySearch(data, 2*j)) System.out.println("Recursive false-positive on value " + (2*j)); if (binarySearchIterative(data, 2*j)) System.out.println("Iterative false-positive on value " + (2*j)); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
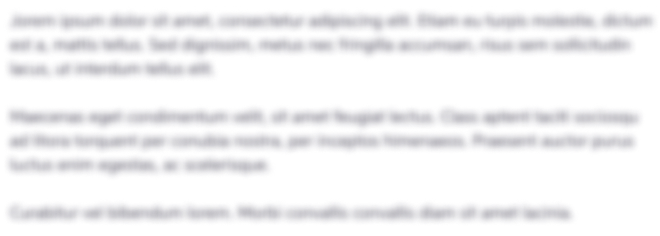
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started