Question
1.11 Lab: Airports 3 The input file airports.txt contains up to 100 lines. On each line there is an airport code (a unique identifier) followed
1.11 Lab: Airports 3
The input file airports.txt contains up to 100 lines. On each line there is an airport code (a unique identifier) followed by the airports number of enplanements (commercial passenger boardings) and city served. You may assume that the data in the input file are sorted by the airports code as shown below:
BFL 100433 Bakersfield BUR 2077892 Burbank LAX 39636042 Los Angeles MRY 192136 Monterey
Reuse code from the previous two labs (Airports 1 & 2) to search airports based on their code calling Binary Search in a loop: prompt the user to enter an airport code, such as LAX then call the Binary Search function to search for LAX. If found, display its number of enplanements and city served, otherwise display an error message. Keep track of the number of searches for each airport in another array. When done searching, write the arrays to an output file named searchReport.txt, as shown below.
2 BFL Bakersfield (100433) 0 BUR Burbank (2077892) 5 LAX Los Angeles (39636042) 0 MRY Monterey (192136)
The first number on the first line, 2, shows that there were 2 searches for BFL, the first number on the second line, 0, shows that there were 0 searches for BUR, and so on.
Requirements
Start with reading and understanding the starter code. This is an incomplete program. Finish the program following the existing design with 6 functions in addition to main():
- printInfo() nothing to do
- showTimeStamp() nothing to do
- binarySearch() reuse
- writeToFile() finish writing this function (prototype and call are given)
- searchTestDriver() finish writing this function (prototype and call are given)
- readArpData() finish writing the this function:
- write the functions header, declaration, and call
- take your time to understand its implementation; you will have to write similar functions for other assignments
Notice the style for defining parameters one per line:
void writeToFile(string filename, const string code[], const int enp[], const string City[], const int searchCount[], int size);
When a function has a long list of parameters, this style enhances the readability of the code, therefore is highly recommended.
Comments
- Write a comment in the beginning of each source file, including your name and IDE
- Write a multi-line comment for each function definition
- Write short one-line comments where appropriate.
For the first assignment, all these comments were given as an example to follow in future assignments.
Indentation and Spacing
Proper indentation and spacing enhances the readability of your code.
- Indent everything in main() and other functions.
- Always indent within curly braces.
- Use one space after comma and no space in front of it
- Leave one blank line between the two sections of any function.
- Use blanks around binary operators: score1 + score2
- No blanks for unary operators: ++count;
Sample Output
Project: Airports Please enter an airport code, such as LAX: SFO SFO found! See related data below: Code: SFO City: San Francisco Enplanements: 25707101 Would you like to look up another airport(Y/N)? N The updated data has been saved into the file "searchReport.txt".
Code to improve
#include
using namespace std;
// function prototypes void printInfo(); void readArpData(); int binarySearch(const string [], int, string); void searchTestDriver(const string [], const int [], const string [], int [], int); void writeToFile(string, const string [], const int [], const string [], const int [], int size);
int main() { // constant definition const int AIRPORTS = 500; // maximum size of arrays // filenames string infilename = "airports.txt"; string outfilename = "searchReport.txt"; // arrays definitions string city[AIRPORTS]; string code[AIRPORTS]; int enp[AIRPORTS]; int searchCount[AIRPORTS]; // other variables int size; // actual size of arrays
// function calls printInfo(); readArpData(); searchTestDriver(code, enp, city, searchCount, size); writeToFile(outfilename, code, enp, city, searchCount, size);
return 0; } /*~*~*~*~*~*~ This function displays the project's title *~*/ void printInfo() { cout << "Project: Airports" << endl; }
/*~*~*~*~*~*~ This function reads data about airports from a file into 3 parallel arrays. The size variable will hold the number of airports that were stored in these arrays. *~*/ void readArpData() { ifstream inputFile; // open file inputFile.open(filename);
// validation if(inputFile.fail()){ cout << "Error opening the inpur file: " << filename << ". "; exit(EXIT_FAILURE); } // read data from file into three parallel arrays size = 0; while (size < limit && inputFile >> code[size] >> enp[size]){ inputFile.ignore(); getline(inputFile, city[size]); size++; }
// check if size reaches maximum size of array and there is more data in the file if(size == limit && !inputFile.eof()){ cout << " The file contains more than "<< limit << "lines! "; exit(EXIT_FAILURE); }
// close file inputFile.close(); }
/*~*~*~*~*~*~ This function performs the binary search on a string array. The array has size elements. A value stored in this array will be searched. It will return the array subscript if found. Otherwise, -1 will be returned. *~*/ int binarySearch(const string code[], int size, string target) {
}
/*~*~*~*~*~*~ This writeToFile function accepts four arrays and their size, and a string value as arguments. It will show the number of searches for each airport, and airports information into an output file. *~*/ void writeToFile(string filename, const string code[], const int enp[], const string city[], const int searchCount[], int size) {
} /*~*~*~*~*~*~ This function: - prompts the user to enter an airport code - calls binary search to search for that code - displays related data if found, or an error message - keeps track of the number of searches - asks the user if they want to continue searching *~*/ void searchTestDriver(const string code[], const int enp[], const string city[], int searchCount[], int size) {
}
/*~*~*~*~*~*~ Save the output below
*~*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
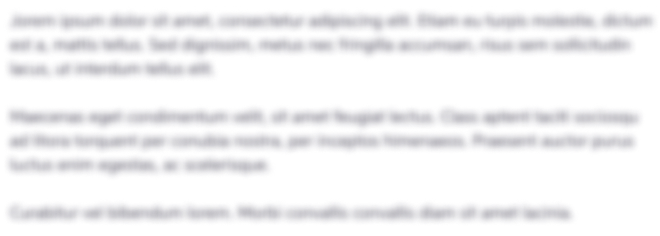
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started