Answered step by step
Verified Expert Solution
Question
1 Approved Answer
1. Write decisions and loops to satisfy requirements. 2. Implement data structures such as lists, tuples, and/or dictionaries 3. Work with user input 4.
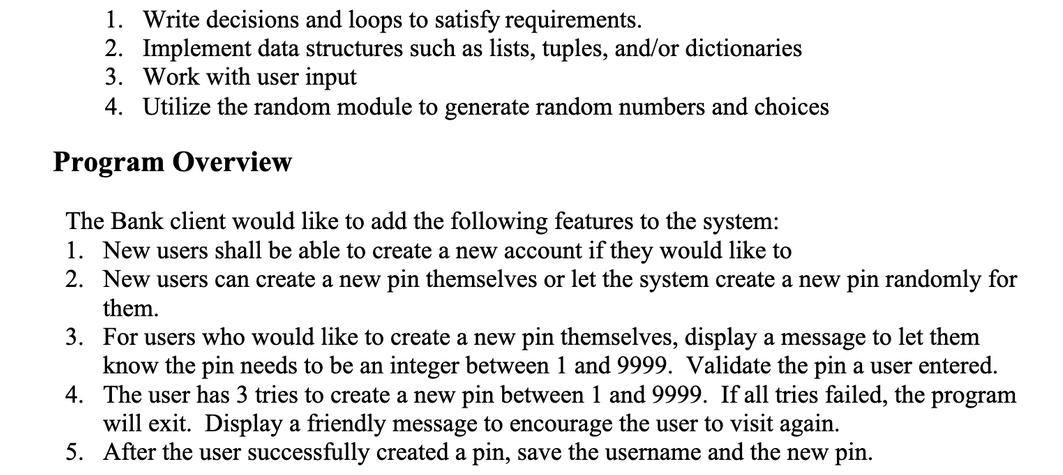
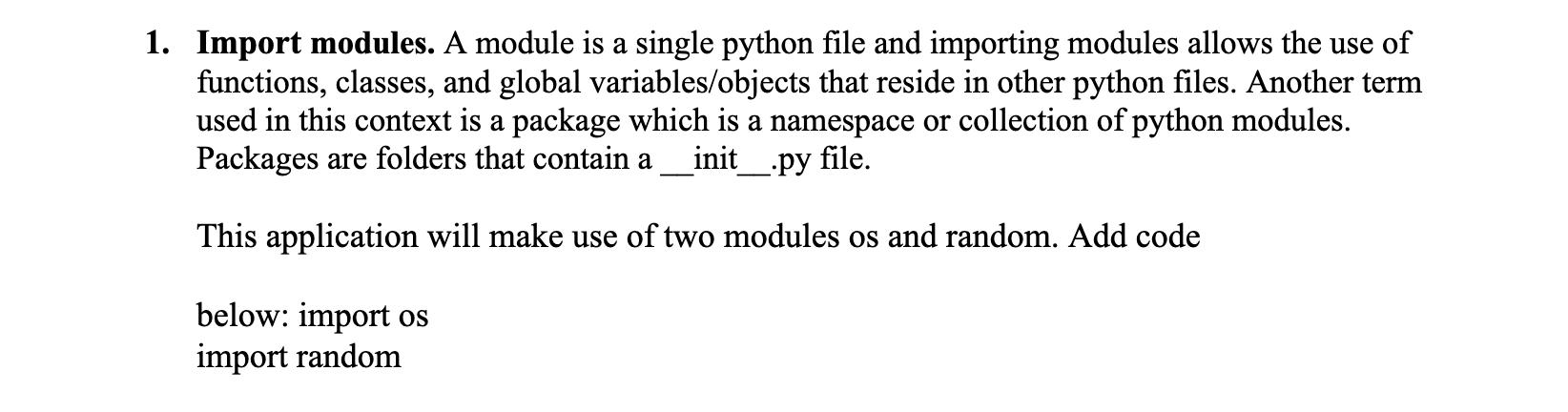
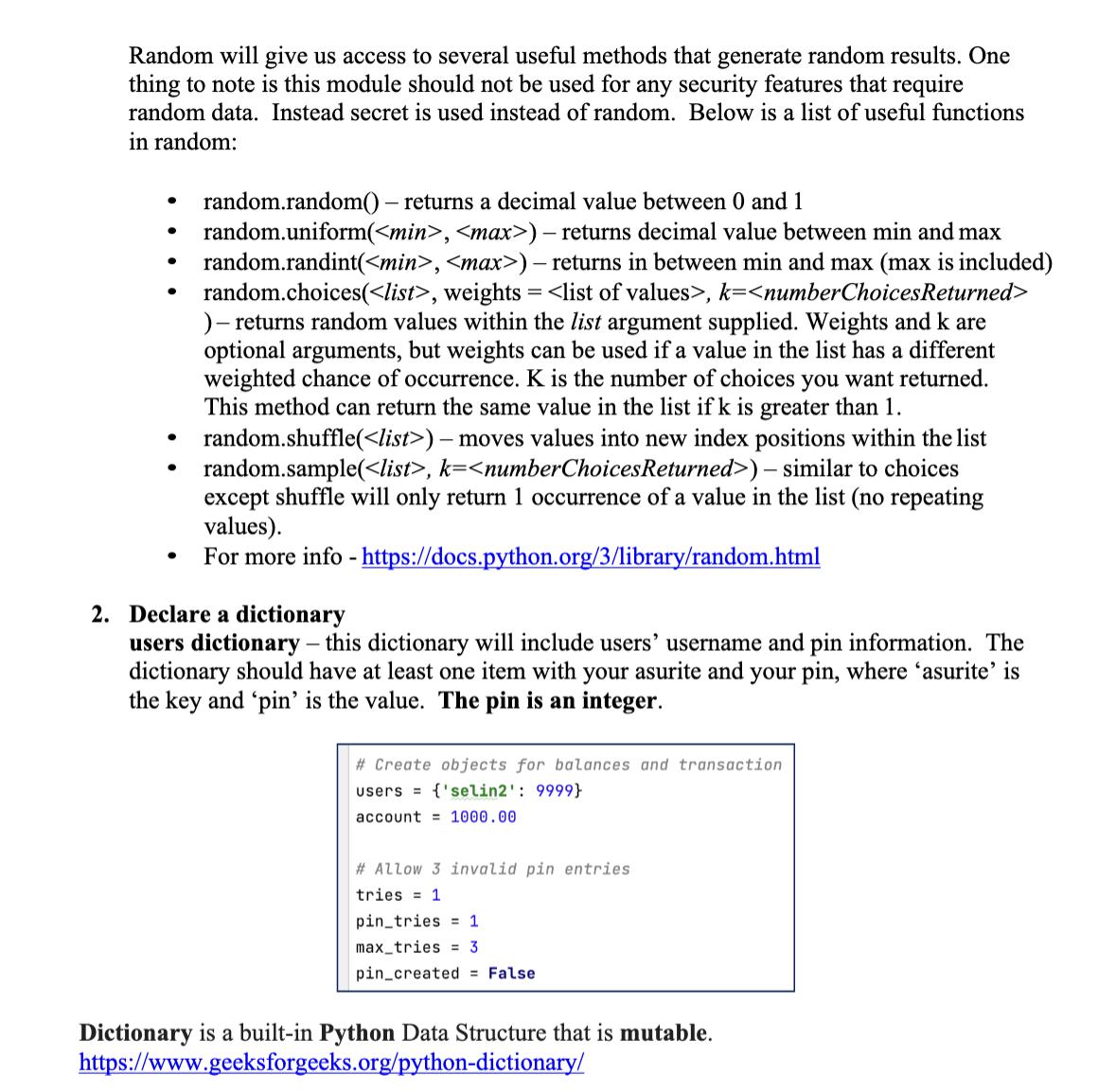
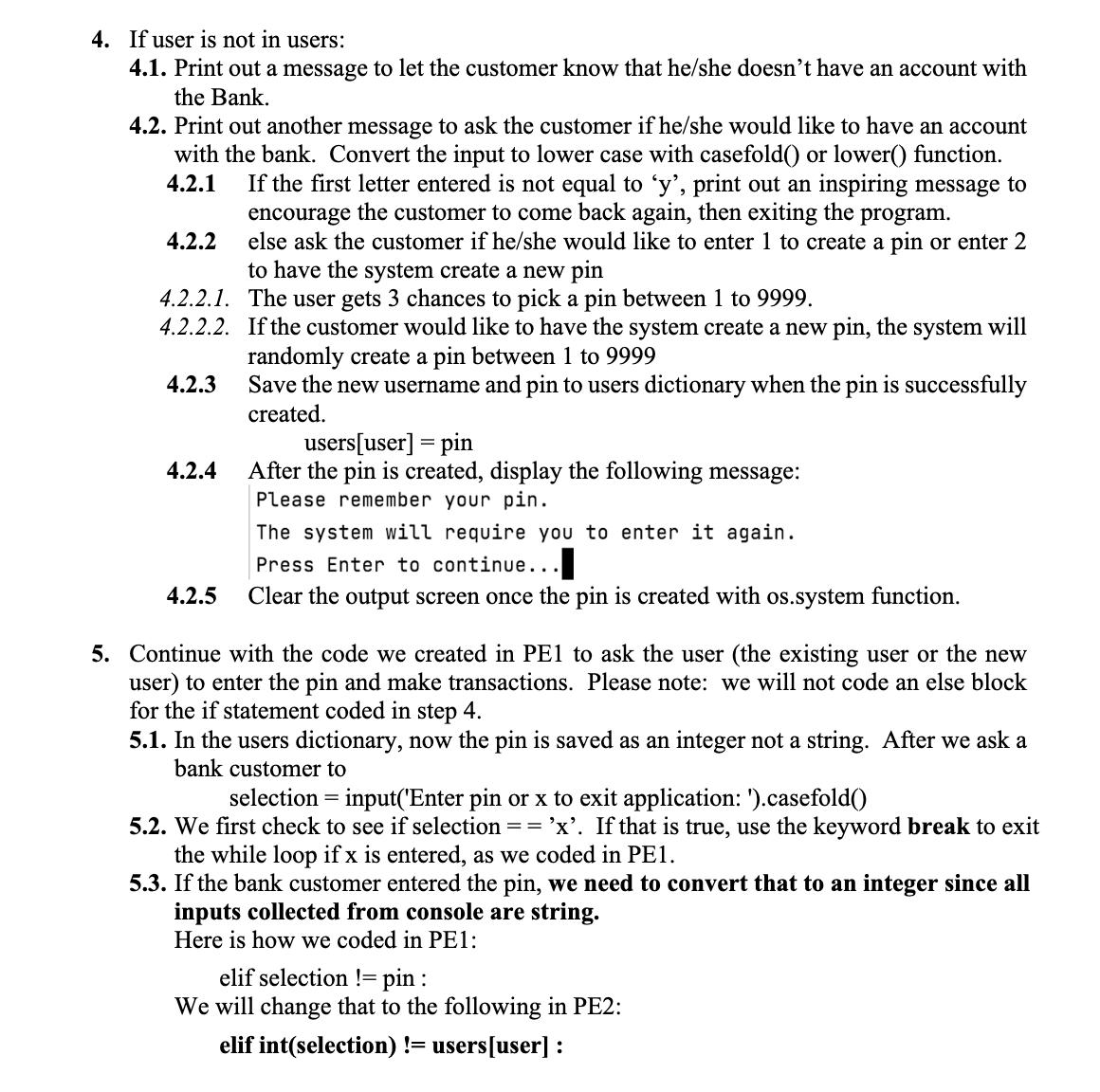
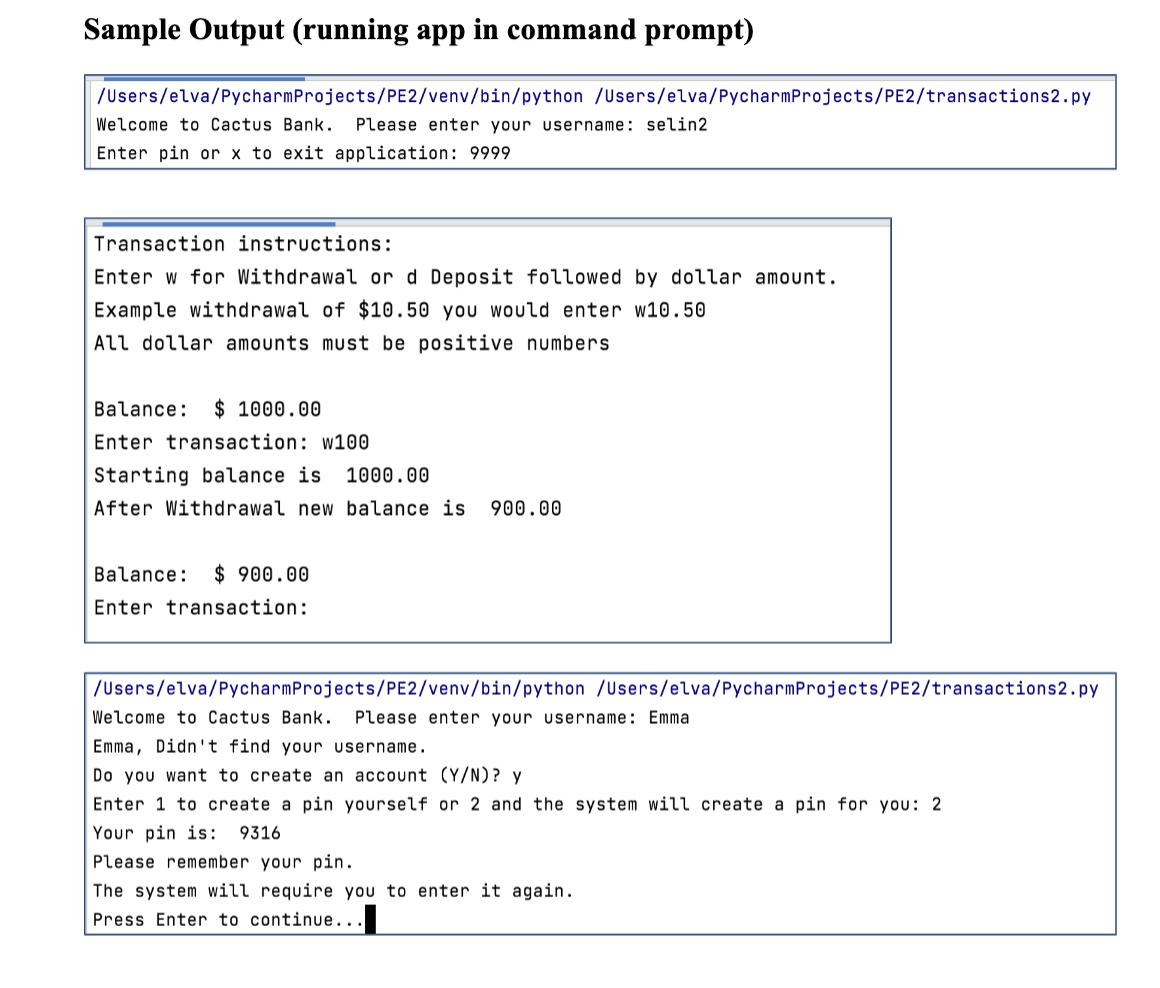
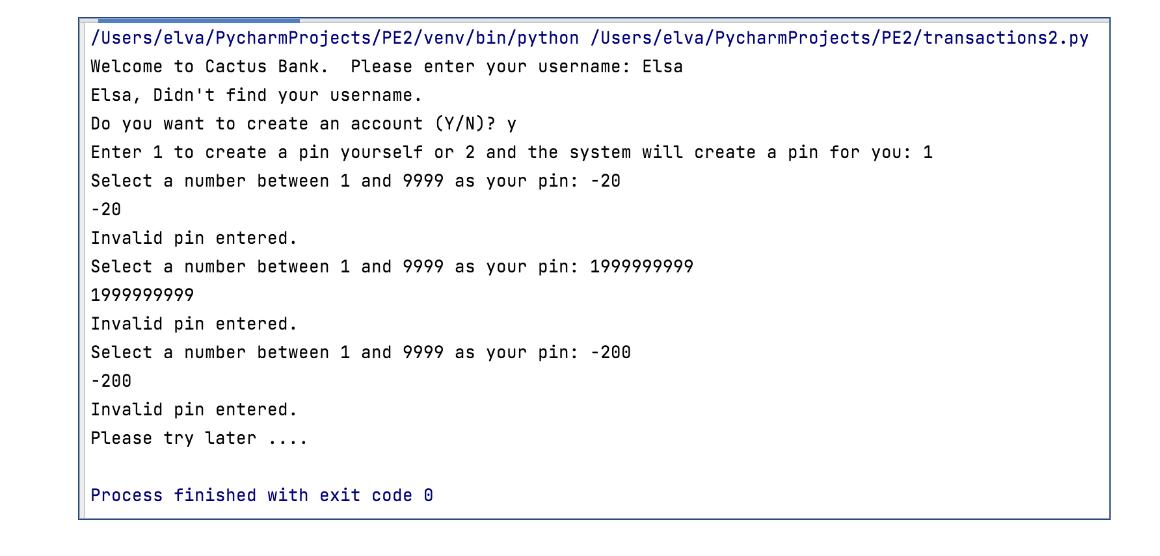
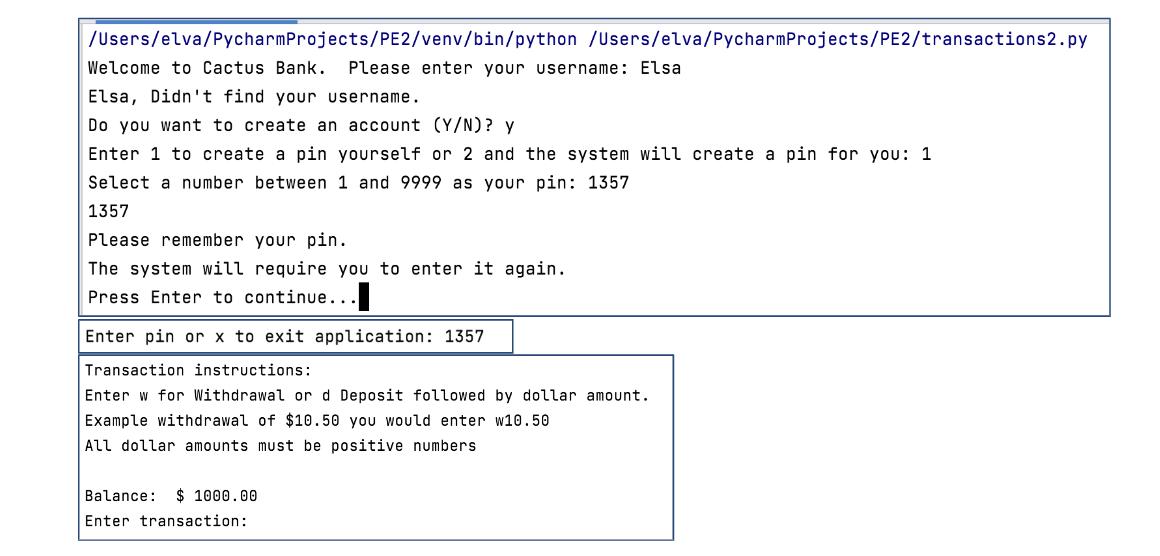
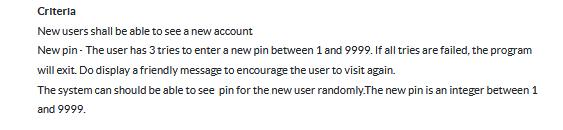
1. Write decisions and loops to satisfy requirements. 2. Implement data structures such as lists, tuples, and/or dictionaries 3. Work with user input 4. Utilize the random module to generate random numbers and choices Program Overview The Bank client would like to add the following features to the system: 1. New users shall be able to create a new account if they would like to 2. New users can create a new pin themselves or let the system create a new pin randomly for them. 3. For users who would like to create a new pin themselves, display a message to let them know the pin needs to be an integer between 1 and 9999. Validate the pin a user entered. 