Question
1. Twenty-One Problem Expected Duration: 2-3 hours For this project you will implement a game of Twenty-One with a dealer and one player. It is
1. Twenty-One
Problem
Expected Duration: 2-3 hours
For this project you will implement a game of Twenty-One with a dealer and one player. It is possible to implement this game in procedural stylebut for this assignment that is not sufficient. You must use objects and classes as described below, because the main purpose is to introduce you to classes and objects. You will create a console application to play the game. Betting, and other rules associated with betting, are not part of this project.
Summary of Rules
Many versions of this game exist that people play. Well be using simplified rules.
The goal of the game for the player is to reach 21. The dealer deals cards. The values of all cards dealt to a player are added together.
If a players sum exceeds 21, the player busts and loses immediately.
If a players sum is exactly 21, they win against the dealer immediately.
In all other cases, the player wins if the sum of their cards is greater than the sum of the dealers cards; the player loses if their sum is less than the dealer.
In any hand, the dealer must take cards until their hand is at least 17 points.
Each face card has a value of 10.
Each number card has its numeric value.
An Ace may be counted as 1 or 11, whichever is better for the hand.
Dealing of Cards and Game Play
The dealer deals a card face up to the player and then to itself.
The dealer deals a second card to the player face up.
If the player has two aces, the first one must count as a one.
The dealer gives itself a second card face down. If a card is face down, display a question mark in pace of the value and a space where the suit would be.
The player has to decide whether to stand or hit. a. if the player stands, then their turn is over and its the dealer turn. b. if the player hits, another card is dealt to the player and they decide again.
The dealer reveals the hidden card. a. if its hand is less than 17, the dealer must take another card. b. once the dealers hand is at least 17, the dealer must stand.
When counting the score for a hand, only count cards that are face up/showing.
This image shows an example game state after the initial deal.
This image shows a game state at the end of an example game.
Design of the Game
OOP is about how code and data are organized. Where you put code and data can make other code that uses it either simpler or more complex. You MUST have:
the classes listed below
each class must have a constructor
in your code.
Other attributes and methods described in each class below are strong suggestions, but not strictly required if another organization makes more sense to you.
Card class
A Card class represents one single card. A card typically has the following attributes:
suit -> one of {"Spades", "Clubs", "Hearts", "Diamonds"}
value -> one of {"A", "K", "Q", "J", 2 up to "10"}
numeric_value -> An ace is either 1 or 11, {10, Jack, Queen, King} are 10, numeric cards have their numeric value.
Suggestions:
A __str__ magic method that returns the card as a string in ASCII art. All cards should be the same size and format.
a boolean flag that indicates whether the card is face up or face down.
Deck class
A deck is a stack of 52 cards. A deck can be shuffled, and usually cards are dealt from only the top or only the bottom.
If you want to print nice unicode characters for suits, and keep track of card values, the following items may be helpful:
suits = ["Spades", "Hearts", "Clubs", "Diamonds"] suits_values = {"Spades":"\u2664", "Hearts":"\u2661", "Clubs": "\u2667", "Diamonds": "\u2662"} cards = ["A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"] numeric_values = {"A": 11, "2":2, "3":3, "4":4, "5":5, "6":6, "7":7, "8":8, "9":9, "10":10, "J":10, "Q":10, "K":10}
An alternate dictionary for suit values should work if your console does not support unicode strings (Windows most likley:
suits_values = {"Spades":chr(6), "Hearts":chr(3), "Clubs": chr(5), "Diamonds": chr(4)}
Dealer class
The dealer deals cards, but also plays a hand. The rules for how a dealer plays are different from how a player plays.
Suggestions:
Hand attribute -> the collection of cards dealt to the dealer
Deck attribute -> the dealer has the deck
deal() method -> the dealer deals a card from the deck
__str__ -> magic method returns the dealers hand and total score as a string
Player class
The player plays a hand. The rules for how a player plays are different from how a dealer plays.
Suggestions:
Hand attribute -> the collection of cards dealt to the player
__str__ -> magic method returns the playerss hand and total score as a string
Hand class
A hand is 0, 1 or more cards that have been dealt to a player or to the dealer. The score of any hand must take into account the value of aces, so its more than just a simple sum for both dealer and player.
Suggestions:
add() method -> adds a card to the hand. If its an ace, check whether it should be a 1 or an 11. This check can also be done in the sum method.
__str__ method -> magic method to return the collection of cards as a ASCII art string
__len__ method -> magic method to return how many cards are in the hand,
sum() method -> return the total score of a hand. The total is affected by the value of aces being 1 or 11, whichever gives the best score.
Game class
A game instance runs a game and keeps track of the state of the game.
Suggestions: run() method -> implements the pseudocode algorithm given in the module docstring.
player_turn() method -> implement code for the players turn.
dealer_turn() method -> implement code for the dealers turn.
player attribute
dealer attribute
Clearing the console
It is often convenient when debugging to see the sequence of cards displayed as they are dealt to a player. But when the game is running, it is often nicer to clear the console. The following utility function accomplishes this:
def clear(): """Clear the console.""" # for windows if os.name == 'nt': _ = os.system('cls') # for mac and linux, where os.name is 'posix' else: _ = os.system('clear')
Testing Your Code
You are not required to create nor submit test code for this project. However, automated testing of pieces of your code to make sure they work is good practice and will save you time.
We provide you a sample pytest file test_twentyone.py file to show what the beginnings of a useful test file might look like. The most notable thing is that its only using simple asserts to check for expected values.
Some day, a more complete version of that file could become an autograder.
Here is a starting code:
'''
Project: Twenty One Game:
Course:
Name:
Due Date:
Description:
This is skeleton starter code for the Twenty-One Game.
Typical pseudocode for such a game would be:
1. initial deal
2. player's turn
3. If player gets twenty-one, immediate win
4. dealer's turn
5. check for winner
6. print results
'''
import os
class Card:
pass
class Deck:
pass
class Hand:
pass
class Dealer:
pass
class Player:
pass
class Game:
def run(self):
pass
def clear():
"""Clear the console."""
# for windows
if os.name == 'nt':
_ = os.system('cls')
# for mac and linux, where os.name is 'posix'
else:
_ = os.system('clear')
def main():
pass
if __name__ == '__main__':
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
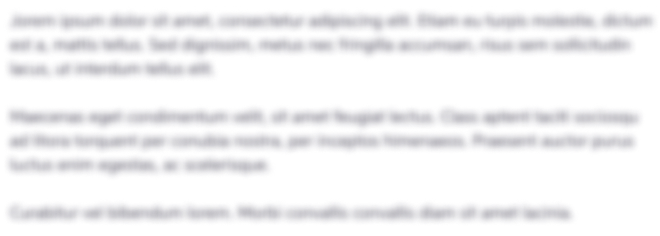
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started