Question
1. The enhanced for loop: Java provides a specialized version of the for loop that, in many circumstances, simplifies array processing. It is known as
1. The enhanced for loop: Java provides a specialized version of the for loop that, in many circumstances, simplifies array processing. It is known as enhanced for loop. General format: for(dataType elementVariable : array) Statements; The enhanced for loop is designed to iterate once for every element in an array. Each time the loop iterates, it copies an array element to a variable. dataType elementVariable: a variable declaration. This variable will receive the value of a different array element during each loop iteration. During the first loop iteration, it receives the value of the first element; during the second iteration, it receives the value of the second element, and so on. This variable must be of the same data type as the array elements, or a type that the elements can automatically be converted to. array: the name of an array that you wish the loop to operate on. The loop will iterate once for every element in the array. Now, open textpad or sublimeText2, and write the following program.
public class enhancedFor { public static void main(String[] args) { int[] numbers = {3, 6, 9}; for(int val : numbers) { System.out.println(val); } } } Above program works as follows: The numbers array has three elements. Thus, the loop will iterate three times. The first time it iterates, the val variable will receive the value in numbers[0]. The second iteration, val will receive the value in numbers[1]. The third iteration, val will receive the value in numbers[2]. The codes output will be as follows: 3 6 9 Benefit of enhanced for loop: Enhanced for loop is simpler to use than the traditional for loop. With the enhanced for loop, you do not have to be concerned about the size of the array. You do not have to create an index variable to hold subscripts. Circumstances that do not allow using enhanced for loop: WHEN You need to change the contents of an array element. You need to work through the array elements in reverse order. You need to access some of the array elements, but not all of them. You need to simultaneously work with two or more arrays within the loop. You need to refer to the subscript number of a particular element. 2. Copying Arrays: When you wish to copy the contents of one array to another, you might be tempted to write something like the following code. int[] array1 = {2, 4, 6, 8, 10}; int[] array2 = array1; The first statement creates an array and assigns its address to the array1 variable. The second statement assigns array1 to array2. THIS DOES NOT MAKE A COPY OF THE ARRAY REFERENCE BY ARRAY1. Rather, it makes a copy of the address stored in array1 and stores it in array2. This is called reference copy. This illustrate with following figure. Following code demonstrate reference copy. Open textpad or sublimeText2 and write the following program. public class referenceCopy { public static void main(String[] args) { int[] array1 = {2, 4, 6, 8, 10}; int[] array2 = array1; //change one of the elements using array1 array1[0] = 200; //change of of the elements using array2 array2[4] = 1000; //display all of the elements using array1 System.out.println("The contents of array1:"); for(int value : array1) System.out.print(value + " "); System.out.println(); //display all of the elements using array2 System.out.println("The contents of array2:"); for(int value : array2) System.out.print(value + " "); System.out.println(); } } address 2 4 6 8 10 An int array address The array1 variable holds the address of an int array. The array2 variable holds the address of an int array. The output of above program will be as follows: The contents of array1: 200 4 6 8 1000 The contents of array2: 200 4 6 8 1000 The output of the program illustrates that you can not copy an array by assigning one array reference variable to another. In order to copy array elements to another array, you must copy the individual elements of one array to another. Usually, this is the best done with a loop. int ARRAY_SIZE = 5; int[] firstArray = {5, 10, 15, 20, 25}; int[] secondArray = new int[ARRAY_SIZE]; for(int index = 0; index < firstArray.length; index++) secondArray[index] = firstArray[index]; The loop in this code copies each element of firstArray to the corresponding element of secondArray. Following code demonstrate copy array to another. Open textpad or sublimeText2 and write the following program. public class copyArray { public static void main(String[] args) { int ARRAY_SIZE = 5; int[] array1 = {2, 4, 6, 8, 10}; int[] array2 = new int[ARRAY_SIZE]; //make array2 references a copy of array1 for(int index = 0; index < array1.length; index++) array2[index] = array1[index]; //change one of the elements using array1 array1[0] = 200; //change of of the elements using array2 array2[4] = 1000; //display all of the elements using array1 System.out.println("The contents of array1:"); for(int value : array1) System.out.print(value + " "); System.out.println(); //display all of the elements using array2 System.out.println("The contents of array2:"); for(int value : array2) System.out.print(value + " "); System.out.println(); } } The output of above program will be as follows: The contents of array1: 200 4 6 8 10 The contents of array2: 2 4 6 8 1000 The result of the program demonstrates that array1 and array2 are independent arrays. please put comments
Step by Step Solution
There are 3 Steps involved in it
Step: 1
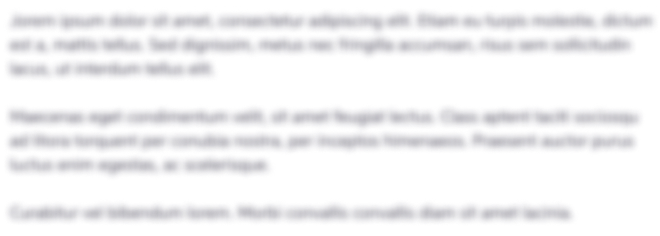
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started