Answered step by step
Verified Expert Solution
Question
1 Approved Answer
1 . Theoretical Analysis: Determine the asymptotic time and space complexities of both algorithms: To determine the asymptotic time and space complexities of both algorithms,
Theoretical Analysis: Determine the asymptotic time and space complexities of both algorithms: To determine the asymptotic time and space complexities of both algorithms, let's analyze each algorithm separately: Naive Algorithm: Time Complexity: The naive algorithm has a time complexity of On where n is the number of people in the queue. This is because for each person, there is a nested loop that iterates over all previous people to decide which ATM to assign them to Space Complexity: The space complexity of the naive algorithm is On where n is the number of people in the queue. This is because it only requires space for the queues representing the two ATMs. Optimized Algorithm: Time Complexity: The optimized algorithm has a time complexity of On where n is the number of people in the queue. This is because it iterates through the list of people once, assigning each person to the ATM with the shorter total transaction time. Space Complexity: The space complexity of the optimized algorithm is On similar to the naive algorithm, as it also requires space for the queues representing the two ATMs. In summary: Naive Algorithm: Time Complexity: On Space Complexity: On Optimized Algorithm: Time Complexity: On Space Complexity: On These complexities represent the theoretical performance of each algorithm as the input size number of people grows. I did point now you do the point and : Empirical Analysis: Test both algorithms with various input sizes and document performance: Results Comparison: Compare theoretical and empirical results, and explain any discrepancies This are two codes : Naive algorithm : #include #include #include struct Person int id; int transactionTime; in minutes ; void naiveATMQueuestd::queue& ATM std::queue& ATM const std::vector& people for int i ; i people.size; i bool assignToATM true; Default assignment to ATM Recalculate assignment for each person up to the current person for int j ; j i; j if j assignToATM true; else assignToATM false; if assignToATM ATMpushpeoplei; std::cout "Person peopleiid assigned to ATM with transaction time peopleitransactionTime minutes. ; else ATMpushpeoplei; std::cout "Person peopleiid assigned to ATM with transaction time peopleitransactionTime minutes. ; int main std::queue ATM ATM; std::vector people ; naiveATMQueueATM ATM people; return ; #include #include struct Person int id; int transactionTime; in minutes ; Optimized algorithm: void optimizedATMQueuestd::queue& ATM std::queue& ATM const std::vector& people int ATMtime ATMtime ; Total transaction times for each ATM for const auto& person : people if ATMtime ATMtime ATMpushperson; ATMtime person.transactionTime; std::cout "Person person.id assigned to ATM Total time now: ATMtime minutes. ; else ATMpushperson; ATMtime person.transactionTime; std::cout "Person person.id assigned to ATM Total time now: ATMtime minutes. ; int main std::queue ATM ATM; std::vector people ; optimizedATMQueueATM ATM people; return ;
Theoretical Analysis: Determine the asymptotic time and space complexities of both
algorithms:
To determine the asymptotic time and space complexities of both algorithms, let's analyze each algorithm separately:
Naive Algorithm:
Time Complexity:
The naive algorithm has a time complexity of On where n is the number of people in the queue. This is because for each person, there is a nested loop that iterates over all previous people to decide which ATM to assign them to
Space Complexity:
The space complexity of the naive algorithm is On where n is the number of people in the queue. This is because it only requires space for the queues representing the two ATMs.
Optimized Algorithm: Time Complexity:
The optimized algorithm has a time complexity of On where n is the number of people in the queue. This is because it iterates through the list of people once, assigning each person to the ATM with the shorter total transaction time.
Space Complexity:
The space complexity of the optimized algorithm is On similar to the naive algorithm, as it also requires space for the queues representing the two ATMs.
In summary:
Naive Algorithm:
Time Complexity: On
Space Complexity: On Optimized Algorithm:
Time Complexity: On
Space Complexity: On
These complexities represent the theoretical performance of each algorithm as the input size number of people grows.
I did point now you do the point and :
Empirical Analysis: Test both algorithms with various input sizes and document
performance:
Results Comparison: Compare theoretical and empirical results, and explain any
discrepancies
This are two codes :
Naive algorithm :
#include
#include
#include
struct Person
int id;
int transactionTime; in minutes
;
void naiveATMQueuestd::queue& ATM std::queue& ATM const std::vector& people
for int i ; i people.size; i
bool assignToATM true; Default assignment to ATM
Recalculate assignment for each person up to the current person
for int j ; j i; j
if j
assignToATM true;
else
assignToATM false;
if assignToATM
ATMpushpeoplei;
std::cout "Person peopleiid assigned to ATM with transaction time
peopleitransactionTime minutes.
;
else
ATMpushpeoplei;
std::cout "Person peopleiid assigned to ATM with transaction time
peopleitransactionTime minutes.
;
int main
std::queue ATM ATM;
std::vector people ;
naiveATMQueueATM ATM people;
return ;
#include
#include
struct Person
int id;
int transactionTime; in minutes
;
Optimized algorithm:
void optimizedATMQueuestd::queue& ATM std::queue& ATM const std::vector& people
int ATMtime ATMtime ; Total transaction times for each ATM
for const auto& person : people
if ATMtime ATMtime
ATMpushperson;
ATMtime person.transactionTime;
std::cout "Person person.id assigned to ATM Total time now: ATMtime minutes.
;
else
ATMpushperson;
ATMtime person.transactionTime;
std::cout "Person person.id assigned to ATM Total time now: ATMtime minutes.
;
int main
std::queue ATM ATM;
std::vector people ;
optimizedATMQueueATM ATM people;
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
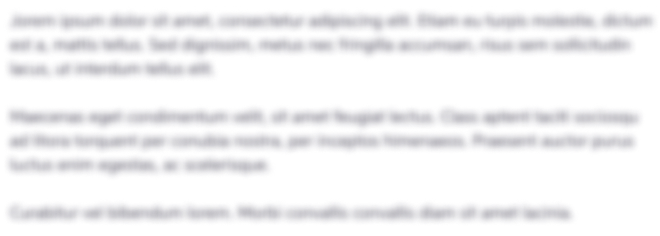
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started