4. The user has 3 tries to create a new pin between 1 and 9999. If all tries failed, the program will exit. Display a friendly message to encourage the user to visit again. 5. After the user successfully created a pin, save the username and the new pin. 1. Import modules. A module is a single python file and importing modules allows the use of functions, classes, and global variables/objects that reside in other python files. Another term used in this context is a package which is a namespace or collection of python modules. Packages are folders that contain a _init__.py file. This application will make use of two modules os and random. Add code below: import os import random Random will give us access to several useful methods that generate random results. One thing to note is this module should not be used for any security features that require random data. Instead secret is used instead of random. Below is a list of useful functions in random: random.random() - returns a decimal value between 0 and 1 random.uniform( , ) - returns decimal value between min and max random.randint( , ) - returns in between min and max (max is included) random.choices( , weights = , k= )-returns random values within the list argument supplied. Weights and k are optional arguments, but weights can be used if a value in the list has a different weighted chance of occurrence. K is the number of choices you want returned. This method can return the same value in the list if k is greater than 1. random.shuffle( ) - moves values into new index positions within the list random.sample( , k= ) - similar to choices except shuffle will only return 1 occurrence of a value in the list (no repeating values). - For more info https://docs.python.org/3/library/random.html 2. Declare a dictionary users dictionary - this dictionary will include users' username and pin information. The dictionary should have at least one item with your asurite and your pin, where asurite' is the key and 'pin' is the value. The pin is an integer. # Create objects for balances and transaction users 'selin2': 9999} account = 1000.00 # Allow 3 invalid pin entries tries 1 pin tries = 1 max tries = 3 pin_created = False. Dictionary is a built-in Python Data Structure that is mutable. https://www.geeksforgeeks.org/python-dictionary/ 4. If user is not in users: 4.1. Print out a message to let the customer know that he/she