Question
1. Which of the following is a value that is written into the code of a program? a. a literal c. an operator b. an
1. Which of the following is a value that is written into the code of a program?
a. | a literal | c. | an operator |
b. | an assignment statement | d. | a variable |
2. A Java program must have at least one of the following:
a. | a comment |
b. | a class definition |
c. | a System.out.println(); statement |
d. | a variable declaration |
3. Which of the following would contain the translated Java byte code for a program named Demo?
a. | Demo.java | c. | Demo.class |
b. | Demo.code | d. | Demo.byte |
4. Which of the following is a named storage location in the computer's memory?
a. | a literal | c. | a constant |
b. | an operator | d. | a variable |
5. Which of the following is not a valid Java comment?
a. | /** Comment one */ | c. | // Comment three |
b. | */ Comment two /* | d. | /* Comment four */ |
6. To compile a program named First you would use which of the following commands?
a. | java First.java | c. | javac First.java |
b. | javac First | d. | compile First.javac |
7. A Java source file must be saved with the extension
a. | .java | c. | .src |
b. | .javac | d. | .class |
8. Which of the following is not a rule that must be followed when naming identifiers?
a. | After the first character, you may use the letters a-z, A-Z, an underscore, a dollar sign, or the digits 0-9. |
b. | Identifiers can contain spaces. |
c. | Uppercase and lowercase characters are distinct. |
d. | The first character must be one of the letters a-z, A-Z, an underscore, or a dollar sign. |
9. Character literals are enclosed in __________ and string literals are enclosed in __________.
a. | single quotes, double quotes |
b. | double quotes, single quotes |
c. | single quotes, single quotes |
d. | double quotes, double quotes |
10. Variables are classified according to their
a. | names | c. | locations |
b. | values | d. | data types |
11. What is the result of the following expression?
17 % 3 * 2 - 12 + 15
a. | 105 | b. | 12 | c. | 7 | d. | 8 |
12. What is the result of the following expression?
10 + 5 * 3 - 20
a. | -5 | b. | -50 | c. | 5 | d. | 25 |
13. In the following Java statement, what value is stored in the variable name?
String name = "John Doe";
a. | "name" |
b. | the memory address where "John Doe" is located |
c. | the memory address where name is located |
d. | John Doe |
14. What is the value of z after the following statements have been executed?
int x = 4, y = 33;
double z;
z = (double) (y / x);
a. | 8.25 | b. | 4 | c. | 0 | d. | 8.0 |
15. What output will be displayed as a result of executing the following code?
int x = 5, y = 20;
x += 32;
y /= 4;
System.out.println("x = " + x + ", y = " + y);
a. | x = 160, y = 80 |
b. | x = 32, y = 4 |
c. | x = 37, y = 5 |
d. | x = 9, y = 52 |
16. Which of the following statements will correctly convert the data type, if x is a float and y is a double?
a. | x = float y; | c. | x = (float)y; |
b. | x = | d. | x = y; |
17. Which of the following statements is invalid?
a. | double r = 9.4632E15; | c. | double r = 2.9X106; |
b. | double r = 9.4632e15; | d. | double r = 326.75; |
18. To print "Hello, world" on the monitor, which of the following Java statements should be used?
a. | System.out.println("Hello, world"); |
b. | System Print = "Hello, world"; |
c. | SystemOutPrintln('Hello, world'); |
d. | system.out.println(Hello, world); |
19. The boolean data type may contain which of the following range of values?
a. | -128 to + 127 |
b. | true or false |
c. | -2,147,483,648 to +2,147,483,647 |
d. | -32,768 to +32,767 |
20. Variables of the boolean data type are useful for
a. | evaluating conditions that are either true or false |
b. | working with small integers |
c. | working with very large integers |
d. | evaluating scientific notation |
21. What would be displayed as a result of executing the following code?
int x = 578;
System.out.print("There are " +
x + 5 + " " +
"hens in the hen house.");
a. | There are 583 hens in the hen house. |
b. | There are 5785 hens in the hen house. |
c. | There are x5 hens in the hen house. |
d. | There are 5785 hens in the hen house. |
22. What would be displayed as a result of executing the following code?
final int x = 22, y = 4;
y += x;
System.out.println("x = " + x + ", y = " + y)
a. | x = 22, y = 26 | c. | x = 22, y = 88 |
b. | x = 22, y = 4 | d. | Nothing. There is an error in the code. |
23. What would be displayed as a result of executing the following code?
int x = 15, y = 20, z = 32;
x += 12;
y /= 6;
z -= 14;
System.out.println("x = " + x +
", y = " + y +
", z = " + z);
a. | x = 27, y = 3.333, z = 18 |
b. | x = 27, y = 2, z = 18 |
c. | x = 37, y = -14, z = 4 |
d. | x = 27, y = 3, z = 18 |
24. What is the value of z after the following code is executed?
int x = 5, y = 28;
float z;
z = (float) (y / x);
