Question
1. Write a program merge.c that include the following function: void merge(int n, int a1[], int a2[], int a3[]); that has a parameter as an
1. Write a program merge.c that include the following function:
void merge(int n, int a1[], int a2[], int a3[]);
that has a parameter as an integer array a1[] of length n and another parameter an integer array a2[] of length n. The function merges the contents of the two integer arrays a1 and a2 to a3. Thus, if a1[] contains the values -9, 16, 0, 2, and a2[] contains 1, 3, 5, 4, then make a3 equal to -9, 1, 16, 3, 0, 5, 2, 4. Nothing is returned.
In the main function, ask the user to enter the length of the arrays, declare the arrays, read in the values for the two arrays, and call the merge function to compute the third array. The main function should display the result of the third array.
Enter the length of the array: 3
Enter the elements of the first array: 3 4 9
Enter the elements of the second array: 7 2 1
Output: The output array is: 3 7 4 2 9 1 2.
2. Write a program range.c that include the following functions.
int consecutive(int a[], int n);
int inRange(int a[], int n, int low, int high);
The consecutive function tests whether the integer array has three consecutive numbers with the same value. The function returns 1 if there are three consecutive numbers with the same value and return 0 if not. Assumes that the array has at least three elements;
The inRange function compares elements of the array with low and high, returns 1 if all the elements of the array are in the range (inclusive) and returns 0 if there is element in the array that are not in the range.
In the main function, ask the user to enter the length of the array, declare the array with the length, read in the values for the array and low and high, and call the two functions. The main function should display the result.
Sample run #1:
Enter the length of the array: 5
Enter the elements of the array: 3 4 4 4 9
Enter low and high: 2 9
Output:
There are three consecutive numbers with the same value in the array.
All numbers are in the range.
Sample run #2:
Enter the length of the array: 5
Enter the elements of the array: 3 7 4 4 1
Enter low and high: 2 9
Output:
The array does not contain three consecutive numbers with the same value.
There are elements in the array that are not in the range.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
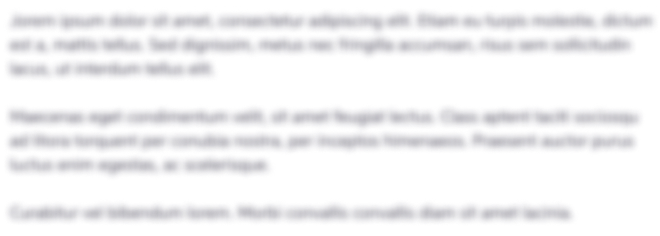
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started