Question
1. Write a method to get a random number for the year the car was built. The method name should be getRandomYear. This method doesnt
1. Write a method to get a random number for the year the car was built. The method name should be getRandomYear. This method doesnt take in any parameters and returns an integer value. Inside the method get a random value in the range between 1950 and 2020 using the random generator.
2. Write a method to get a random integer value which represents number of doors that a car has. The method should be named getRandomNumDoors. It doesnt take anything in, and returns an integer value. Using the random generator return a value between 1 and 4(inclusive).
3. The last method which you will write will be named createData. This method takes in two parameters, the first one is the file name in a form of a String. The file name specifies where the program should write the information to. The second parameter represents the number of data samples we want in the file. The method doesnt return anything. Make sure you include throws IOException in the method header. Inside the method create an instance of FileWriter. Dont forget to import it on top. Make a loop and inside the loop call the appropriate methods to get the car make, model, year, color, ..etc and write to the file. After the loop, make sure to close the writer. Refer to the lecture slides and lab assignment for help on how to write a method. Or how to call one and save the returning value into a variable.
4. Compile and run your code. Fix any syntax errors which may occur. Check the text file to see if the data is formatted nicely with comas, and to make sure it has only one data sample per line.
Here is the given file:
import java.util.Random;import java.io.*;public class ArtificialData{ /* Method name : getRandomMake Parameter list : Return type : String Description : This method returns a random car make. There are a few car makes predefined and stored in an array of type String. Using the random generator a random car make will be returned. */ public static String getRandomMake(){ String[] makes = {"Toyota", "BMW", "Dodge", "Carriage", "Ferrari", "Sled", "Chevrolet", "Hyundai", "Ford", "Mercedes", "Honda"}; Random rand = new Random(); int randomIndex = rand.nextInt(makes.length); String randomMake = makes[randomIndex]; return randomMake; } /* Method name : getRandomModel Parameter list : Return type : String Description : This method returns a random car model. There are a few car modelspredefined and stored in an array of type String. Using the random generator a random car model will be returned. */ public static String getRandomModel(){ String[] models = {"335xi", "Civic", "Camry", "G500", "Huracan", "3500", "Genesis", "Corvette", "Viper", "Model S", "Corolla"}; Random rand = new Random(); int randomIndex = rand.nextInt(models.length); String randomModel = models[randomIndex]; return randomModel; } /* Method name : getRandomMPG Parameter list : Return type : String Description : This method returns a random value between 7 and 80. The value represents miles per gallon(mpgs). It is in a form of a string so it can be formatted to have one decimal place. */ public static String getRandomMPG(){ int lowest = 7, highest = 80;
Random rand = new Random(); double randomDouble = rand.nextDouble(); int randomInt = rand.nextInt((highest - lowest)+1)+lowest; String randomMPG = String.format("%.1f", (randomInt + randomDouble)); return randomMPG; } /* Method name : getRandomColor Parameter list : Return type : String Description : This method returns a random car Color. There are a few car colorspredefined and stored in an array of type String. Using the random generator a random color will be returned. */ public static String getRandomColor(){ String[] colors = {"Green", "White", "Red", "Purple", "Blue", "Pink", "Black", "Lime", "Brown", "Maroon", "Orange", "Yellow"}; Random rand = new Random(); int randomIndex = rand.nextInt(colors.length); String randomColor = colors[randomIndex]; return randomColor; } /* Method name : getRandomYear Parameter list : Return type : int Description : This method returns a random year. between 1950 and 2020. //WRITE THE METHOD */ /* Method name : getRandomNumDoors Parameter list : Return type : int Description : This method returns a random number to represent number of doors on the car. The random doors will be chosen between 1 and 4(inclusive). //WRITE THE METHOD */ /* Method name : createData Parameter list : String fileName, int numsOfData Return type : void
Description : This method writes to the file one line at a time. It takes in two parameters, first is the file name of type String. The second one is an integer number for how many data samples you want. Make sure to add "throws IOException" after the closing parenthesis and before the opening curly braket for the body of the method. Inside the method call each of the methods to get the make, model,year,.. and write it to the file comma separated. Make a loop do this as many times as the value which will be passed into the parameter "numsOfData". Make sure you close the writer at the end. WRITE THE METHOD */ /* The main method, calls one method and has a print statement once its finished. createData("CarData.txt", 3000) specifies the name of the file and that you want 3000 samples of data to be writen to the file. */ public static void main(String[] args)throws IOException{ //createData("CarData.txt", 3000); System.out.println("Finished.."); }}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
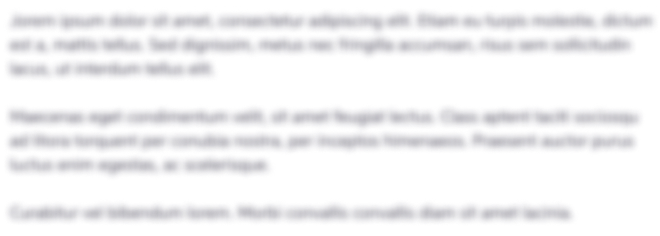
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started