a. | 5.6 | b. | 3.0 | c. | 5.0 | d. | 5.60 |
25. Which of the following statements correctly creates a Scanner object for keyboard input?
a. | Scanner kbd = new Scanner(System.keyboard); |
b. | Scanner keyboard = new Scanner(System.in); |
c. | Scanner keyboard(System.in); |
d. | Keyboard scanner = new Keyboard(System.in); |
26. Which Scanner class method reads a String?
a. | nextLine | c. | nextString |
b. | charAt | d. | getLine |
27. The primitive data types only allow a(n) __________ to hold a single value.
a. | class | c. | object |
b. | literal | d. | variable |
28. In Java, __________ must be declared before they can be used.
a. | variables | c. | key words |
b. | literals | d. | comments |
29. If the following Java statements are executed, what will be displayed?
System.out.println("The top three winners are ");
System.out.print("Jody, the Giant ");
System.out.print("Buffy, the Barbarian");
System.out.println("Adelle, the Alligator");
a. | The top three winners are Jody, the Giant Buffy, the Barbarian Adelle, the Alligator |
b. | The top three winners are Jody, the Giant Buffy, the BarbarianAdelle, and the Albino |
c. | The top three winners are Jody, the Giant Buffy, the BarbarianAdelle, the Alligator |
d. | The top three winners are Jody, the Giant Buffy, the BarbarianAdelle, the Alligator |
30. A value that is written into the code of a program is a(n) __________.
a. | literal | c. | variable |
b. | assignment statement | d. | operator |
31. When the + operator is used with strings, it is known as the
a. | assignment operator | c. | addition operator |
b. | string concatenation operator | d. | combines assignment operator |
32. What would be printed out as a result of the following code?
System.out.println("The quick brown fox" +
"jumped over the "
"slow moving hen.");
a. | The quick brown fox jumped over the slow moving hen. |
b. | The quick brown fox jumped over the slow moving hen. |
c. | The quick brown fox jumped over the slow moving hen. |
d. | Nothing - this is an error |
33. Which of the following is not a rule that must be followed when naming identifiers?
a. | The first character must be one of the letters a-z, A-Z, and underscore or a dollar sign. |
b. | Identifiers can contain spaces. |
c. | Uppercase and lowercase characters are distinct. |
d. | After the first character, you may use the letters a-z, A-Z, the underscore, a dollar sign, or digits 0-9. |
34. Which of the following cannot be used as identifiers in Java?
a. | variable names | c. | key words |
b. | class names | d. | objects |
35. Which of the following is not a primitive data type?
a. | short | c. | float |
b. | long | d. | string |
36. Which of the following is valid?
a. | float y; y = 54.9; | c. | float w; w = 1.0f; |
b. | float y; double z; z = 934.21; y = z; | d. | float v; v = 1.0 |
37. If x has been declared an int, which of the following statements is invalid?
a. | x = 0; | c. | x = 1,000; |
b. | x = -59832; | d. | x = 592 |
38. To display the output on the next line, you can use the println method or use the __________ escape sequence in the print method.
a. |
| b. |
| c. | \t | d. | \b |
39. Every Java application program must have
a. | a class named MAIN | c. | at least two data types |
b. | a method named main | d. | integer variables |
40. What will be displayed as a result of executing the following code?
int x = 6;
String msg = "I am enjoying this class.";
String msg1 = msg.toUpperCase();
String msg2 = msg.toLowerCase();
char ltr = msg.charAt(x);
int strSize = msg.length();
System.out.println(msg);
System.out.println(msg1);
System.out.println(msg2);
System.out.println("Character at index x = " + ltr);
System.out.println("msg has " + strSize + "characters.");
a. | I am enjoying this class. I AM ENJOYING THIS CLASS. i am enjoying this class. Character at index x = e msg has 24 characters. | c. | I am enjoying this class. I AM ENJOYING THIS CLASS. i am enjoying this class. Character at index x = n msg has 24 characters. |
b. | I am enjoying this class. I AM ENJOYING THIS CLASS. i am enjoying this class. Character at index x = e msg has 25 characters. | d. | I am enjoying this class. I AM ENJOYING THIS CLASS. i am enjoying this class. Character at index x = n msg has 25characters. |
41. What will be displayed as a result of executing the following code?
public class test
{
public static void main(String[] args)
{
int value1 = 9;
System.out.println(value1);
int value2 = 45;
System.out.println(value2);
System.out.println(value3);
value = 16;
}
}
a. | 9 45 16 | c. | 9 45 16 |
b. | 94516 | d. | Nothing. Code will not compile |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
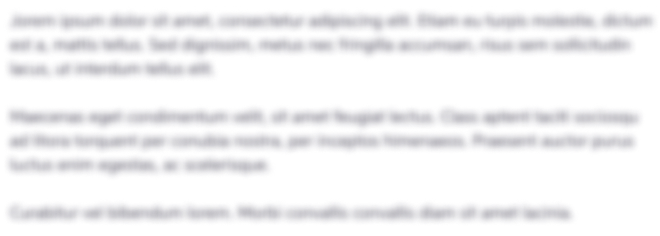
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started