doesn't have an account with the Bank. 4.2. Print out another message to ask the customer if he/she would like to have an account with the bank. Convert the input to lower case with casefold() or lower() function. 4.2.1 4.2.2 If the first letter entered is not equal to 'y', print out an inspiring message to encourage the customer to come back again, then exiting the program. else ask the customer if he/she would like to enter 1 to create a pin or enter 2 to have the system create a new pin 4.2.2.1. The user gets 3 chances to pick a pin between 1 to 9999. 4.2.2.2. If the customer would like to have the system create a new pin, the system will randomly create a pin between 1 to 9999 4.2.3 4.2.4 4.2.5 Save the new username and pin to users dictionary when the pin is successfully created. users[user] = pin After the pin is created, display the following message: Please remember your pin. The system will require you to enter it again. Press Enter to continue.. Clear the output screen once the pin is created with os.system function. 5. Continue with the code we created in PE to ask the user (the existing user or the new user) to enter the pin and make transactions. Please note: we will not code an else block for the if statement coded in step 4. 5.1. In the users dictionary, now the pin is saved as an integer not a string. After we ask a bank customer to selection = input('Enter pin or x to exit application: ').casefold() 5.2. We first check to see if selection == 'x'. If that is true, use the keyword break to exit the while loop if x is entered, as we coded in PE1. 5.3. If the bank customer entered the pin, we need to convert that to an integer since all inputs collected from console are string. Here is how we coded in PE1: elif selection!= pin: We will change that to the following in PE2: elif int(selection) != users [user] : Sample Output (running app in command prompt) /Users/elva/Pycharm Projects/PE2/venv/bin/python /Users/elva/Pycharm Projects/PE2/transactions2.py Welcome to Cactus Bank. Please enter your username: selin2 Enter pin or x to exit application: 9999 Transaction instructions: Enter w for Withdrawal or d Deposit followed by dollar amount. Example withdrawal of $10.50 you would enter w10.50 All dollar amounts must be positive numbers. Balance: $ 1000.00 Enter transaction: w100 Starting balance is 1000.00 After Withdrawal new balance is 900.00 Balance: $ 900.00 