Question
1.) Use the attached skeleton to write a sorting class that has 3 methods that will accept an array of ints to sort using each
1.) Use the attached skeleton to write a sorting class that has 3 methods that will accept an array of ints to sort using each of these algorithms: insertion, selection, & bubble. Note: Make sure the sorting is in ASCENDING order!
2.) Write a driver that will do the following:
a.) Create an array of 20,000 ints (generate the numbers randomly in a loop, in the range of 1 - 5000)
b.) Call each of the sorting methods, passing it the array of 20,000 ints (Note: make a copy of the array before sorting the copy).
c.) Calculate the number of nanoseconds taken to sort the same array, using each of the 3 algorithms.
d.) Display the time for each. Say which of the 3 algorithms was fastest (most efficient).
Optional - extra: To prove that the algorithm is working, create a small array of 10 randomly generated ints between 1 and 100, and sort them using each of the 3 sorting methods, and print them after each sort.
Make a copy of the original array, so that you are re-sorting an unsorted array, instead of the sorted array. This is proof of concept.
Hint: Make a copy of the original array, so that you use the unsorted version as input to each method.
Hint #2: Use either System.currentTimeMillis() or System.nanoTime() to get the start and end time assigned to 2 long variables, then subtract, and that is your total time.
Extra Credits: Do the same for mergeSort and quickSort.
please use the easiest java way to solve this program, and use comments through out since i am learning.
Here is the skeleton.
package sortingalgos;
/** * * @author mtsguest */ public class SortingAlgos {
public static int[] myOriginalUnsortedArray1 = new int[20000]; public static int[] myCopyUnsortedArray2 = new int[20000]; public static long bubbleSortDuration, quickSortDuration, selectionSortDuration, insertionSortDuration, mergeSortDuration; /** * @param args the command line arguments */ public static void main(String[] args) { } public void generateRanNums(){ } copyRanNums(); quickSort(); //Extra Credit! copyRanNums(); mergeSort(); //Extra Credit! copyRanNums(); selectionSort(); copyRanNums(); insertionSort(); copyRanNums(); bubbleSort(); compareSortTimes(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
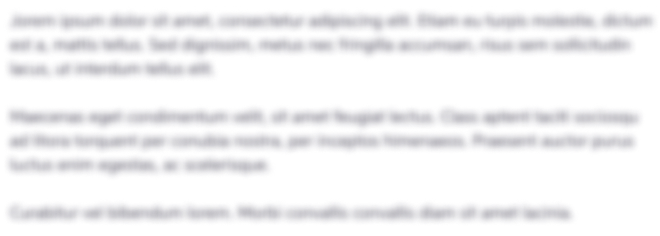
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started