Question
1. Write a program that adds the values of an integer array. Declare an array of integer values that will represent the first five odd
1. Write a program that adds the values of an integer array.
Declare an array of integer values that will represent the first five odd numbers: 1, 3, 5, 7, and 9.
Initialize each element of the array with the prime number values shown above.
Calculate the sum of the array elements, and store the result in a variable named sum. You must access the array elements to accomplish this. Do not write
sum = 1 + 3 + 5 + 7 + 9 ;
or
sum = 25
Print the calculated sum to the screen along with a message describing what is being printed.
2. The following code segment is part of a bigger program to add the corresponding values of two arrays, a1 and a2. Arrays a1 and a2 are declared and initialized to store five doubles.
double[ ] a1 = new double[5]; double[ ] a2 = new double[5]; // Assume that there is code here that prompts the user to enter these 10 double // values and stores them in a1 and a2. You don't know what the user will type, but // you may assume that the code is correct and no errors occur in this portion of the program.
// Complete your code below this line
Add code to the segment shown above to do the following: - declare an additional array of five doubles. - fill the new array with the sum of the corresponding elements in a1 and a2. - print the values stored in the new array to the screen.
For example, if the first two arrays hold the following values:
{1.2, 2.3, 3.4, 4.5, 5.6} and{1.0, 2.0, 3.0, 4.0, 5.0},
your array should be filled with the following values:
{2.2, 4.3, 6.4, 8.5, 10.6}
Complete the code segment to accomplish the additional tasks described.
3. Note that ^ means "raised to the power of" in this problem! It is only used to indicate exponentiation in the example.
Fermat's Last Theorem says that there are no integers a, b, and c such that a^n + b^n =c^n, except in the case when n = 2 (the familiar Pythagorean Theorem).
Assume that you are given four integer values that have been initialized: a, b, c, and n.
Write a program that verifies Fermat's Theorem for the integers a, b, c, and n. (Do not use 2 as a value for n.)
For the values assigned to the variables, - print "Fermat was correct!" if the left side of the equation does not equal the right side. - print "Fermat was wrong!" if the left side of the equation equals the right side (remember n may not equal 2).
Since the ^ symbol is not a valid Java operator, you may use the Math.pow(double, double) method in your solution. Math.pow( ) takes two double parameters and returns the double value of its first parameter raised to the value of its second parameter as shown below. Both of these expressions return the value of 8.0
double answer1 = Math.pow(2, 3); double answer2 = Math.pow(2.0, 3.0);
Notice that answer1 and answer2 are both doubles. You may need to evaluate these as integers in your program.
Write your program to test Fermat's Last Theorem with specific values of a, b, c, and n below.
4. The following data represent Richter Scale data for earthquakes. Write a program to calculate and print the average of any valid earthquake data.
Store the Richter values in an array of doubles named quakeLevels.
Unfortunately, your seismograph is known to sometimes produce unreliable readings (like the value of 10.1 in this example). So you decide to throw out the maximum and minimum readings.
Your program should do the following:
- declare and initialize the quakeLevels array using the following data. { 5.6, 6.2, 4.0, 5.5, 5.7, 6.1,7.4, 8.5, 5.5, 6.3, 6.4, 2.1, 6.9, 4.3, 3.1, 7.0, 10.1 } - determine the maximum and minimum values in the array. - compute the average of the array contents, excluding the maximum and minimum values. - print the values in the array excluding the maximum and minimum values. - print the average
Complete your code below:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
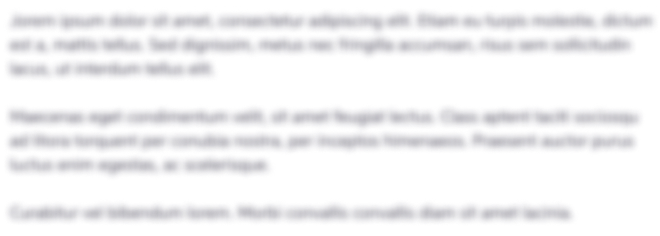
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started