Question
1. // This program takes two values from the user and then swaps them // before printing the values. The user will be prompted to
1.
// This program takes two values from the user and then swaps them
// before printing the values. The user will be prompted to enter
// both numbers.
#include
using namespace std;
int main()
{
float firstNumber;
float secondNumber;
// Prompt user to enter the first number.
cout << "Enter the first number" << endl;
cout << "Then hit enter" << endl;
cin >> firstNumber;
// Prompt user to enter the second number.
cout << "Enter the second number" << endl;
cout << "Then hit enter" << endl;
cin >> secondNumber;
// Echo print the input.
cout << endl << "You input the numbers as " << firstNumber
<< " and " << secondNumber << endl;
// Now we will swap the values.
firstNumber = secondNumber;
secondNumber = firstNumber;
// Output the values.
cout << "After swapping, the values of the two numbers are "
<< firstNumber << " and " << secondNumber << endl;
return 0;
}
2.
// This is the first program that just writes out a simple message
#include
using namespace std;
int main()
{
cout << "Now is the time for all good men" << endl;
cout << "To come to the aid of their party" << endl;
return 0;
}
3.
// This program demonstrates a compile error.
#include
using namespace std;
int main()
{
int number;
float total;
cout << "Today is a great day for Lab"
cout << endl << "Let's start off by typing a number of your choice" << endl;
cin >> number;
total = number * 2;
cout << total << " is twice the number you typed" << endl;
return 0;
}
4.
// This program will take a number and divide it by 2.
#include
using namespace std;
int main()
{
float number;
int divider;
divider = 0;
cout << "Hi there" << endl;
cout << "Please input a number and then hit return" << endl;
cin >> number;
number = number / divider;
cout << "Half of your number is " << number << endl;
return 0;
}
5.
- Both main memory and secondary storage are types of memory. Describe the differ-ence between the two.
- What is the difference between system software and application software? 3. What type of software controls the internal operations of the computer's hardware? 4. Why must programs written in a high-level language be translated into machine lan-guage before they can be run?
- 5. Why is it easier to write a program in a high-level language than in machine language? 6. Explain the difference between an object file and an executable file. 7. What is the difference between a syntax error and a logical error?
- Fill-in-the-Blank 8. Computers can do many different jobs because they can be _________. 9. The job of the _________ is to fetch instructions, carry out the operations commanded by the instructions, and produce some outcome or resultant information.
- 10. Internally, the CPU consists of the _________ and the _________. 11. A(n) _________ is an example of a secondary storage device. 12. The two general categories of software are _________ and _________. 13. A program is a set of _________. 14. Since computers can't be programmed in natural human language, algorithms must be written in a(n) _________ language.
- 15. _________ is the only language computers really process. 16. _________ languages are close to the level of humans in terms of readability. 17. _________ languages are close to the level of the computer. 18. A program's ability to run on several different types of computer systems is called _________.
- 19. Words that have special meaning in a programming language are called _________. 20. Words or names defined by the programmer are called _________. 21. _________ are characters or symbols that perform operations on one or more operands. 22. __________ characters or symbols mark the beginning or end of programming state-ments, or separate items in a list.
- 23. The rules that must be followed when constructing a program are called __________. 24. A(n) __________ is a named storage location. 25. A variable must be __________ before it can be used in a program. 26. The three primary activities of a program are __________, __________, and __________.
- 27. __________ is information a program gathers from the outside world. 28. __________ is information a program sends to the outside world. 29. A(n) __________ is a diagram that graphically illustrates the structure of a program.
6.
// This program will output the circumference and area
// of the circle with a given radius.
#include
using namespace std;
const double PI = 3.14;
const double RADIUS = 5.4;
int main()
{
area // definition of area of circle
float circumference; // definition of circumference
circumference = 2 * PI * RADIUS; // computes circumference
area = ; // computes area
// Fill in the code for the cout statement that will output (with description)
// the circumference
// Fill in the code for the cout statement that will output (with description)
// the area of the circle
return 0;
}
7.
// This program will write the name, address and telephone
// number of the programmer.
#include
using namespace std;
int main()
{
// Fill in this space to write your first and last name
// Fill in this space to write your address (on new line)
// Fill in this space to write you city, state and zip (on new line)
// Fill in this space to write your telephone number (on new line)
return 0;
}
8.
// This program demonstrates the use of characters and strings
#include
#include
using namespace std;
// Definition of constants
const string FAVORITESODA = "Dr. Dolittle"; // use double quotes for strings
const char BESTRATING = 'A'; // use single quotes for characters
int main()
{
char rating; // 2nd highest product rating
string favoriteSnack; // most preferred snack
int numberOfPeople; // the number of people in the survey
int topChoiceTotal; // the number of people who prefer the top choice
// Fill in the code to do the following:
// Assign the value of "crackers" to favoriteSnack
// Assign a grade of 'B' to rating
// Assign the number 250 to the numberOfPeople
// Assign the number 148 to the topChoiceTotal
// Fill in the blanks of the following:
cout << "The preferred soda is " << << endl;
cout << "The preferred snack is " << << endl;
cout << "Out of " << << " people "
<< << " chose these items!" << endl;
cout << "Each of these products were given a rating of " << ;
cout << " from our expert tasters" << endl;
cout << "The other products were rated no higher than a " << rating
<< endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
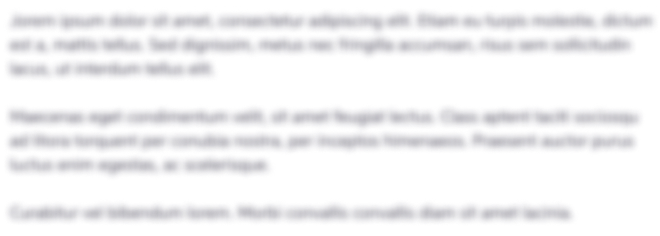
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started