Question
1) What is the value of x after the following statements execute? int x = 25; int *p; p = &x; *p = 46; 2.
1) What is the value of x after the following statements execute?
int x = 25;
int *p;
p = &x;
*p = 46;
2. If you overload the binary arithmetic operator + as a member function, how many objects must be passed as parameters?
3. If you overload the binary arithmetic operator + as a friend function, how many objects must be passed as parameters?
4. Given the following class:
class Money
{
public:
Money(int d, int c);
//initializes the object with the parameters
Money();
//intialized the object so its value is $0.00
double getValue(); //returns the amount of money as a double
void nicePrint() const; //prints out the money in nice format
private:
int dollars;
int cents;
};
Money::Money(int d, int c)
//initializes the object with the parameters
{
dollars = d;
cents = c;
}
Money::Money()
{
dollars = 0;
cents = 0;
}
double Money::getValue()//returns the amount of money as a double
{
return (dollars + cents/100.00);
}
void Money::nicePrint() const
{
cout << '$' << dollars << '.' << setfill('0') << setw(2) << cents << endl;
cout << setfill(' ');
}
a. Write the prototype and definition of the overloaded operator + as a member function.
b. Write the prototype and definition of the overloaded operator < as a friend function.
c. Assume that all the functions from the class definition, and from part a and b of this question are properly defined.
What is printed by the following code?
int main()
{
Money yours(2,34);
Money mine(4,5);
yours.nicePrint();
mine.nicePrint();
cout << (mine + yours).getValue();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
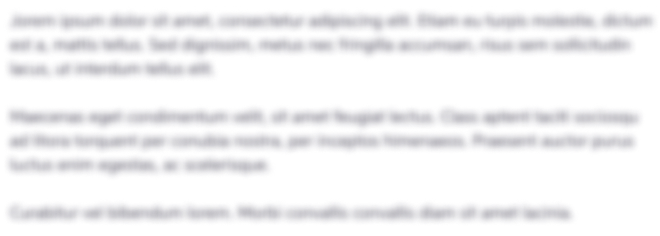
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started