Enter transaction: /Users/elva/Pycharm Projects/PE2/venv/bin/python /Users/elva/Pycharm Projects/PE2/transactions2.py Welcome to Cactus Bank. Please enter your username: Emma Emma, Didn't find your username. Do you want to create an account (Y/N)? y Enter 1 to create a pin yourself or 2 and the system will create a pin for you: 2 Your pin is: 9316 Please remember your pin. The system will require you to enter it again.. Press Enter to continue... /Users/elva/PycharmProjects/PE2/venv/bin/python /Users/elva/PycharmProjects/PE2/transactions2.py Welcome to Cactus Bank. Please enter your username: Elsa Elsa, Didn't find your username. Do you want to create an account (Y/N)? y Enter 1 to create a pin yourself or 2 and the system will create a pin for you: 1 Select a number between 1 and 9999 as your pin: -20 -20 Invalid pin entered. Select a number between 1 and 9999 as your pin: 1999999999 1999999999 Invalid pin entered. Select a number between 1 and 9999 as your pin: -200 -200 Invalid pin entered. Please try later ..... Process finished with exit code 0 /Users/elva/PycharmProjects/PE2/venv/bin/python /Users/elva/PycharmProjects/PE2/transactions2.py Welcome to Cactus Bank. Please enter your username: Elsa Elsa, Didn't find your username. Do you want to create an account (Y/N)? y Enter 1 to create a pin yourself or 2 and the system will create a pin for you: 1 Select a number between 1 and 9999 as your pin: 1357 1357 Please remember your pin. The system will require you to enter it again. Press Enter to continue... Enter pin or x to exit application: 1357 Transaction instructions: Enter w for Withdrawal or d Deposit followed by dollar amount. Example withdrawal of $10.50 you would enter w10.50 All dollar amounts must be positive numbers Balance: $1000.00 Enter transaction: Criteria New users shall be able to see a new account New pin - The user has 3 tries to enter a new pin between 1 and 9999. If all tries are failed, the program will exit. Do display a friendly message to encourage the user to visit again. The system can should be able to see pin for the new user randomly.The new pin is an integer between 1 and 9999.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
importrandom importos classCactusBank def initself selfuseraccounts def createaccountself username C...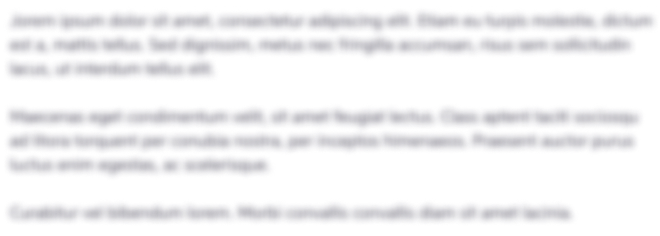
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started